Welcome to the ultimate guide on mastering email validation in .NET. As a seasoned .NET developer, ensuring the accuracy and validity of user-provided email addresses is crucial for data integrity and security. In this comprehensive article, we will explore various techniques, best practices, and code examples to help you implement effective email address verification in your .NET applications. Whether you're a beginner or an experienced developer, this guide will equip you with the knowledge and skills to ensure accurate and secure email validation.
Understanding Email Validation in .NET
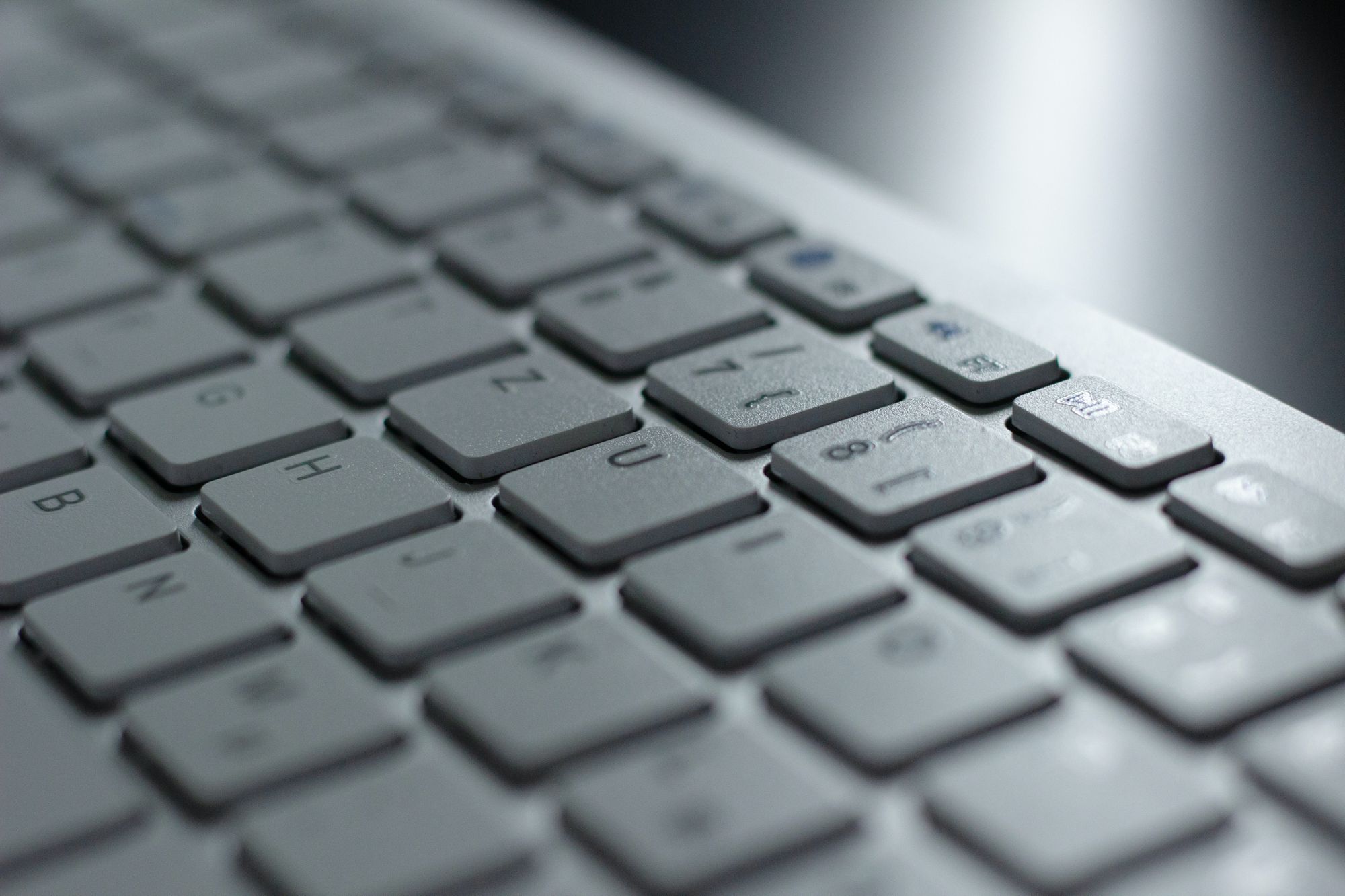
Email validation is the process of verifying the format and authenticity of an email address provided by a user. In .NET, there are several approaches and libraries available to perform email validation effectively. By implementing proper email validation techniques, you can prevent incorrect or malicious email addresses from being submitted, ensuring the integrity of your data.
Using Regular Expressions for Email Validation
Regular expressions are a powerful tool for pattern matching and can be used to validate email addresses in .NET. They provide flexibility in defining custom validation rules based on email address patterns. Let's explore an example of email validation using regular expressions in C#:csharpCopy codeusing System; using System.Text.RegularExpressions; public class EmailValidator{ public static bool IsValidEmail(string email) { string pattern = @"^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$"; return Regex.IsMatch(email, pattern); } } // Usagestring emailAddress = "example[email protected]"; if (EmailValidator.IsValidEmail(emailAddress)) { // Valid email address Console.WriteLine("Email is valid."); } else{ // Invalid email address Console.WriteLine("Email is invalid."); }
Leveraging .NET Framework for Email Validation
The .NET framework provides built-in classes and methods to facilitate email validation. The System.Net.Mail
namespace offers the MailAddress
class, which simplifies the process of email validation. Let's take a look at an example:csharpCopy codeusing System; using System.Net.Mail; public class EmailValidator{ public static bool IsValidEmail(string email) { try { var mailAddress = new MailAddress(email); return true; } catch (FormatException) { return false; } } } // Usagestring emailAddress = "example[email protected]"; if (EmailValidator.IsValidEmail(emailAddress)) { // Valid email address Console.WriteLine("Email is valid."); } else{ // Invalid email address Console.WriteLine("Email is invalid."); }
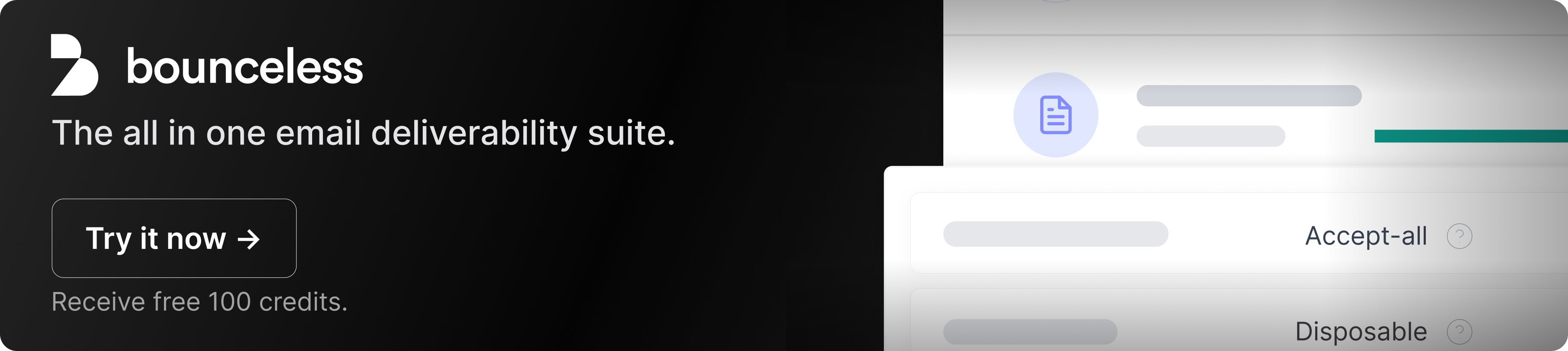
Implementing Email Validation in .NET Applications
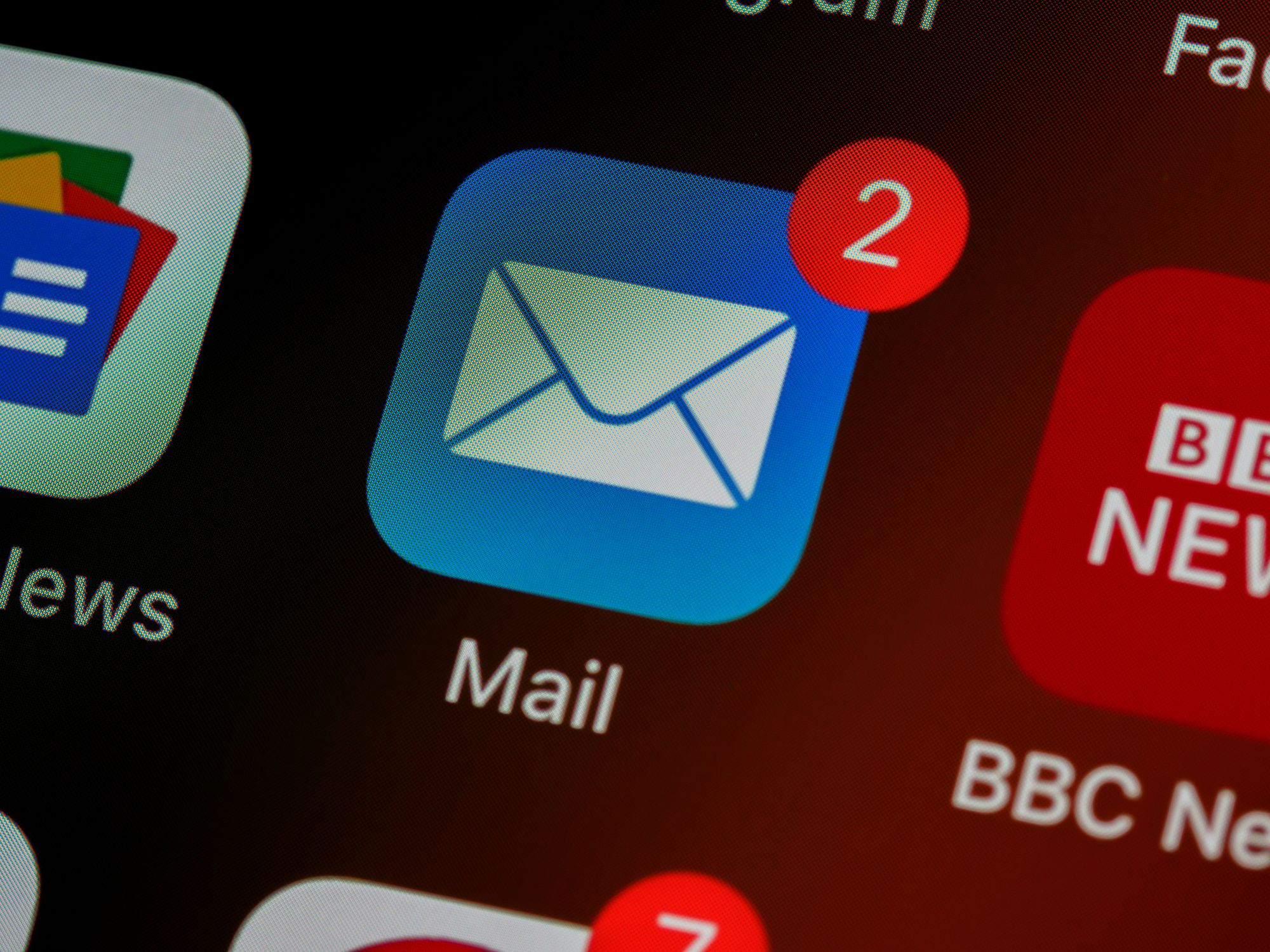
Now that we understand the basic techniques for email validation in .NET, let's explore how we can implement email validation in different scenarios.
Web Forms
In web applications, validating email addresses entered through web forms is crucial. You can leverage the power of .NET and HTML form validation to ensure accurate email validation. Here's an example using ASP.NET Web Forms:htmlCopy code<asp:TextBox ID="txtEmail" runat="server"></asp:TextBox><asp:RegularExpressionValidator ID="revEmail" runat="server" ControlToValidate="txtEmail" ValidationExpression="^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$" ErrorMessage="Please enter a valid email address." />
MVC Applications
In ASP.NET MVC applications, you can perform email validation using model annotations or custom validation attributes. Here's an example using model annotations:csharpCopy codepublic class User{ [Required(ErrorMessage = "Email is required.")] [EmailAddress(ErrorMessage = "Please enter a valid email address.")] public string Email { get; set; } }
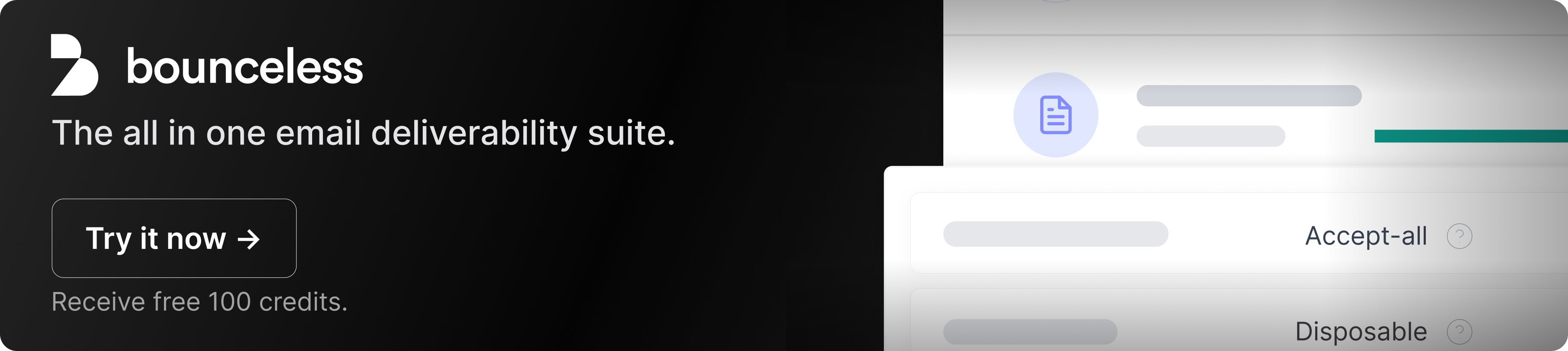
Best Practices for Email Validation
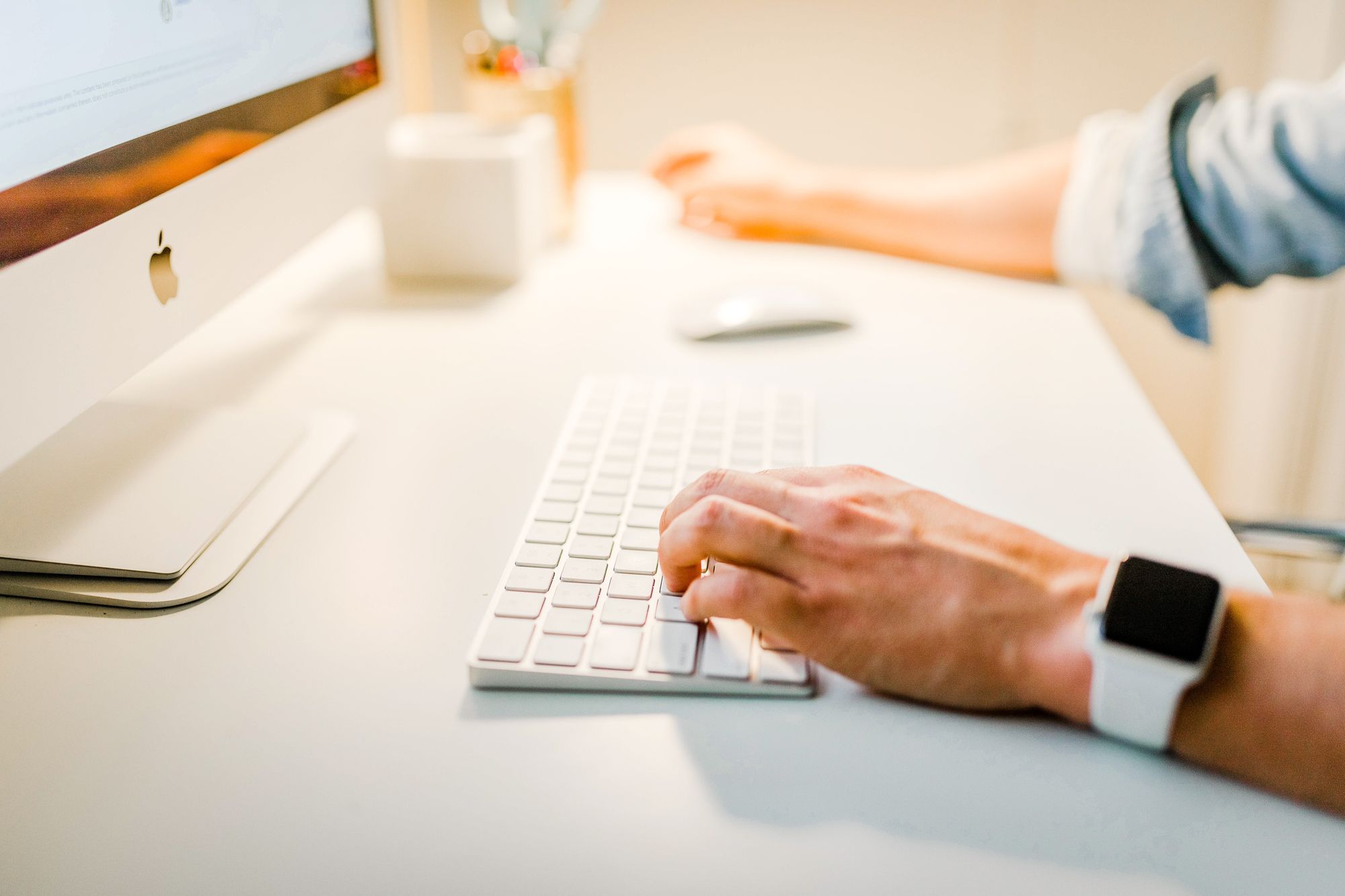
To ensure effective email validation in your .NET applications, consider the following best practices:
Combine Client-side and Server-side Validation
While client-side validation improves user experience by providing real-time feedback, it can be bypassed. Therefore, it's essential to implement server-side validation to ensure data integrity and security.
Use .NET Libraries and Frameworks
Utilize libraries and frameworks specifically designed for email validation in .NET, such as FluentValidation and DataAnnotations. These tools provide convenient methods and utilities to simplify email validation code and promote maintainability.
Sanitize User Input
Before validating an email address, ensure that user input is properly sanitized to prevent common security vulnerabilities such as cross-site scripting (XSS) attacks.
Frequently Asked Questions
Q1: Can .NET validate all possible email addresses?
A1: While .NET can effectively validate most common email address formats, it cannot validate all possible email addresses due to the complexity and constant evolution of email standards.
Q2: Is client-side validation enough for email validation?
A2: No, client-side validation alone is not sufficient. It's crucial to implement server-side validation as well to ensure data integrity and security.
Q3: Are there any third-party libraries available for email validation in .NET?
A3: Yes, there are several third-party libraries such as FluentValidation, MimeKit, and MailKit that provide advanced email validation features and additional functionalities.
Q4: How can I handle email validation errors gracefully?
A4: When email validation fails, provide clear and user-friendly error messages to guide users in correcting their input. Consider using validation summary or inline error messages for better user experience.
Q5: What are some common pitfalls to avoid in email validation?
A5: Some common pitfalls to avoid include relying solely on client-side validation, not considering international email address formats, and not keeping up with email standards updates.
Conclusion
Email validation is a crucial aspect of building robust and secure .NET applications. By leveraging the power of regular expressions and the .NET framework, you can ensure the accuracy and security of email addresses provided by users. With the knowledge gained from this comprehensive guide, you are now equipped to master email validation in .NET and create applications that validate email addresses effectively. Implement best practices, utilize appropriate libraries, and combine client-side and server-side validation to achieve accurate and secure email validation in your .NET projects.