Welcome to the ultimate guide on mastering email check in PHP. As a web developer, ensuring the validity and accuracy of user-submitted data, particularly email addresses, is of utmost importance. In this comprehensive guide, we will delve into the world of PHP and explore various techniques, best practices, and code examples to help you validate email addresses with confidence. Whether you're a beginner or an experienced developer, this article will equip you with the knowledge and skills to build robust and secure web applications.
Understanding Email Validation in PHP
Email validation involves verifying the format and authenticity of an email address provided by a user. PHP, being a powerful server-side scripting language, offers a range of tools and functions specifically designed for email validation. These tools enable you to validate email addresses efficiently, preventing incorrect or malicious data from being submitted and ensuring data integrity.
Using Filter Functions for Email Validation
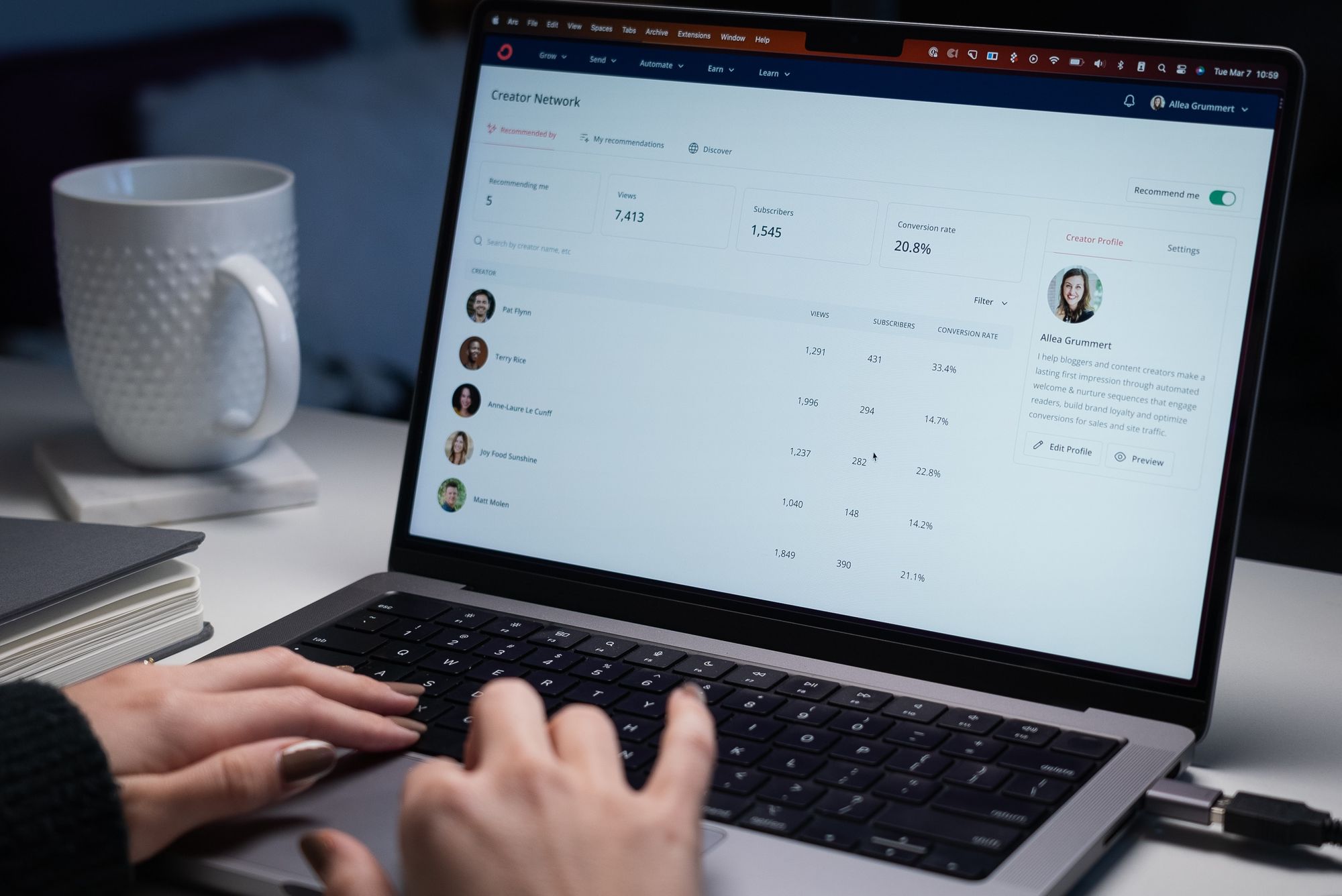
PHP provides built-in filter functions that simplify the process of email validation. These functions leverage filters and predefined validation rules to ensure the validity of email addresses. Let's explore two commonly used filter functions for email validation in PHP:
filter_var() Function
The filter_var()
function is a versatile tool that allows you to apply various filters to a given value. For email validation, you can use the FILTER_VALIDATE_EMAIL
filter, which specifically validates email addresses. Here's an example:phpCopy code$email = "example[email protected]"; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { // Valid email address echo "Email is valid."; } else { // Invalid email address echo "Email is invalid."; }
filter_input() Function
The filter_input()
function enables you to directly validate user input received from a specific input variable. By specifying the input variable and the desired filter, you can validate email addresses effectively. Here's an example:phpCopy code$email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL); if ($email) { // Valid email address echo "Email is valid."; } else { // Invalid email address echo "Email is invalid."; }
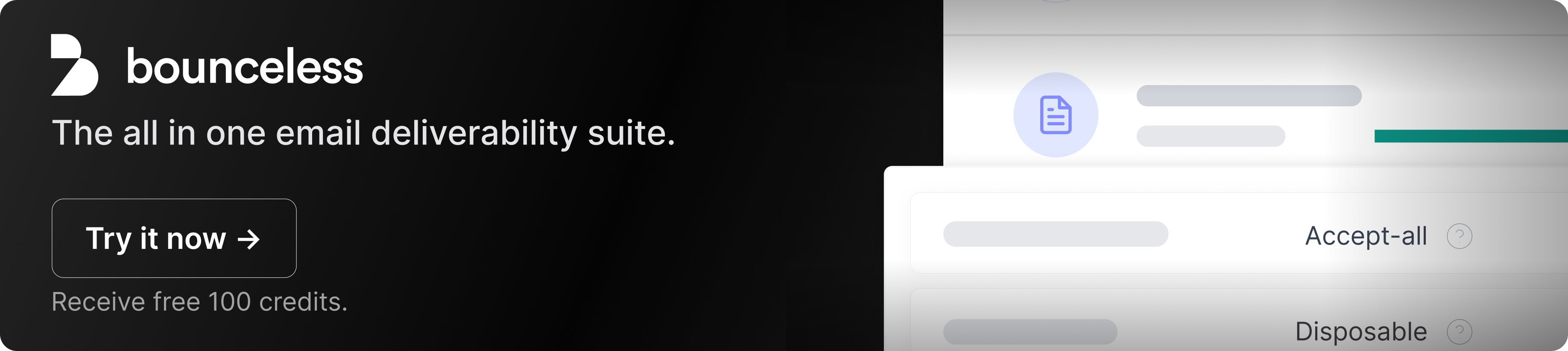
Implementing Email Validation in PHP
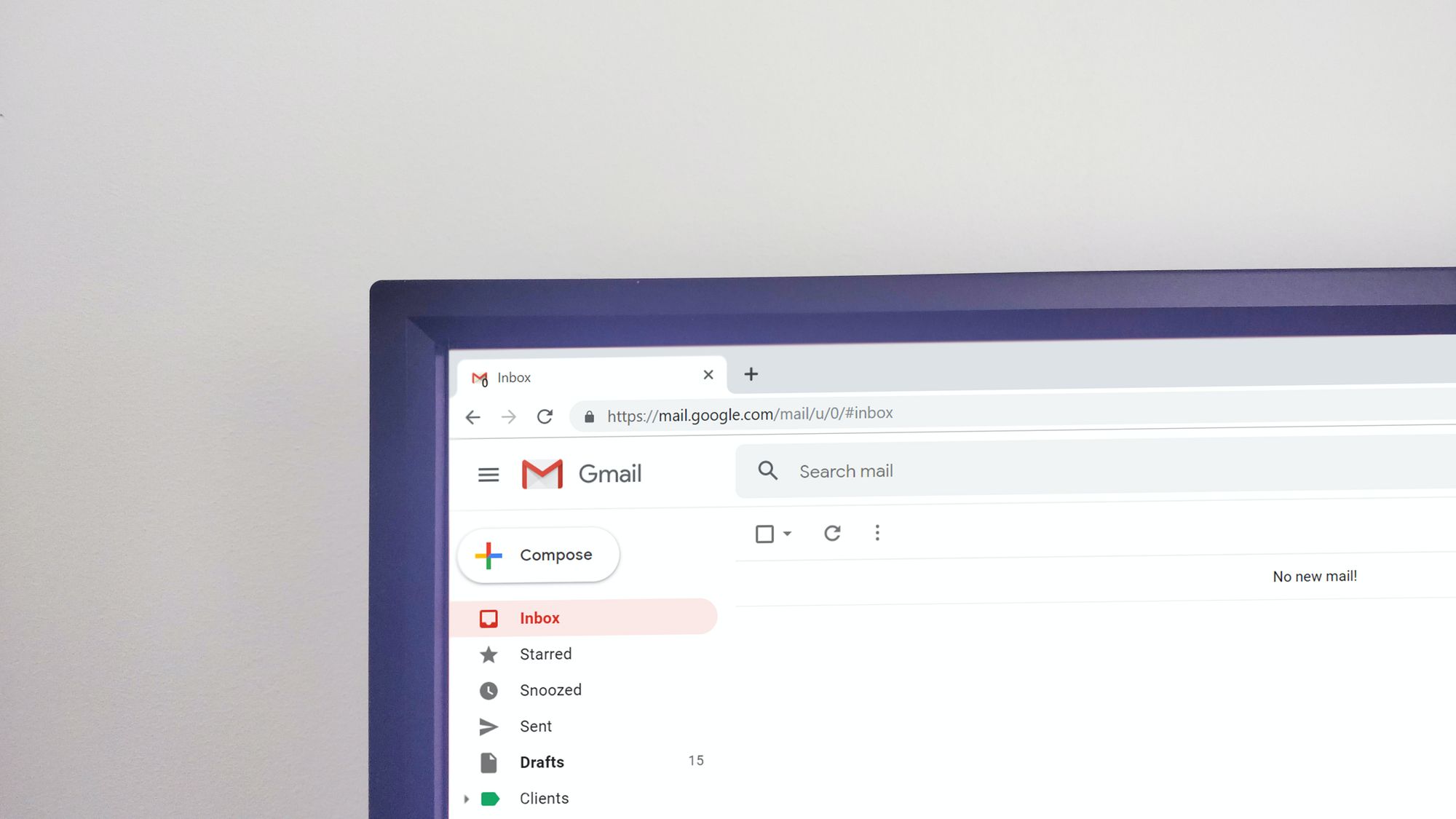
Now that we have explored the filter functions for email validation in PHP, let's dive into implementing email check in different scenarios.
Form Validation
Form validation is a common use case where email validation plays a crucial role. By utilizing the filter functions in combination with form submissions, you can validate email addresses efficiently. Here's an example:phpCopy code$email = $_POST['email']; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { // Valid email address // Process form submission} else { // Invalid email address // Display error message to the user}
Real-time Validation
Real-time validation provides users with immediate feedback as they type, guiding them to correct any mistakes. By combining JavaScript and PHP, you can implement real-time email validation. Here's an example:javascriptCopy code// JavaScript code for real-time validationconst emailInput = document.getElementById('email'); emailInput.addEventListener('input', function() { const email = this.value; fetch('validate-email.php?email=' + encodeURIComponent(email)) .then(response => response.text()) .then(result => { if (result === 'valid') { // Valid email address this.classList.remove('invalid'); this.classList.add('valid'); } else { // Invalid email address this.classList.remove('valid'); this.classList.add('invalid'); } }); });
phpCopy code// PHP code for real-time validation (validate-email.php)$email = $_GET['email']; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo "valid"; } else { echo "invalid"; }
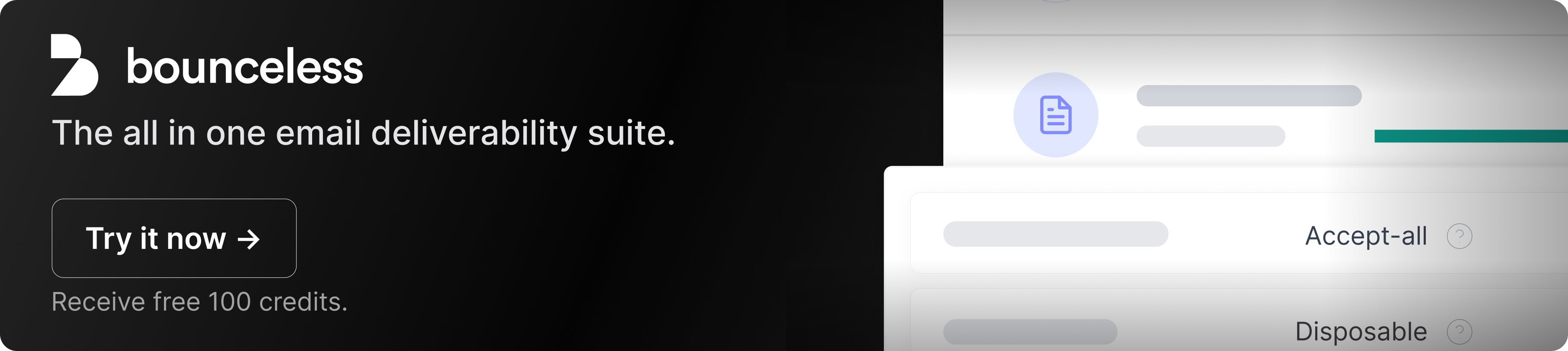
Best Practices for Email Validation
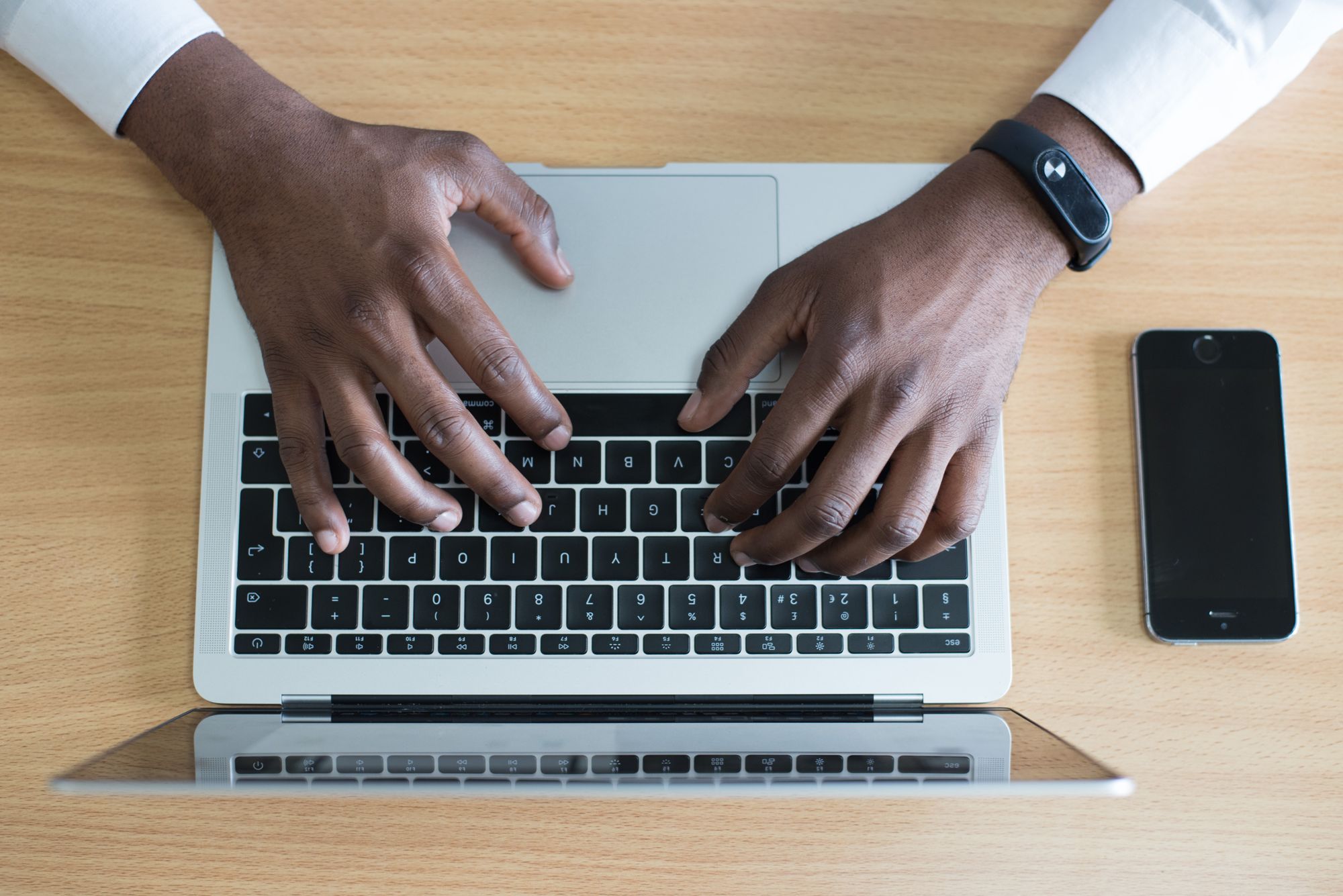
To ensure effective email validation in your PHP code, consider the following best practices:
Combine Front-end and Back-end Validation
While PHP validation provides server-side security, it's crucial to implement front-end validation as well. This approach provides a better user experience by offering real-time feedback and reduces unnecessary server requests.
Use Prepared Statements for Database Operations
When storing or retrieving email addresses from a database, utilize prepared statements or parameterized queries to prevent SQL injection attacks.
Display Clear Error Messages
When email validation fails, provide clear and meaningful error messages to users. Inform them about the specific validation requirements and how to correct the error.
Frequently Asked Questions
Q1: Can PHP validate all possible email addresses?
A1: While PHP can effectively validate most common email address formats, it cannot validate all possible email addresses due to the complexity and constant evolution of email standards.
Q2: Is server-side validation necessary if JavaScript validation is already in place?
A2: Yes, server-side validation is essential because client-side validation can be bypassed by users or automated scripts. Server-side validation adds an extra layer of security and ensures data integrity.
Q3: Are there any PHP libraries or frameworks available for email validation?
A3: Yes, there are several PHP libraries and frameworks, such as Symfony Validator, Laravel Validation, and Respect\Validation, that provide convenient functions and utilities for email validation and form validation in general.
Q4: What are some common mistakes to avoid in email validation?
A4: Some common mistakes to avoid in email validation include relying solely on client-side validation without server-side validation, not using secure database operations when storing email addresses, and not providing clear error messages to users.
Q5: Can I use regular expressions for email validation in PHP?
A5: While regular expressions can be used for email validation in PHP, utilizing PHP's built-in filter functions provides a more reliable and efficient approach.
Conclusion:
Email validation is a critical aspect of web development, and PHP offers powerful tools to ensure the accuracy and reliability of email addresses. By leveraging filter functions and following best practices, you can effectively validate email addresses in PHP and create robust and secure web applications. With the knowledge gained from this comprehensive guide, you are now well-equipped to master email check in PHP and enhance the integrity of user-submitted data.Was this response better or worse?BetterWorseSame