In today's digital landscape, security and user trust are paramount for the success of any web application. Laravel, the popular PHP framework, offers a robust and convenient way to implement email verification during the registration process. By incorporating email verification, you can ensure that only valid users gain access to your application, enhancing security and building user confidence. In this article, we will explore the benefits of using Laravel's email verification feature, provide a comprehensive step-by-step implementation guide, and answer commonly asked questions.
Why Use Email Verification?
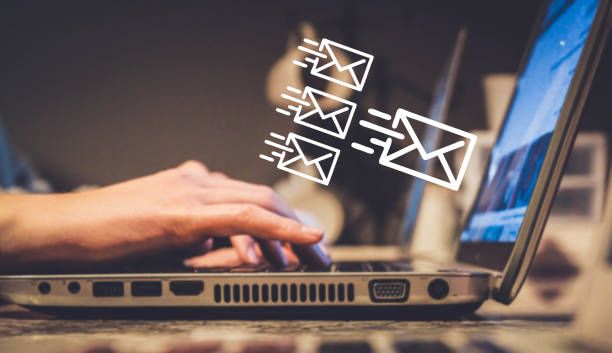
Email verification is a crucial security measure that helps prevent unauthorized access and malicious activities on your application. By requiring users to verify their email addresses during registration, you can:
- Confirm the user's identity: Email verification ensures that the person registering is the legitimate owner of the email address, reducing the risk of fake or fraudulent accounts.
- Reduce spam and bots: By validating email addresses, you can effectively block spam registrations and prevent bots from creating accounts on your application.
- Enhance user trust: When users see that your application employs email verification, they feel more confident about the security measures in place, leading to increased trust and engagement.
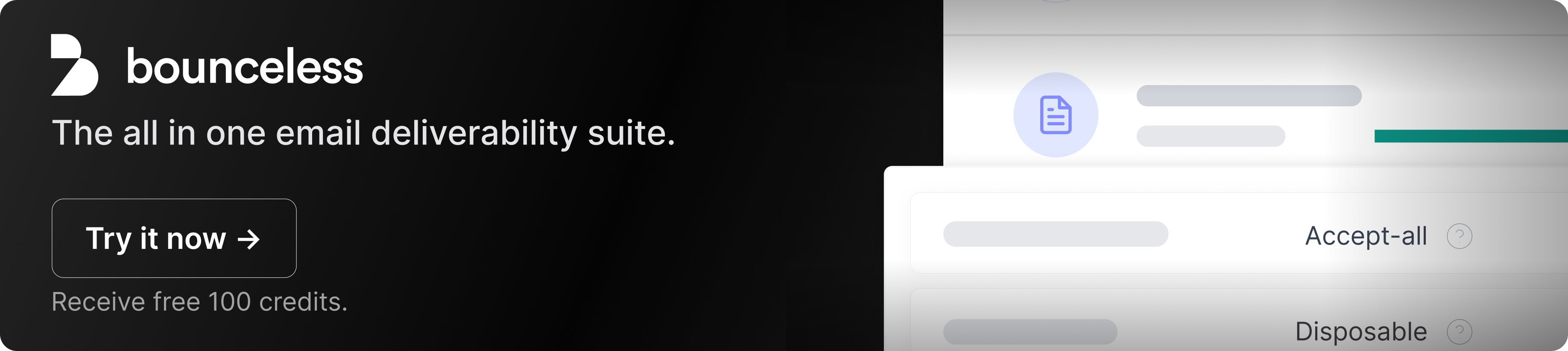
Implementing Email Verification in Laravel
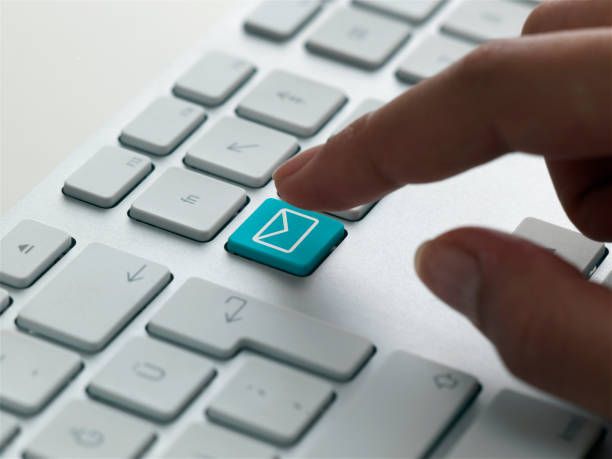
Now let's dive into the step-by-step process of implementing email verification in Laravel. We will cover the key aspects, including configuration, routing, email generation, and user verification.
- Configure your application: First, make sure your Laravel application is properly configured to handle email sending. Set up your mail driver, SMTP credentials, and other necessary configurations in the
.env
file. - Database migration: Laravel provides a migration command to create the necessary database table for email verification. Use the
php artisan migrate
command to generate the required migration file, and then run the migration to create the table. - Update User model: In your User model, implement the
MustVerifyEmail
contract provided by Laravel. This contract adds the necessary methods and attributes required for email verification. - Email generation: Laravel makes it easy to generate the email that will be sent to users for verification. Customize the email template by modifying the
verification.blade.php
file located in theresources/views/auth
directory. You can add your application's branding and personalized messages to the email template. - Route setup: Define the necessary routes for email verification in your
web.php
file. Laravel provides aAuth::routes(['verify' => true])
method that automatically sets up the required routes for registration and email verification. - Protect routes: To ensure that only verified users can access specific routes, use the
verified
middleware provided by Laravel. Apply this middleware to any routes that require user authentication. - Send verification email: After a user successfully registers, generate a verification email using Laravel's
sendEmailVerificationNotification()
method. This method sends an email with a unique verification link to the user's registered email address. - Verify user's email: When the user clicks the verification link, Laravel's built-in
EmailVerificationRequest
middleware handles the verification process. The user's email address is marked as verified in the database, and they are redirected to the designated success page.
Commonly Asked Questions
How does Laravel handle email verification tokens?
Laravel uses a secure and unique token-based system for email verification. Each user is assigned a unique verification token, which is stored in the database. This token is embedded in the verification link sent to the user's email address. When the user clicks the link, Laravel verifies the token and marks the email address as verified.
Can I customize the email sent for verification?
Yes, Laravel allows you to fully customize the email sent for verification. By modifying the verification.blade.php
file, you can add your application's branding, personalized messages, and design elements to create a seamless user experience.
How can I resend the verification email if a user doesn't receive it?
Laravel provides a convenient way to resend the verification email. By implementing the resend()
method in your UserController, you can trigger the generation and sending of a new verification email to the user.
Can I use a different email service for sending verification emails?
Yes, Laravel supports various mail drivers, including SMTP, Mailgun, SendGrid, and more. You can configure your preferred email service in the .env
file, allowing you to use the service of your choice for sending verification emails.
Conclusion
Implementing email verification during the registration process in Laravel offers numerous benefits, including enhanced security, reduced spam, and increased user trust. By following the step-by-step implementation guide provided in this article, you can easily incorporate email verification into your Laravel applications. Remember to customize the email template, configure your mail driver, and protect routes to ensure a seamless and secure user experience. With email verification in place, you can build a trustworthy platform that protects user data and provides peace of mind to your application's users.