Email validation is a crucial aspect of web development, ensuring that the email addresses provided by users are valid and formatted correctly. jQuery, a popular JavaScript library, offers powerful tools and functions that can simplify the process of email validation. In this comprehensive guide, we will explore various techniques and methods to validate email addresses using jQuery. Whether you are a beginner or an experienced developer, this article will provide you with the knowledge and resources to implement effective email validation in your web applications.
Why Validate Email Addresses?
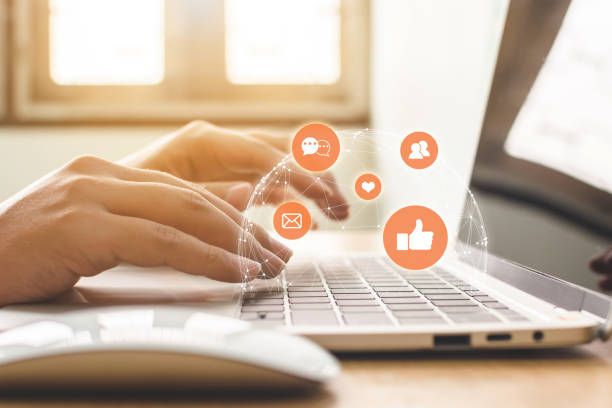
Before we delve into the details of email validation with jQuery, let's understand why it is important. Email validation serves multiple purposes, including:
- Ensuring Data Accuracy: Validating email addresses helps maintain accurate user data by filtering out incorrectly formatted or invalid email addresses. This is essential for maintaining the integrity of your database and ensuring reliable communication with users.
- Enhancing User Experience: By validating email addresses in real-time, you can provide instant feedback to users, alerting them if they have entered an incorrect or invalid email address. This improves the overall user experience and prevents frustration caused by submitting incorrect data.
- Preventing Spam and Abuse: Validating email addresses can help prevent spam registrations or abuse of your system by verifying that the email address belongs to a legitimate user. This helps in maintaining the security and integrity of your application.
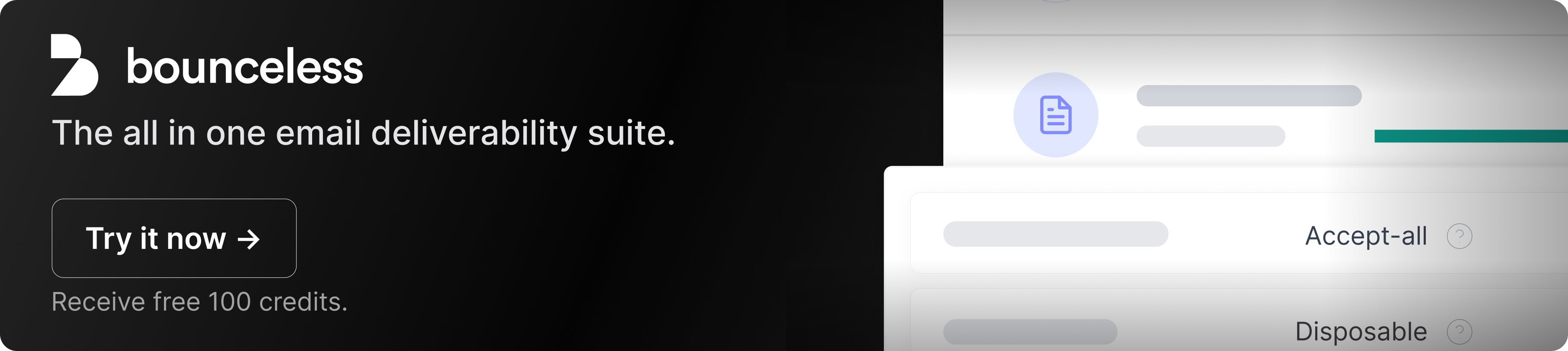
Now that we understand the importance of email validation, let's explore how jQuery can assist in achieving this task.
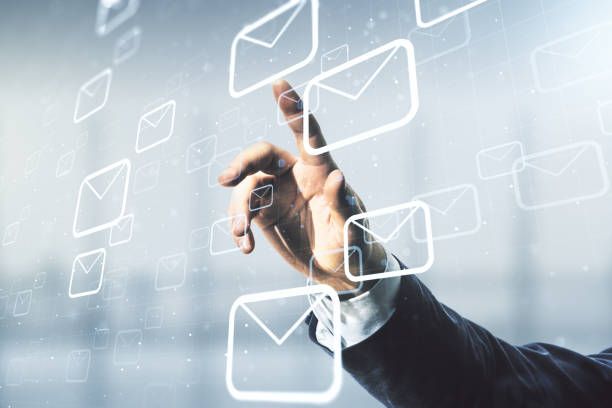
Email Validation with jQuery: jQuery provides several methods and plugins that simplify email validation. Let's explore some of the most commonly used techniques:
Regular Expressions: Regular expressions are powerful patterns that can match and validate email addresses based on a specific set of rules. jQuery allows you to leverage regular expressions to validate email addresses easily. Here's an example:javascriptCopy codefunction validateEmail(email) { var emailRegex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/; return emailRegex.test(email); }
In this example, we define a regular expression pattern that matches the standard email format. The test()
method checks if the given email matches the pattern, returning true
if it is valid and false
otherwise.
jQuery Validation Plugin: The jQuery Validation plugin is a popular choice for form validation, including email validation. It provides a simple and intuitive way to validate various form inputs, including email addresses. Here's an example of how to use the plugin for email validation:javascriptCopy code$("#myForm").validate({ rules: { email: { required: true, email: true } }, messages: { email: "Please enter a valid email address" } });
In this example, we apply the validate()
method to the form with the ID "myForm". The rules
object specifies that the "email" field is required and must be a valid email address. The messages
object defines the error message to display if the validation fails.
Remote Validation: Sometimes, you may need to validate an email address by making an AJAX request to the server and checking if it exists or is associated with a specific domain. jQuery provides the $.ajax()
method to perform such requests. Here's an example:javascriptCopy code$("#emailInput").blur(function() { var email = $(this).val(); $.ajax({ url: "validate-email.php", method: "POST", data: { email: email }, success: function(response) { if (response === "valid") { // Email is valid } else { // Email is invalid } } }); });
In this example, we bind the blur()
event to the email input field. When the user moves away from the field, an AJAX request is sent to the server, passing the email address. The server-side script (validate-email.php
) validates the email and returns a response indicating its validity.
Commonly Asked Questions
Q1: Can email validation be performed without using jQuery?
A1: Yes, email validation can be done using other programming languages and frameworks as well. However, jQuery simplifies the process by providing easy-to-use methods and plugins specifically designed for client-side validation.
Q2: Is client-side validation enough to ensure email address validity?
A2: Client-side validation is convenient for providing instant feedback to users. However, it is essential to perform server-side validation as well to prevent any malicious attempts to bypass client-side checks.
Q3: Are regular expressions the only way to validate email addresses?
A3: No, regular expressions are just one approach. There are other techniques, such as domain verification and SMTP checks, which involve additional server-side processing. The choice of method depends on the specific requirements of your application.
Q4: Are there any jQuery plugins or libraries dedicated to email validation?
A4: Yes, apart from the jQuery Validation plugin mentioned earlier, there are several other plugins available, such as "jQuery EmailValidator" and "jQuery Email Validation Plugin." These plugins offer additional features and customization options for email validation.
Conclusion:
Email validation is a crucial aspect of web development, ensuring the accuracy of user data and improving the overall user experience. With jQuery, you have powerful tools and plugins at your disposal to simplify the process of email validation. By implementing these techniques and following best practices, you can enhance the reliability and security of your web applications. Remember to combine client-side validation with server-side validation for optimal results. Now that you have a comprehensive understanding of email validation with jQuery, it's time to implement these techniques and create robust, user-friendly web forms.