In the world of programming, Python has emerged as a versatile and powerful language. It offers a wide range of libraries and functionalities that can be leveraged for various tasks, including email checking and validation. If you're looking to validate email addresses efficiently and accurately, Python provides the tools and code snippets necessary to achieve that goal. In this comprehensive guide, we will explore the best practices, effective strategies, and commonly asked questions surrounding email checking with Python code.
Understanding the Importance of Email Checking
Email checking plays a crucial role in ensuring the validity and accuracy of email addresses. It helps prevent the entry of incorrect or malformed email addresses and ensures that only valid addresses are accepted in your applications or systems. By implementing email checking mechanisms, you can enhance data quality, improve user experience, and maintain the integrity of your communication processes.
Effective Strategies for Email Checking with Python Code
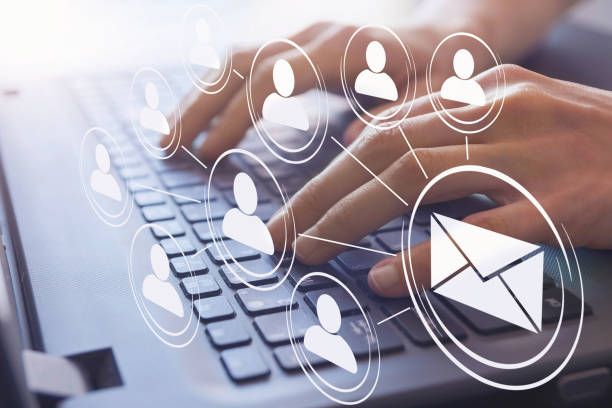
- Using Regular Expressions: Regular expressions (regex) provide a powerful method for pattern matching and validation. Python offers the
re
module, which allows you to write regex patterns to validate email addresses. By defining a pattern that adheres to the email address format, you can check if a given email address is valid. - Leveraging Email Validation Libraries: Python provides several libraries specifically designed for email validation. The
email-validator
library, for instance, offers a simple and efficient way to validate email addresses using predefined validation rules. It handles various aspects of email validation, including checking the format, domain existence, and MX record lookup. - SMTP Email Validation: Another approach to validate email addresses is by utilizing the Simple Mail Transfer Protocol (SMTP). Python's
smtplib
library enables you to connect to the recipient's mail server and simulate the sending of an email. If the server accepts the email, it indicates the validity of the email address. - Domain Verification: In addition to email format validation, you can also verify the existence of the domain associated with the email address. Python's
socket
library allows you to perform DNS (Domain Name System) lookups and validate the domain's existence.
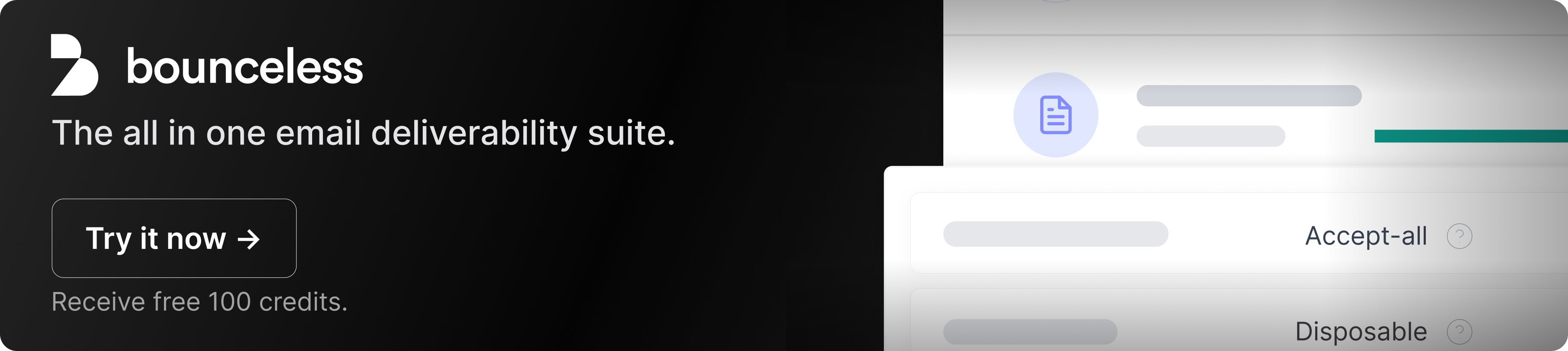
Python Code Snippets for Email Checking
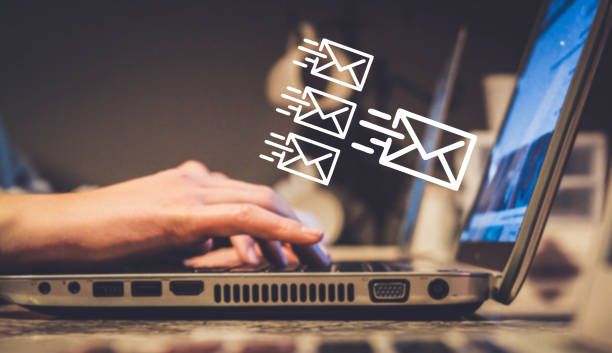
- Using Regular Expressions:pythonCopy code
import re def is_valid_email(email): pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$' if re.match(pattern, email): return True return False
- Using the email-validator Library:pythonCopy code
from email_validator import validate_email, EmailNotValidError def is_valid_email(email): try: v = validate_email(email) return True except EmailNotValidError: return False
- SMTP Email Validation:pythonCopy code
import smtplib from email.utils import parseaddr def is_valid_email(email): _, addr = parseaddr(email) try: with smtplib.SMTP() as smtp: code, _ = smtp.helo() if code != 250: return False smtp.mail('') code, _ = smtp.rcpt(addr) if code != 250: return False return True except smtplib.SMTPConnectError: return False
- Domain Verification:pythonCopy code
import socket def is_valid_email(email): _, domain = email.split('@') try: socket.gethostbyname(domain) return True except socket.gaierror: return False
Commonly Asked Questions
What is the difference between regex and email validation libraries
Regular expressions provide more flexibility but require manual pattern creation, while email validation libraries offer predefined rules and handle various aspects of validation, such as format and domain checks.
Which method is the most reliable for email checking?
The reliability of email checking methods depends on your specific requirements. SMTP email validation provides a higher level of accuracy by simulating the sending of an email, but it might be slower compared to regex or library-based validation.
Can Python code check if an email address exists?
Python can verify the domain existence associated with an email address by performing DNS lookups. However, it cannot confirm the existence of a specific mailbox or user within that domain.
How can I handle large-scale email checking tasks?
To handle large-scale email checking, consider implementing multithreading or multiprocessing techniques in your Python code. These approaches allow you to perform multiple email checks simultaneously, improving performance.
By leveraging the power of Python and the code snippets provided, you can effortlessly validate email addresses, ensure data integrity, and optimize your applications' performance. Master the art of email checking with Python code, enhance your validation processes, and unlock new possibilities for efficient email management.