Email validation is an essential part of any web application, especially those
developed using the MVC framework. In this article, we will explore the
reasons why email validation is important, how to implement it in MVC, and
answer some frequently asked questions about the topic.
Why is Email Validation Important?
Email validation is crucial for several reasons. Firstly, it ensures that the
email addresses entered by users are valid and formatted correctly. This
prevents errors in communication, such as bounced emails or undeliverable
messages.
Secondly, email validation helps to prevent spam and fraudulent activities. By
verifying that an email address belongs to a real person or organization, you
can reduce the number of fake registrations and malicious attacks targeting
your application.
Finally, email validation can improve the user experience by providing instant
feedback to users when they enter an incorrect or invalid email address. This
can reduce frustration and improve the overall usability of your application.
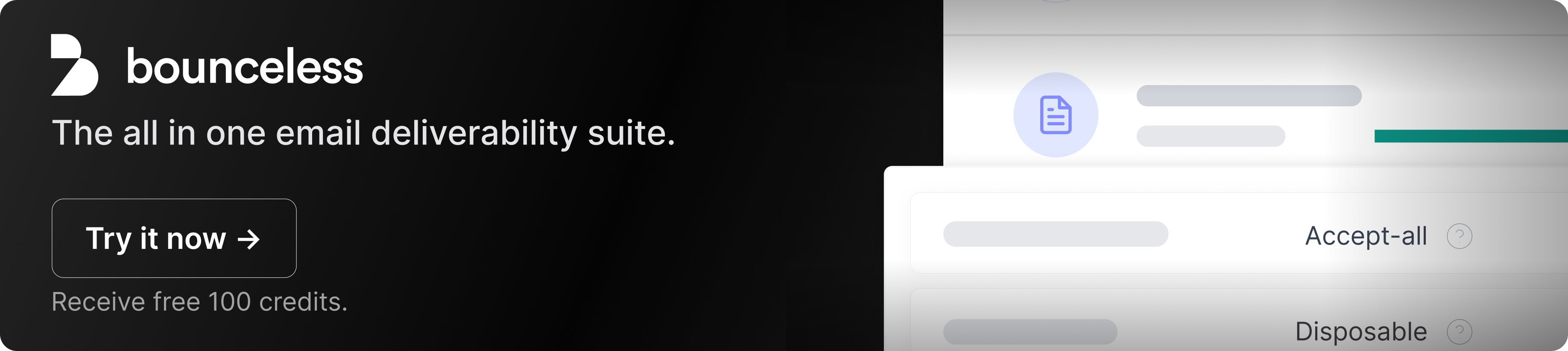
Implementing Email Validation in MVC
There are several ways to implement email validation in MVC, depending on your
specific requirements and preferences. One of the most common approaches is to
use data annotations, which are attributes that you can apply to model
properties to specify validation rules.
Here is an example of how to implement email validation using data annotations
in MVC:
public class User { [Required] [EmailAddress] public string Email { get; set; } }
In this example, we have defined a User model with an Email property that is
required and must be a valid email address. We have used the [Required] and
[EmailAddress] data annotations to specify these validation rules.
You can also use regular expressions to validate email addresses in MVC, as
shown in this example:
public class User { [Required] [RegularExpression(@"[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}$", ErrorMessage = "Invalid email address")] public string Email { get; set; } }
In this example, we have used a regular expression to validate email addresses
that follow a specific pattern. We have also specified a custom error message
to display when the validation fails.
Frequently Asked Questions
What is the best way to validate email addresses in MVC?
The best way to validate email addresses in MVC depends on your specific needs
and preferences. Using data annotations is a popular approach because it is
easy to implement and provides a lot of flexibility.
What are the common validation rules for email addresses?
The most common validation rules for email addresses include checking for the
presence of an "@" symbol, verifying that the domain name is valid, and
ensuring that the email address is formatted correctly.
How can I display validation errors to users in MVC?
You can display validation errors to users in MVC by using the
ValidationMessageFor or ValidationSummary helpers in your views. These helpers
will automatically generate error messages based on the validation rules
specified in your model.