jQuery is a popular JavaScript library that simplifies the process of web development by providing powerful tools and functionalities. One area where jQuery shines is in form validation, particularly email validation. Validating email inputs is crucial for ensuring the accuracy and reliability of user-submitted data. In this comprehensive guide, we'll explore the world of jQuery email validation, covering various methods, plugins, and techniques. By the end of this article, you'll be equipped with the knowledge and skills to implement robust email validation in your web applications.
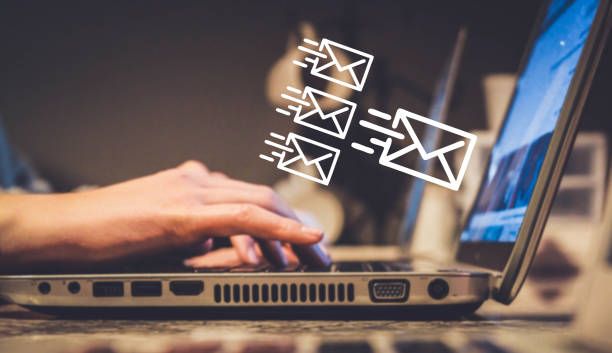
- The Importance of Email Validation: Email validation is a critical aspect of web development as it ensures that users provide valid email addresses. Validating email inputs helps in:
- Preventing errors: Validating email addresses reduces the number of errors caused by typos or incorrect formatting.
- Enhancing user experience: By providing real-time feedback on email input errors, you can improve the user experience and guide users towards correct inputs.
- Protecting data integrity: Valid email addresses ensure the accuracy and integrity of user data, minimizing issues such as bounced emails or undeliverable messages.
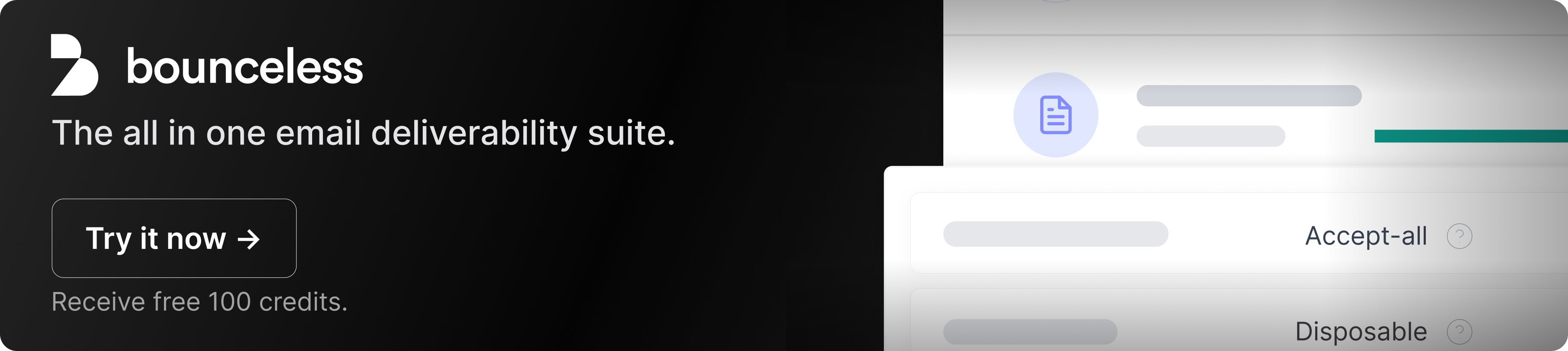
- Basic Email Validation with jQuery: jQuery provides built-in methods to perform basic email validation. The
.val()
function retrieves the value of an input field, which can then be tested using regular expressions or other validation techniques. Here's a simple example:javascriptCopy codelet email = $('#emailInput').val(); let emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; if (emailRegex.test(email)) { // Email is valid} else { // Email is invalid}
- jQuery Validation Plugins: To simplify the email validation process, several jQuery plugins are available. These plugins offer advanced features, such as real-time validation, customizable error messages, and additional validation options. Some popular plugins include:
- jQuery Validation Plugin: This plugin provides a comprehensive set of validation rules, including email validation. It offers customizable error messages, real-time validation, and support for complex validation scenarios.
- Parsley.js: Parsley.js is a powerful form validation library that includes built-in email validation functionality. It supports various validation constraints, customizable error messages, and internationalization.
- FormValidation: FormValidation is a versatile validation plugin that supports multiple frameworks, including jQuery. It offers an extensive range of validation options, including email validation with customizable error messages.
- Server-Side Email Validation: While client-side validation with jQuery is essential for providing real-time feedback to users, it's crucial to complement it with server-side validation. Client-side validation can be bypassed, and malicious users may attempt to submit invalid or harmful data. Server-side validation acts as a final layer of defense, ensuring that only valid email addresses are accepted and processed.
Tips for Effective Email Validation
a) Use Regular Expressions (Regex): Regular expressions are powerful patterns used to match and validate strings. Utilize regex patterns specifically designed for email validation to ensure accurate results.
b) Consider Domain Validation: In addition to syntax validation, consider implementing domain validation by checking if the email address domain exists and is active.
c) Provide Clear Error Messages: Clear and concise error messages help users understand why their email inputs are invalid. Customize error messages to guide users towards the correct format.
d) Leverage HTML5 Validation Attributes: HTML5 provides email input type and pattern attributes that can perform basic email validation without JavaScript. Combine these attributes with jQuery validation for enhanced functionality.
FAQs
Q1. Is jQuery the only way to perform email validation?
A1. No, jQuery is just one of many tools available for email validation. You can also achieve email validation using other JavaScript libraries, server-side languages like PHP or Python, or even HTML5 attributes.
Q2. Are jQuery plugins necessary for email validation?
A2. While not necessary, jQuery plugins offer additional features and customization options that can simplify and enhance the email validation process in your web applications.
Q3. Can email validation prevent all fake or invalid email addresses?
A3. Email validation can significantly reduce the number of fake or invalid email addresses but cannot guarantee 100% accuracy. Some users may intentionally provide fake or temporary email addresses.
Q4. Should I perform email validation on the client-side or server-side?
A4. It is recommended to perform email validation on both the client-side and server-side. Client-side validation provides real-time feedback to users, while server-side validation ensures the integrity and security of data.
Q5. Can I create custom email validation rules with jQuery?
A5. Yes, jQuery allows you to create custom validation rules using regular expressions or JavaScript functions. This flexibility allows you to tailor the validation to your specific requirements.
Conclusion
Email validation is a crucial aspect of web development to ensure accurate and reliable email inputs. By leveraging jQuery's powerful tools, plugins, and techniques, you can enhance user experience, prevent errors, and protect data integrity. Remember to combine client-side validation with server-side validation for optimal security. With the knowledge gained from this comprehensive guide, you can confidently implement robust email validation in your web applications and ensure the smooth handling of user-submitted email addresses.