Are you tired of dealing with inaccurate or non-existent email addresses in your Python applications? Ensuring the validity of email addresses is crucial for effective communication and data integrity. Luckily, Python provides a wealth of tools and libraries for email validity checking. In this comprehensive guide, we will dive into the world of email address verification in Python, explore popular methods and libraries, and answer the most commonly asked questions surrounding this topic.
In today's digital era, email communication serves as the backbone of various industries. However, the presence of invalid or incorrect email addresses can lead to undelivered messages, compromised data integrity, and a waste of valuable resources. Python, a powerful and versatile programming language, offers several methods and libraries to validate the authenticity and accuracy of email addresses. By implementing an email validity checker in your Python applications, you can ensure the reliability and efficiency of your email communications.
Understanding Email Address Validation in Python
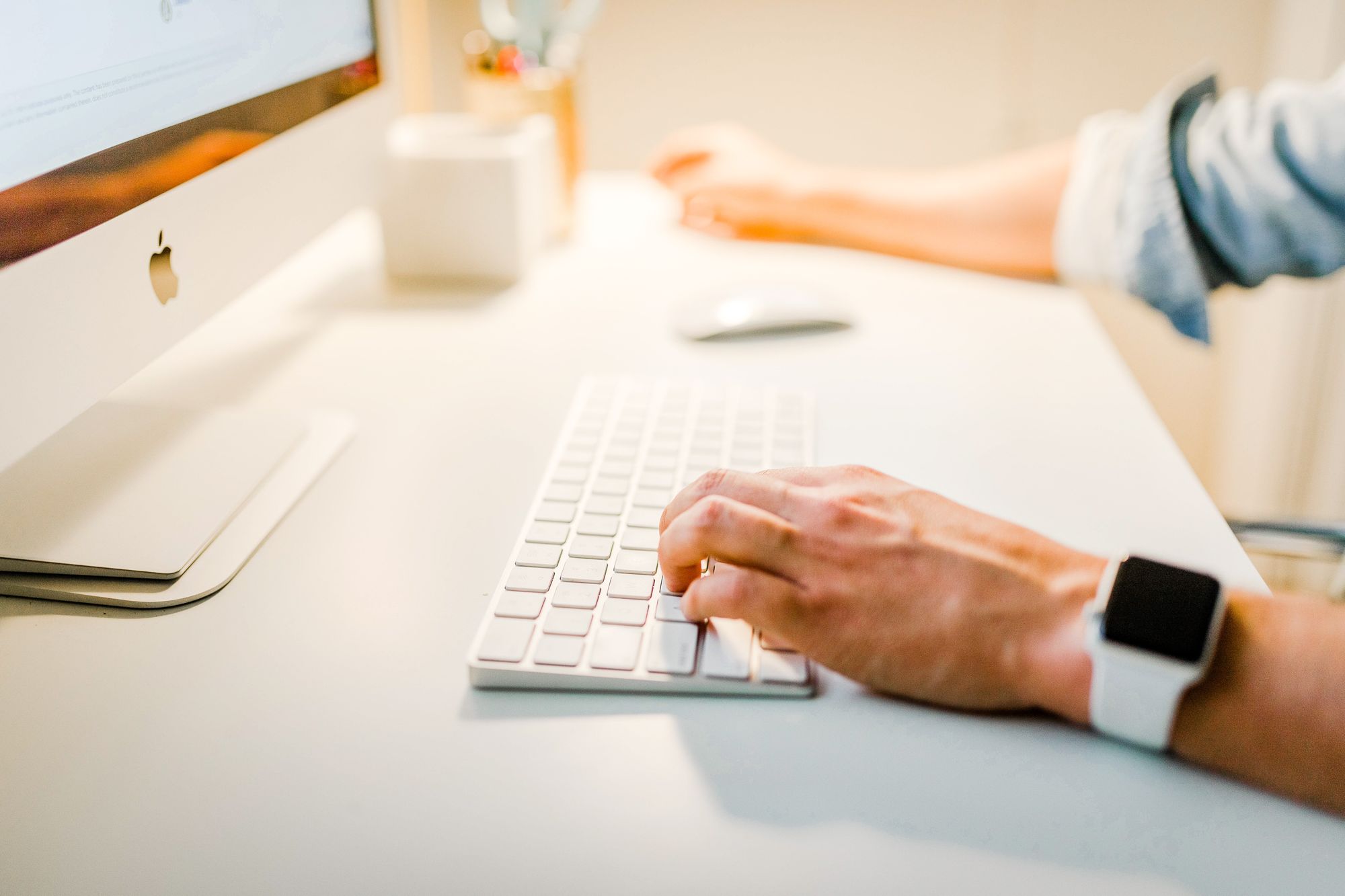
Email address validation involves verifying if an email address follows the correct syntax and if the domain exists and is active. Python provides various techniques and libraries that simplify the process of email validity checking. Let's explore some of the popular methods and libraries used for email address validation in Python:
- Regular Expressions (RegEx): Regular expressions are a powerful tool for pattern matching in Python. By constructing a RegEx pattern that matches the syntax of a valid email address, you can check if a given email address conforms to the expected format. While RegEx provides a basic level of email validation, it may not guarantee the existence or deliverability of the email domain.
- Built-in
email
Module: Python's built-inemail
module provides functionality to parse, manipulate, and validate email addresses. It offers classes likeemail.parser
andemail.utils
that can assist in validating the structure and format of an email address. However, the built-inemail
module may not perform domain-specific validation or provide domain existence checks. - Third-Party Libraries: Python boasts a vast ecosystem of third-party libraries that specialize in email validation. One such popular library is
email-validator
. It provides a comprehensive set of tools to validate the syntax, domain existence, and deliverability of email addresses. These libraries often offer additional features like DNS lookups, disposable email detection, and more, ensuring a higher level of accuracy in email validation.
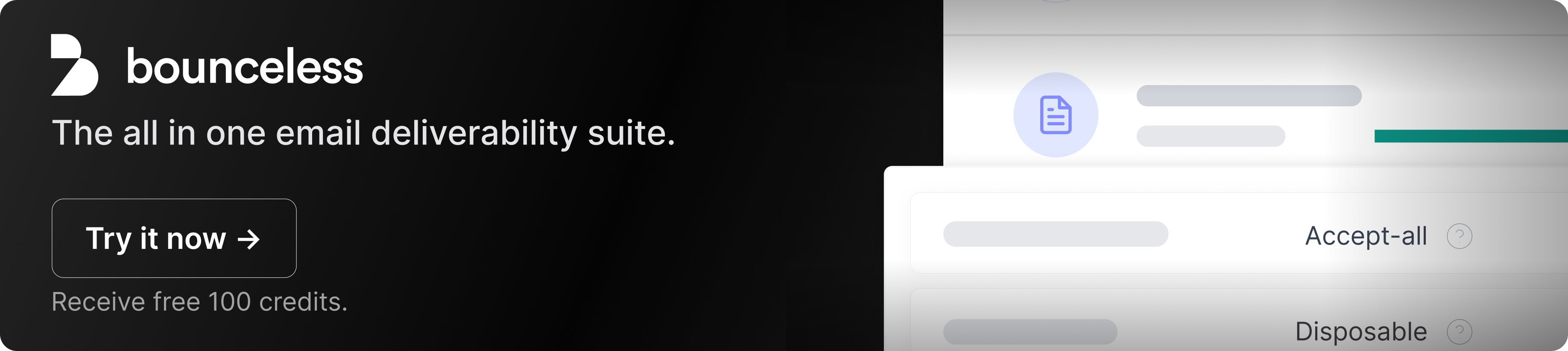
Implementing Email Validity Checker in Python
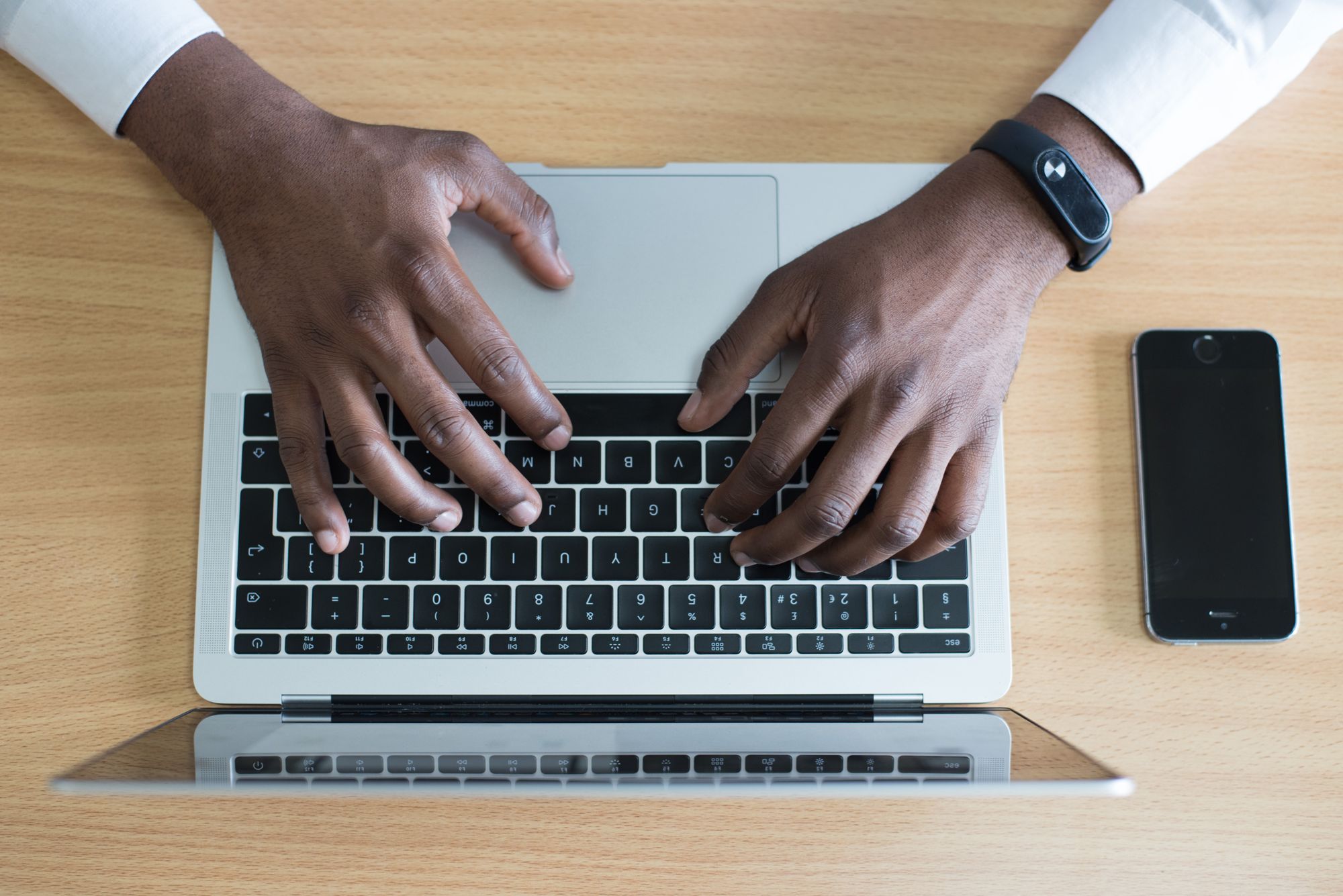
Now, let's delve into the implementation of an email validity checker in Python using various methods and libraries:
- Using Regular Expressions:The RegEx approach involves creating a pattern that represents the expected structure of a valid email address. By utilizing Python's
re
module, you can match the input email address against the defined pattern. However, it's important to note that RegEx alone cannot guarantee the existence or deliverability of the email domain. Here's an example:pythonCopy codeimport re def validate_email_regex(email): pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$' return re.match(pattern, email) is not None # Usageemail_address = "example@email.com"is_valid = validate_email_regex(email_address)
- Using the
email
Module:Python's built-inemail
module provides functionality to parse and validate email addresses. While it primarily focuses on the structure and format of the email, it may not perform domain-specific validation. Here's an example:pythonCopy codeimport email.utils def validate_email_builtin(email): try: email.utils.parseaddr(email) return True except email.utils.AddressParseError: return False # Usageemail_address = "example@email.com"is_valid = validate_email_builtin(email_address)
- Using the
email-validator
Library:Theemail-validator
library offers advanced email validation features, including domain existence checks, DNS lookups, disposable email detection, and more. It provides a comprehensive solution for email address verification. Here's an example:pythonCopy codefrom email_validator import validate_email, EmailNotValidError def validate_email_library(email): try: valid = validate_email(email) return valid.is_valid except EmailNotValidError: return False # Usageemail_address = "example@email.com"is_valid = validate_email_library(email_address)
Commonly Asked Questions
Q1. Can email validation guarantee the deliverability of an email?
No, email validation alone cannot guarantee the deliverability of an email. It focuses on checking the syntax, format, and domain existence of an email address. The deliverability of an email also depends on factors such as the recipient's email server configuration, spam filters, and the sender's reputation.
Q2. Which method or library should I choose for email validation in Python?
The choice of method or library depends on your specific requirements. If you need a basic validation of email syntax, regular expressions or the built-in email
module can suffice. However, for more advanced validation, including domain existence checks and deliverability, using a dedicated library like email-validator
is recommended.
Q3. How often should I validate email addresses in my application?
The frequency of email validation depends on your specific use case and requirements. If you deal with a static email list, validating it once during the data import or entry process may be sufficient. However, if you handle a dynamic list with frequent additions and updates, periodic validation can help maintain data accuracy.
Conclusion
Implementing an email validity checker in Python empowers you to ensure the accuracy, reliability, and efficiency of your email communications. Whether you choose to use regular expressions, the built-in email
module, or third-party libraries like email-validator
, Python provides an array of tools and techniques to validate email addresses. By incorporating robust email validation processes into your applications, you can enhance data integrity, improve communication, and optimize resource utilization. Embrace the power of Python's email validity checker and unlock the potential for high-quality email address verification.