Email validation is a crucial aspect of web development, especially when handling user-submitted data. JavaScript provides a powerful tool for validating email addresses using regular expressions (regex). In this comprehensive guide, we will delve into the intricacies of email validation in JavaScript, exploring different techniques and regex patterns to ensure accurate email validation. As an expert in the field, I will equip you with the knowledge and skills necessary to master email validation and optimize your form submission process.
Understanding Email Validation
Email validation refers to the process of verifying whether an email address is correctly formatted and follows the standard conventions. It helps ensure that users provide valid email addresses when submitting forms, signing up for accounts, or engaging in other online activities. By validating email addresses, you can enhance data integrity, prevent potential errors, and deliver a better user experience.
Regex Patterns for Email Validation
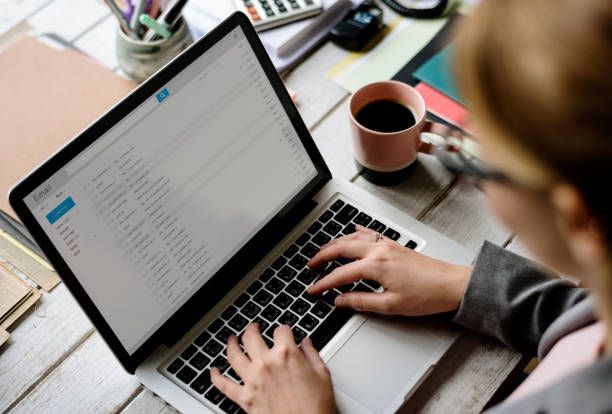
Regular expressions provide a powerful and flexible way to define patterns for email validation. Here are some commonly used regex patterns for email validation in JavaScript:
- Basic Email Validation: This simple regex pattern checks for the presence of an @ symbol and a domain extension. It ensures that the email address contains at least one character before and after the @ symbol.
- Strict Email Validation: A stricter regex pattern includes additional checks for email addresses, such as validating the format of the domain extension and disallowing special characters in the username.
- Comprehensive Email Validation: For a more comprehensive validation, a regex pattern can include checks for the username, domain, and subdomains. This pattern accounts for various scenarios, including hyphens, underscores, and multiple subdomains.
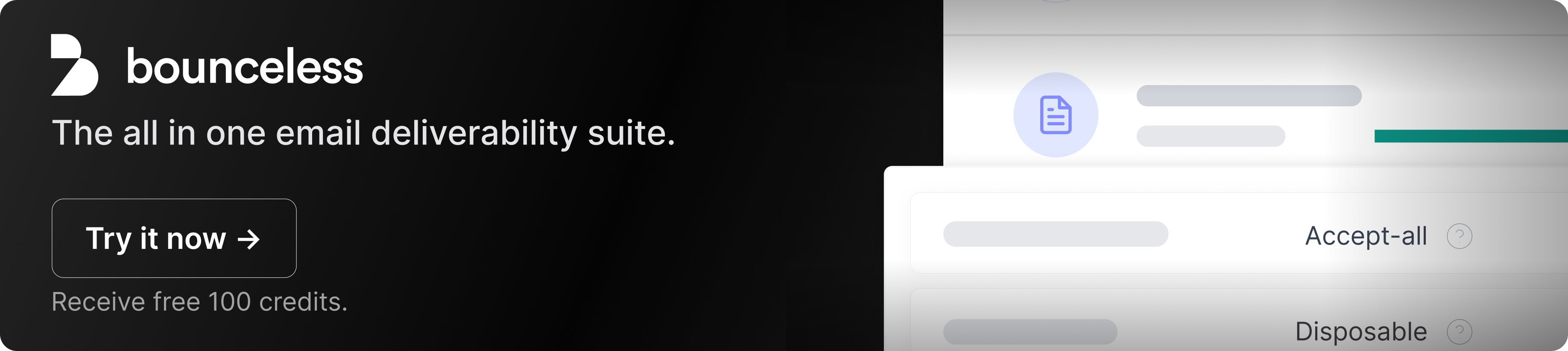
Implementing Email Validation in JavaScript
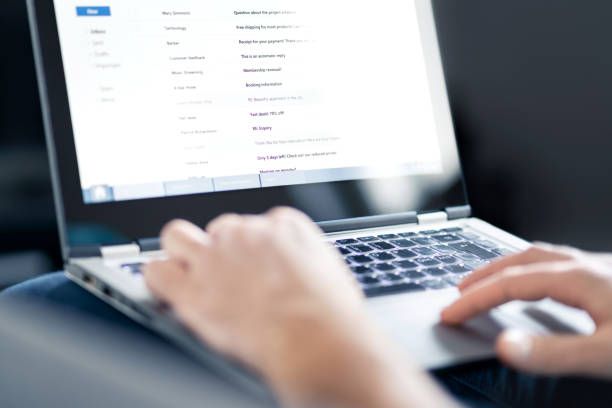
To implement email validation in JavaScript using regex, follow these steps:
- Create a Regular Expression: Define a regex pattern that matches the desired email format. Consider using one of the regex patterns mentioned earlier or customize the pattern to meet your specific requirements.
- Use the test() Method: Apply the regex pattern to the email address using the
test()
method of the regex object. This method returns a Boolean value indicating whether the email address matches the pattern. - Handle Validation Results: Based on the result of the
test()
method, provide appropriate feedback to the user. Display error messages if the email address is invalid or proceed with the form submission if it is valid.
Commonly Asked Questions
- Can email validation using regex guarantee 100% accuracy?While regex patterns can help validate email addresses effectively, they are not foolproof. Email validation using regex cannot verify the actual existence of an email address. It can only validate the format and structure. Additional server-side validation may be required for more robust verification.
- What are the potential drawbacks of using regex for email validation?Regex patterns can become complex, making them harder to maintain and understand. Additionally, as email standards evolve, regex patterns may need to be updated to accommodate new formats. It's crucial to regularly review and update your regex patterns to ensure accurate validation.
- Should email validation be done on the client-side or server-side?Client-side validation using JavaScript provides instant feedback to users, enhancing the user experience. However, server-side validation is essential to ensure data integrity and security. Implementing both client-side and server-side validation is the best practice for comprehensive email validation.
- Can I use a prebuilt library for email validation instead of writing regex patterns from scratch?Yes, several prebuilt JavaScript libraries exist that offer email validation functionality. These libraries often include comprehensive regex patterns and additional validation features. Consider using a well-maintained library to streamline your email validation process.
In conclusion, email validation using regular expressions in JavaScript is a powerful technique that helps ensure accurate and valid email addresses in web applications. By implementing appropriate regex patterns and following best practices, you can enhance data integrity, provide a better user experience, and optimize your form submission process. Remember to regularly review and update your regex patterns as email standards evolve. Mastering email validation in JavaScript is a valuable skill that will benefit your web development endeavors.