In the world of web development, validating user input is essential to ensure data accuracy and enhance the user experience. When it comes to email addresses, having a robust validation mechanism is particularly crucial. JavaScript, being a versatile programming language, provides developers with powerful tools to implement effective email validation. In this comprehensive article, we will explore the intricacies of email validation in JavaScript, empowering you to master this essential skill and deliver exceptional web applications.
Understanding the Significance of Email Validation
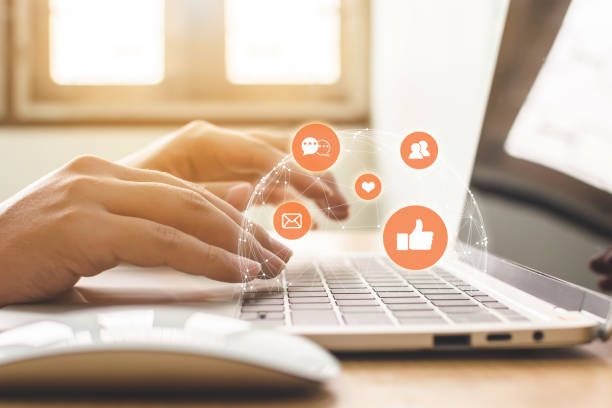
Email validation serves as a fundamental step in data validation, particularly when it involves user input. By implementing email validation in your web forms or applications, you can achieve the following benefits:
- Accurate Data Input: Email validation helps ensure that users provide a correctly formatted email address, reducing the risk of errors, typos, or invalid entries. This leads to accurate and reliable data that can be utilized for various purposes, such as user registration, communication, and marketing campaigns.
- Enhanced User Experience: Providing real-time feedback to users during the input process contributes to a seamless user experience. By validating email addresses on the client-side using JavaScript, you can instantly notify users about any errors or invalid entries, preventing form submissions and guiding them towards correction.
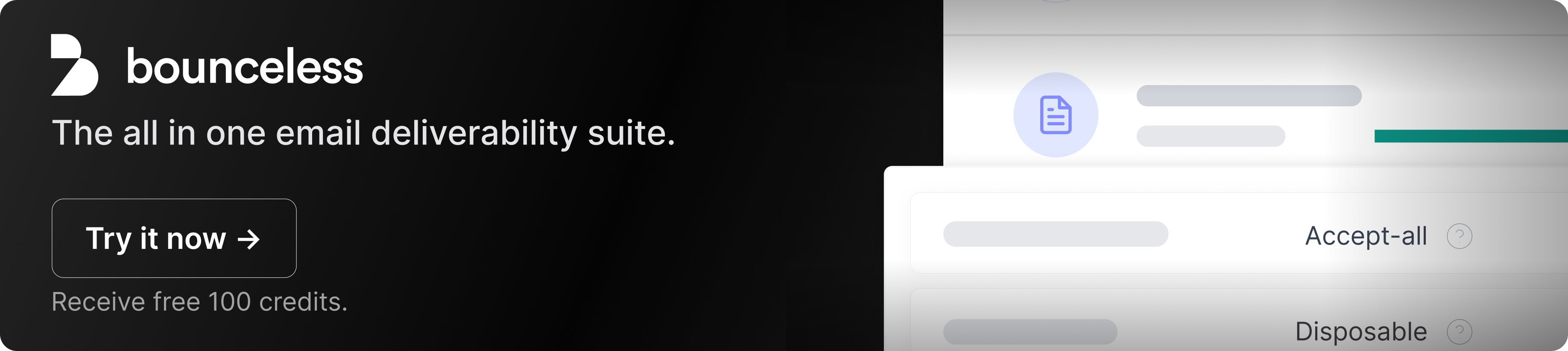
Implementing Email Validation in JavaScript
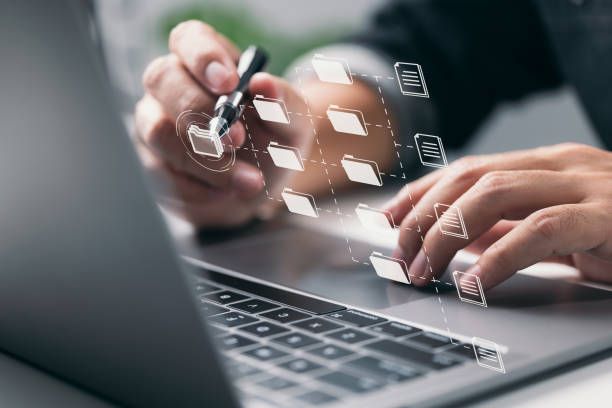
Now, let's dive into the process of implementing email validation in JavaScript. There are various approaches you can take, depending on the level of complexity and flexibility you desire. Here, we'll explore a simple yet effective method using regular expressions (regex) to validate email addresses. Regular expressions are patterns used to match and manipulate strings, making them ideal for email validation.
javascriptCopy codefunction validateEmail(email) { const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; return regex.test(email); }
In the example above, we define a function called validateEmail
that takes an email
parameter. The regular expression pattern /^[^\s@]+@[^\s@]+\.[^\s@]+$/
ensures that the email address consists of at least one character before the "@" symbol, followed by the "@" symbol itself, another set of characters before the domain name, a dot (.), and the domain name itself.
To utilize this function, you can call it when handling form submissions or during the input validation process:
javascriptCopy codeconst emailInput = document.getElementById('email'); const submitButton = document.getElementById('submit'); submitButton.addEventListener('click', function(event) { event.preventDefault(); const email = emailInput.value; if (validateEmail(email)) { // Valid email address // Proceed with form submission or desired action } else { // Invalid email address // Display an error message or provide feedback to the user } });
In this example, we retrieve the email input field element and the submit button element using the getElementById
method. We then add an event listener to the submit button, preventing the default form submission behavior. Inside the event listener, we retrieve the value of the email input field and validate it using the validateEmail
function. Based on the validation result, you can proceed with the desired action or display an error message.
Commonly Asked Questions (FAQs)
Q1: Is JavaScript email validation sufficient for complete security?
A1: JavaScript email validation primarily focuses on ensuring correct formatting and reducing user input errors. While it provides a convenient way to validate email addresses on the client-side, server-side validation is also essential to ensure comprehensive security and prevent malicious data submissions.
Q2: Are there any built-in JavaScript functions for email validation?
A2: JavaScript does not provide built-in functions specifically for email validation. However, regular expressions offer a powerful and flexible solution for implementing email validation logic.
Q3: Can I customize the email validation pattern to meet specific requirements?
A3: Absolutely! Regular expressions allow you to customize the email validation pattern based on your specific requirements. For instance, you can add additional constraints or allow certain variations based on your application's needs.
Q4: Is client-side email validation alone sufficient?
A4: Client-side email validation offers instant feedback to users and reduces unnecessary server requests. However, it should always be accompanied by server-side validation to ensure the integrity and security of the data being submitted.
Conclusion
Email validation in JavaScript plays a crucial role in ensuring accurate data input and enhancing the user experience. By leveraging the power of regular expressions, you can implement robust email validation mechanisms in your web applications. Remember to strike a balance between client-side and server-side validation for comprehensive security. Mastering email validation in JavaScript empowers you to deliver exceptional web forms and applications that provide real-time feedback, prevent erroneous data submissions, and optimize user interactions. Embrace the power of JavaScript email validation and elevate the quality of your web development projects.