In the digital era, email has become a fundamental means of communication. Whether you are developing a web application, creating an online form, or implementing a subscription system, validating email addresses is crucial. As a Java developer, you have the power to enhance the quality of your applications by implementing robust email validation techniques. In this comprehensive guide, we will explore the best practices and methods for email validation in Java. By the end, you will have the expertise to ensure the accuracy and integrity of email addresses, enhance user experience, and optimize the performance of your Java applications.
Understanding Email Validation in Java
Email validation in Java refers to the process of verifying the syntax and format of an email address to ensure it is valid and conforms to standard conventions. By validating email addresses, you can prevent invalid or malicious entries, enhance data integrity, and optimize your application's functionality. Here are some key points to consider when implementing email validation in Java:
- Regular Expressions (Regex): Regular expressions play a vital role in email validation. They provide a powerful and flexible mechanism for pattern matching and validating email addresses based on specific criteria.
- Syntax Validation: Syntax validation checks whether an email address conforms to the basic structure and format defined by email standards. It ensures that the address contains essential elements like a username, domain name, and a valid top-level domain (TLD).
- Domain Validation: Domain validation involves verifying the existence and accessibility of the domain associated with an email address. It ensures that the domain has valid DNS records and is active.
- Real-Time Validation vs. Batch Validation: Email validation can be performed in real-time as users enter their email addresses or in batch mode when processing a large dataset. The choice depends on the specific requirements of your application.
Implementing Email Validation in Java
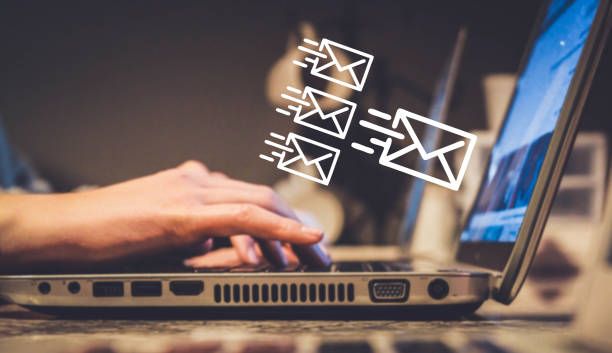
Now, let's dive into the implementation of email validation in Java. Here are some approaches you can follow:
- Regular Expression (Regex) Validation: One common method is to use regular expressions to define a pattern that represents a valid email address. You can utilize the
java.util.regex
package to match the email address against the specified regex pattern. For example:javaCopy codeimport java.util.regex.Pattern; import java.util.regex.Matcher; public class EmailValidator { private static final String EMAIL_REGEX = "^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$"; private static final Pattern EMAIL_PATTERN = Pattern.compile(EMAIL_REGEX); public static boolean validateEmail(String email) { Matcher matcher = EMAIL_PATTERN.matcher(email); return matcher.matches(); } }
- Using Libraries and APIs: Several Java libraries and APIs offer ready-made solutions for email validation. These libraries provide comprehensive functionality and handle various aspects of email validation, including syntax, domain, and even SMTP validation. Some popular libraries include Apache Commons Validator, javax.mail, and Hibernate Validator.
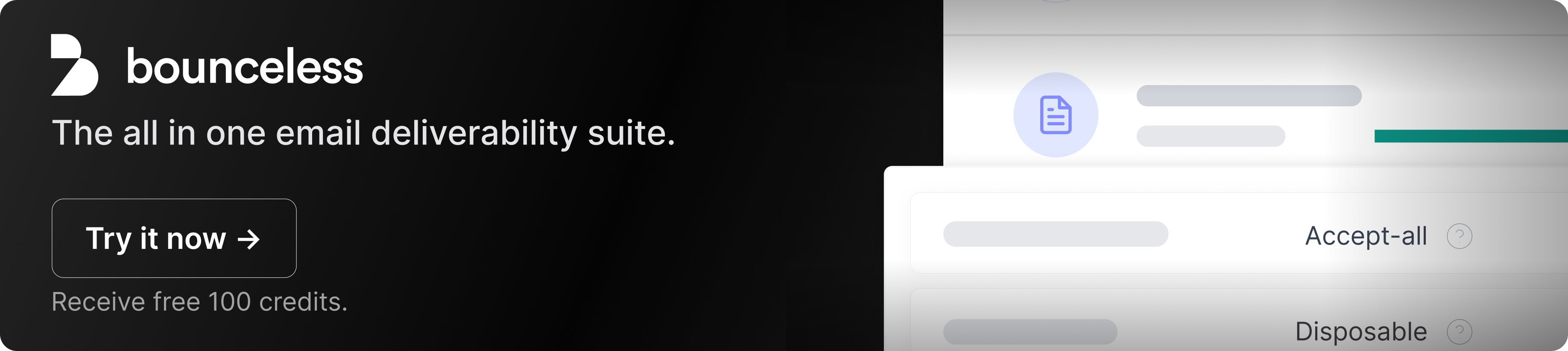
Best Practices for Email Validation in Java
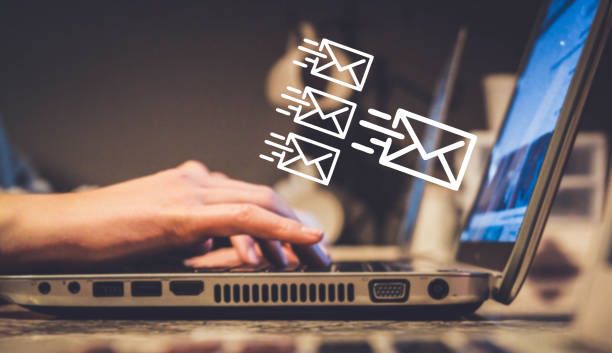
To ensure effective email validation in your Java applications, consider the following best practices:
- Validate Email at Multiple Stages: Validate email addresses at different stages of your application, such as during form submission, registration, or data processing. This helps catch errors early and provide prompt feedback to users.
- Validate Length and Character Limits: In addition to syntax validation, enforce sensible limits on the length of email addresses and the allowed characters. This helps prevent issues with storage, database constraints, and potential security vulnerabilities.
- Handle Internationalized Email Addresses: With the increasing globalization of the internet, it is important to support internationalized email addresses (addresses containing non-ASCII characters). Utilize libraries or built-in Java classes like
IDN
to handle such addresses correctly. - Regularly Update Email Validation Logic: Email standards and conventions may evolve over time. Stay up to date with the latest best practices and update your email validation logic accordingly to ensure compatibility and accuracy.
Frequently Asked Questions (FAQs)
Q1. Can I validate email addresses without using regular expressions in Java?
Yes, while regular expressions are a common approach, you can also utilize libraries and APIs that offer email validation functionality. Libraries like Apache Commons Validator provide ready-made methods to validate email addresses without explicitly using regular expressions.
Q2. How can I check if an email address exists and is active?
Email address existence and activity cannot be reliably determined solely through syntax validation. To verify the existence and activity of an email address, you can perform additional steps such as sending a verification email with a confirmation link or utilizing specialized email validation services that integrate with SMTP protocols.
Q3. Are there any performance considerations for email validation in Java?
Email validation, especially with complex regular expressions, can impact the performance of your application. To optimize performance, consider caching regex patterns, implementing multi-threading for batch validation, and leveraging libraries or APIs designed for efficient email validation.
Q4. Can I use the same email validation logic for client-side and server-side validation?
While the same regex pattern can be used for both client-side and server-side validation, it is generally recommended to perform server-side validation to ensure security and integrity. Client-side validation can be easily bypassed, so server-side validation acts as an additional layer of protection.
Q5. How do I handle temporary or disposable email addresses?
Temporary or disposable email addresses are commonly used for spam or fraudulent activities. To mitigate their usage, you can implement additional checks or integrate with third-party services that maintain databases of such email addresses.
By following these guidelines and utilizing the appropriate techniques, you can ensure accurate and reliable email validation in your Java applications. Implementing robust email validation not only enhances data integrity but also improves user experience and safeguards your application against potential vulnerabilities. Master the art of email validation in Java and empower your applications with efficient and reliable email handling capabilities.
Remember, email validation is a critical aspect of building robust and trustworthy applications, so investing time and effort into mastering these techniques is well worth it. Happy coding and may your Java applications excel in email validation!