In the digital landscape, email remains a primary means of communication, making email verification a crucial aspect of web development. With Node.js, a powerful server-side JavaScript runtime, you can implement robust email verification techniques to ensure secure communication and maintain the integrity of user data. In this comprehensive guide, we will explore the world of email verification in Node.js, empowering you to implement reliable email validation with confidence.
Understanding Email Verification in Node.js
Email verification in Node.js involves the process of confirming the authenticity and validity of an email address. It includes checking the email format, validating the domain existence, and ensuring that the email address can receive messages. Node.js provides various modules and libraries that simplify the email verification process, making it efficient and secure.
Step-by-Step Email Verification in Node.js: To implement email verification in Node.js, follow these steps:
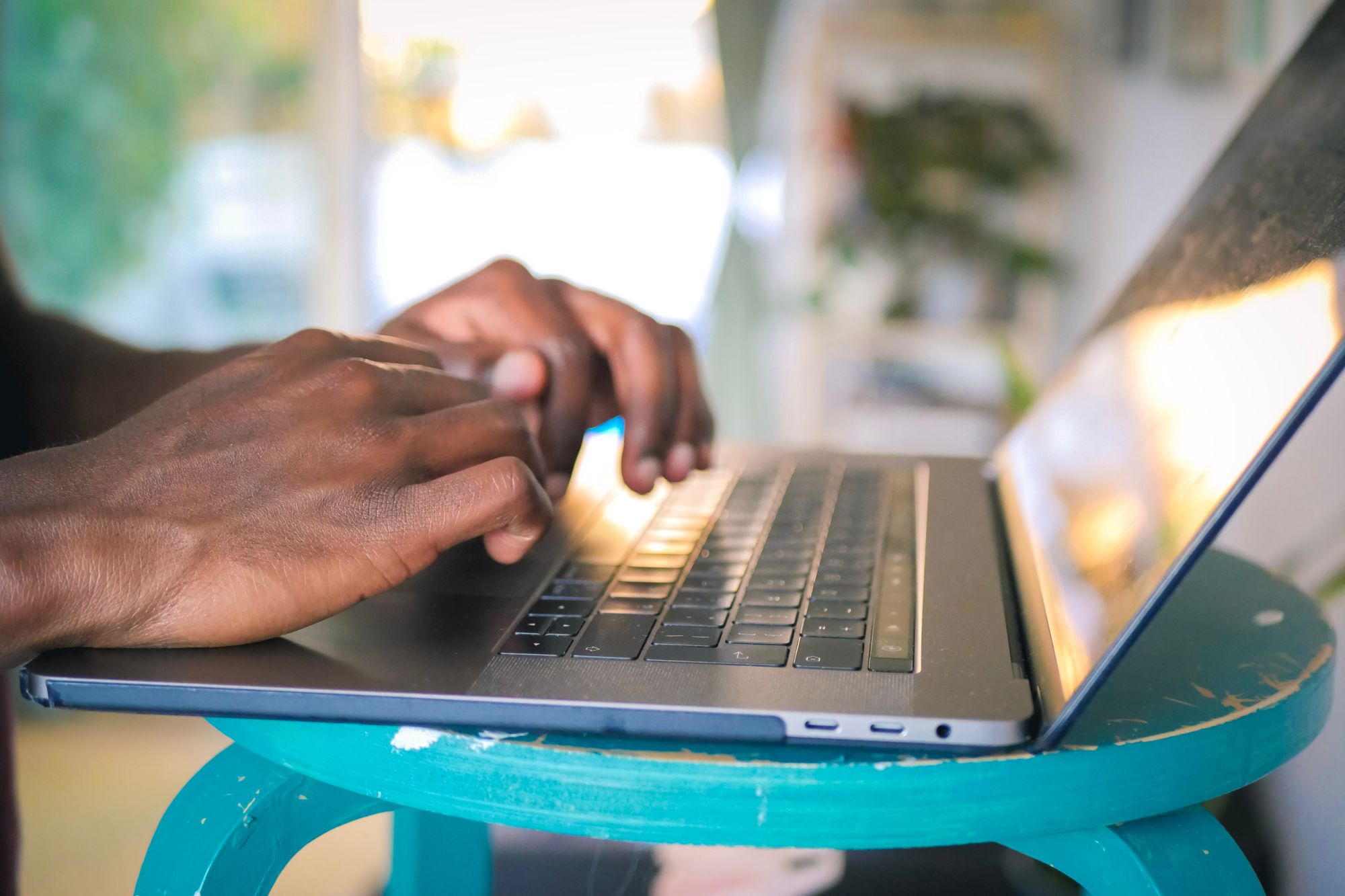
- Set up a Node.js project and install the necessary dependencies, such as Express and Nodemailer.
- Create an API endpoint or route to handle email verification requests.
- Retrieve the email address from the client-side and pass it to the server.
- Implement email validation using libraries or custom validation logic.
- Send a verification email containing a unique link to the provided email address.
- Handle the verification link on the server-side, validate it, and mark the email address as verified.
- Provide appropriate feedback to the user, confirming the successful email verification.
Here's an example of email verification implementation using Node.js and Express:javascriptCopy codeconst express = require('express'); const nodemailer = require('nodemailer'); const app = express(); const PORT = 3000; // Define an API endpoint for email verificationapp.get('/verify-email', (req, res) => { const email = req.query.email; // Perform email validation using libraries or custom logic // Send a verification email const transporter = nodemailer.createTransport({ // Configure the email transport options }); const mailOptions = { // Define the email content, including the verification link }; transporter.sendMail(mailOptions, (error, info) => { if (error) { // Handle error } else { // Handle success } }); }); app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
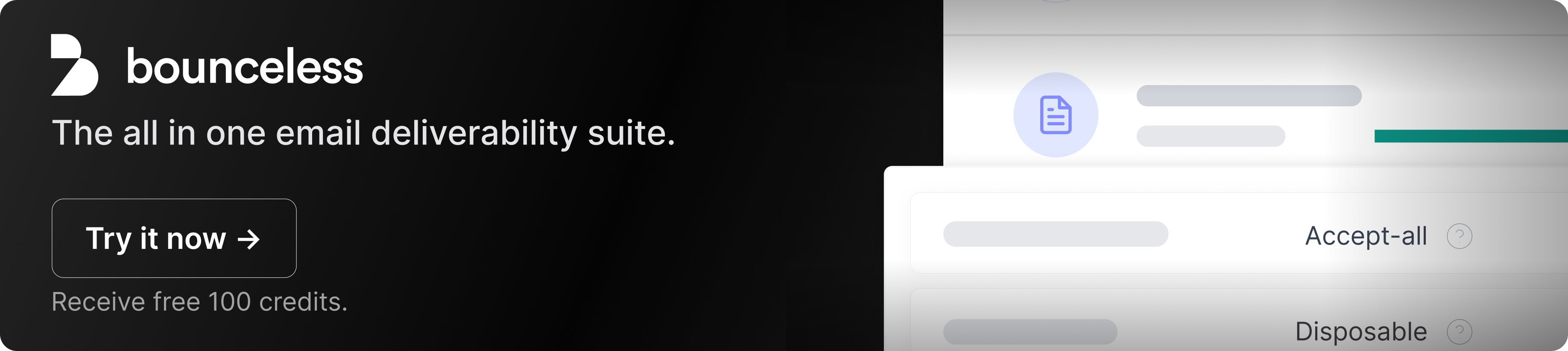
Best Practices for Email Verification in Node.js: When implementing email verification in Node.js, consider the following best practices:
- Validate emails on the server-side: Client-side validation can be bypassed by malicious users, so always perform server-side validation to ensure data integrity and security.
- Implement rate limiting: To prevent abuse and spam, implement rate limiting on your email verification endpoints to restrict the number of verification requests from a single IP address within a specific timeframe.
- Store email verification status: Maintain a record of the email verification status in your database to track and enforce verified email addresses.
- Secure your email transport: Use secure protocols (e.g., SMTP over TLS/SSL) and proper authentication mechanisms when configuring your email transport options. This ensures the confidentiality and integrity of the verification emails.
Commonly Asked Questions
Q1: Can I use third-party services for email verification in Node.js?
Yes, several third-party services, such as SendGrid, Mailgun, and AWS SES, offer email verification APIs that simplify the verification process. These services provide features like bulk email sending, analytics, and reputation monitoring.
Q2: How can I handle email verification asynchronously?
Node.js's non-blocking nature allows you to handle email verification asynchronously. You can use callback functions, promises, or async/await syntax to ensure smooth and efficient execution of email verification tasks without blocking the event loop.
Q3: Are there any specific libraries or modules available for email verification in Node.js?
Yes, there are popular Node.js libraries like email-validator, email-existence, and validator.js that provide email validation and verification functionalities. These libraries offer comprehensive validation rules, domain checks, and email format validation.
Q4: What measures should I take to prevent abuse during email verification?
To prevent abuse, implement measures such as rate limiting, CAPTCHA challenges, and email confirmation expiry. Rate limiting ensures that a user cannot flood your server with excessive verification requests, while CAPTCHA challenges help differentiate between human users and bots.
Conclusion
Email verification in Node.js plays a vital role in securing communication and maintaining data integrity in web applications. By implementing robust email validation techniques and following best practices, you can ensure the accuracy and security of user data. Combine server-side validation with client-side validation for comprehensive email verification. Leverage Node.js libraries and modules to simplify the implementation process. With email verification in Node.js, you can enhance the reliability, security, and trustworthiness of your applications, fostering strong user engagement and satisfaction.