Implementing email verification in a Laravel application is crucial for enhancing security, validating user email addresses, and improving user engagement. By verifying user emails, you can ensure that only legitimate users can access your application's features and functionalities. In this comprehensive guide, we will explore the process of implementing email verification in Laravel. We will cover the necessary steps, provide code examples, and offer best practices to help you integrate a robust email verification system into your Laravel project.
Understanding Laravel Email Verification
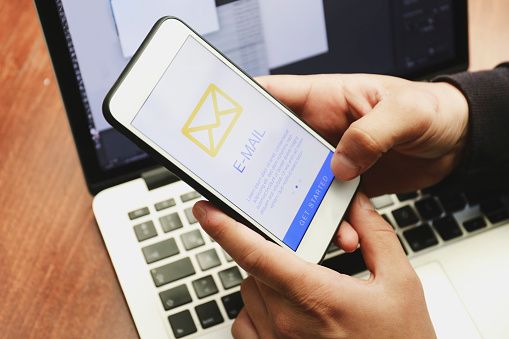
Email verification is a process that confirms the authenticity of user email addresses by sending a verification link or token to the provided email address. When users click on the verification link or enter the token, their email addresses are validated, and they gain access to the application's features. Laravel provides a convenient way to implement email verification with built-in features and functionalities.
Step-by-Step Implementation of Laravel Email Verification
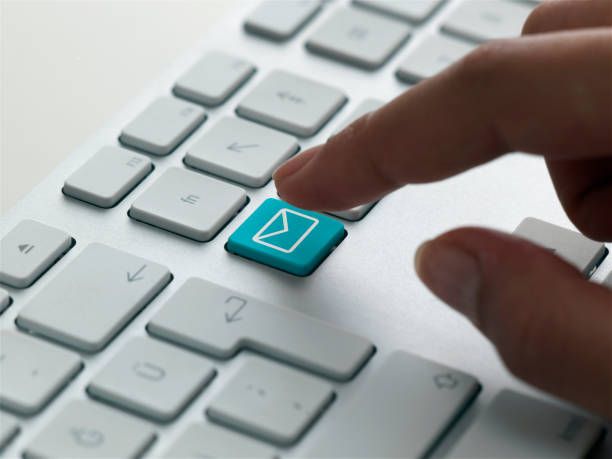
- Setting Up a New Laravel Project: Begin by creating a new Laravel project or using an existing one. Ensure that you have Laravel installed on your development environment. Open your terminal and run the necessary commands to create a new Laravel project.
- Configuring the Database: Configure your database connection in the
.env
file of your Laravel project. Specify the database driver, host, port, database name, username, and password. This step is crucial for storing user information, including email verification status. - Generating the User Authentication System: Use the Laravel
ui
command to generate the user authentication scaffolding. This command generates the necessary views, controllers, and routes for user authentication. Run the commandphp artisan ui vue --auth
to generate the scaffolding. - Configuring the Email Driver: Configure the email driver in the
.env
file to specify the service that will be used to send verification emails. Laravel supports various email drivers, such as SMTP, Mailgun, and Sendmail. Provide the necessary credentials and settings for the selected email driver. - Implementing Email Verification: In Laravel, email verification is implemented through the
MustVerifyEmail
trait, which can be added to theUser
model. The trait provides the necessary methods and functionalities for email verification. Add theMustVerifyEmail
trait to theUser
model to enable email verification. - Modifying the User Registration Process: Modify the user registration process to include email verification. When users register, Laravel automatically sends a verification email to the provided email address. Users need to click on the verification link to confirm their email addresses.
- Handling Email Verification: Laravel provides default routes and controllers for handling email verification. These routes are defined in the
web.php
file and theEmailVerificationController
class. You can customize these routes and controllers as per your project requirements. - Customizing Email Templates: Customize the email templates that Laravel uses for sending verification emails. Laravel provides default email templates, but you can modify them to match your application's branding and design. The email templates are located in the
resources/views/auth/
directory. - Testing the Email Verification Process: To ensure the successful implementation of email verification, thoroughly test the registration and verification process. Register new users, receive the verification emails, and click on the verification links to confirm that the email verification process works as expected.
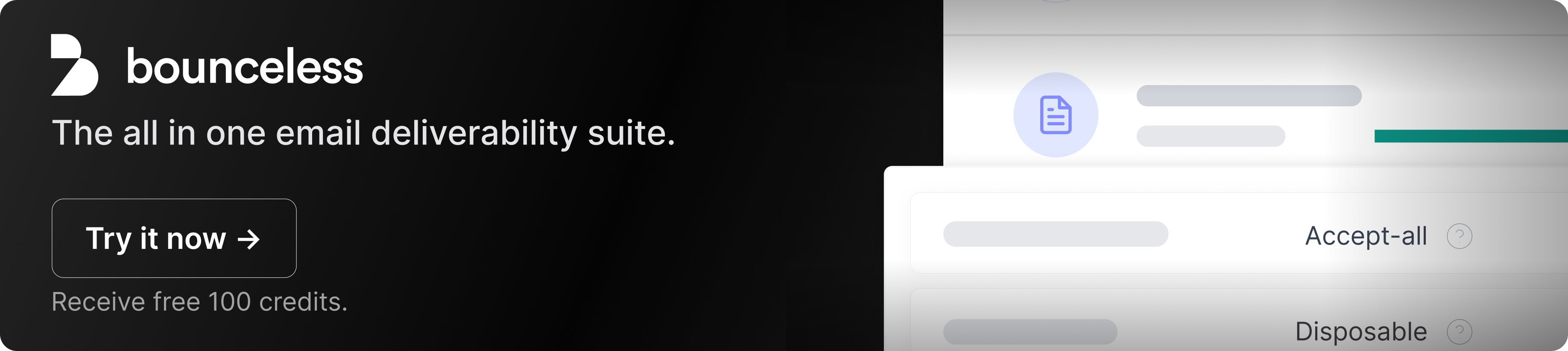
Best Practices for Laravel Email Verification
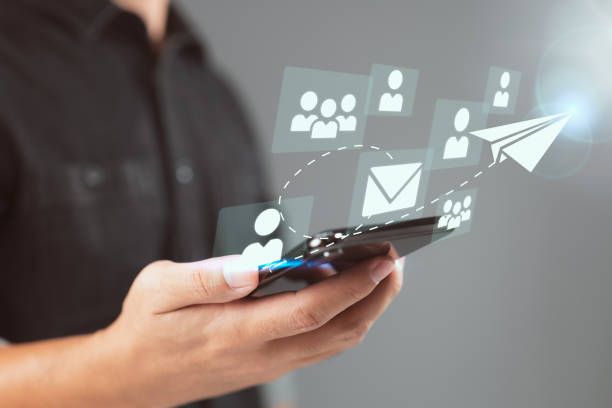
- Use Queues for Sending Emails: To optimize the performance of your application and prevent delays during email sending, utilize Laravel's queue system. By using queues, the email sending process is offloaded to a separate worker, improving the responsiveness of your application.
- Handle Expired Verification Links: Implement a mechanism to handle expired verification links. Set an expiration time for the verification links and provide users with an option to resend the verification email if the link has expired.
- Display User-Friendly Messages: Ensure that users receive clear and user-friendly messages throughout the email verification process. Communicate the success of email verification, display appropriate error messages for failed verification attempts, and guide users through the necessary steps.
- Implement Two-Factor Authentication: Consider implementing two-factor authentication (2FA) in addition to email verification for enhanced security. Two-factor authentication adds an extra layer of protection by requiring users to provide a second verification factor, such as a one-time password (OTP) sent to their mobile device.
Frequently Asked Questions
Q1: Can I customize the email verification process in Laravel?
A1: Yes, Laravel provides flexibility to customize the email verification process. You can modify the routes, controllers, email templates, and other aspects of the process to align with your application's requirements and branding.
Q2: How can I resend the email verification link to users?
A2: Laravel provides a convenient method to resend the email verification link. By implementing the resend
method in the EmailVerificationController
, you can resend the verification email to users who haven't yet verified their email addresses.
Q3: Can I use a different email service provider for sending verification emails?
A3: Yes, Laravel supports various email drivers, allowing you to use different email service providers. You can configure the desired email driver in the .env
file and provide the necessary credentials for the chosen provider.
Q4: Is email verification mandatory for all users in Laravel?
A4: No, email verification is not mandatory for all users. You have the flexibility to configure whether new users need to verify their email addresses before gaining access to your application's features. You can modify this behavior as per your project requirements.
Conclusion
Implementing email verification in your Laravel application is a crucial step to enhance security, validate user email addresses, and improve user engagement. With the step-by-step examples, best practices, and answers to commonly asked questions provided in this comprehensive guide, you have the knowledge and resources to successfully integrate email verification into your Laravel project. By incorporating a robust email verification system, you can increase user trust, mitigate the risk of fake accounts, and ensure a secure and engaging user experience in your Laravel application.