In Laravel, verifying user emails is a crucial step to ensure the authenticity and security of user accounts. By implementing email verification, you can confirm that the email address provided during registration belongs to the user and is valid. If you're looking to check if an email is verified in Laravel, you've come to the right place. As an expert in Laravel development, I will guide you through the process and provide comprehensive insights on email verification in Laravel.
Understanding Email Verification in Laravel: Email verification in Laravel involves sending a verification link to the user's email address upon registration. The user then clicks the link, confirming that the email address is valid and authenticating their account. This process enhances the security of user accounts and prevents unauthorized access.
Implementing Email Verification in Laravel: To check if an email is verified in Laravel, follow these steps:
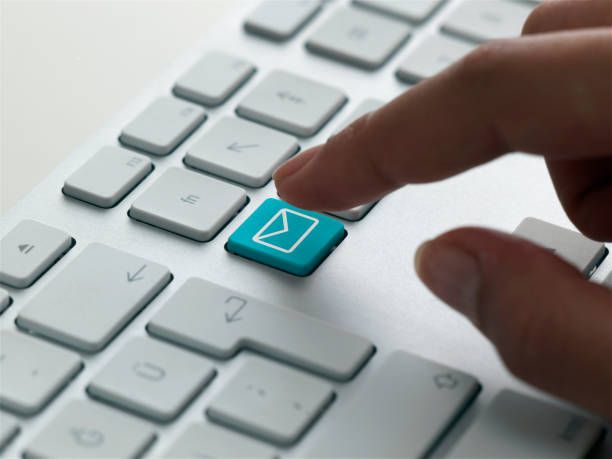
Step 1: Set Up Email Verification: Start by configuring Laravel's built-in email verification feature. This involves updating the User
model, creating the required routes, and configuring the email template for verification.
Step 2: Send the Verification Email: Once the user registers, generate a verification token and send an email containing a verification link. Laravel provides helper methods, such as sendEmailVerificationNotification()
, to simplify this process.
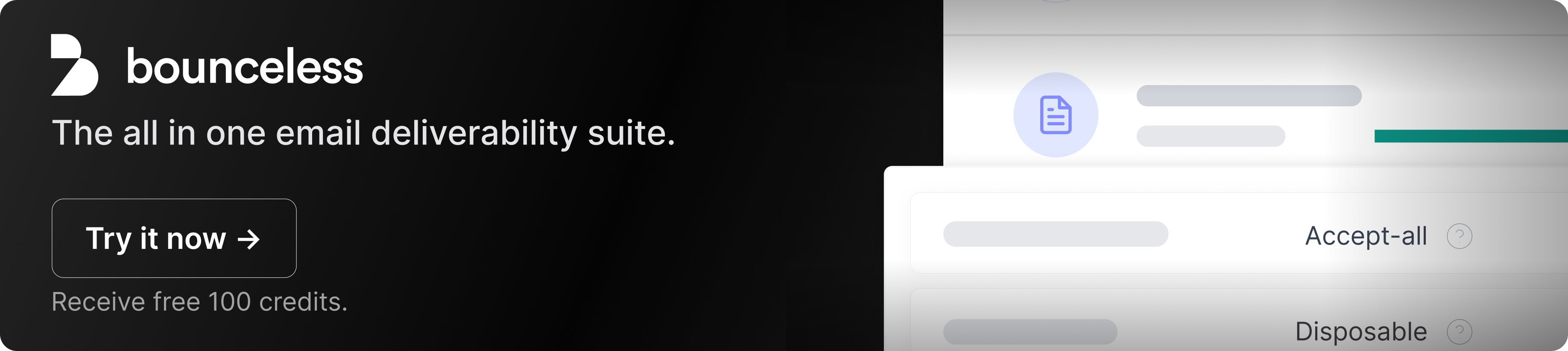
Step 3: Handle the Verification Route: Create a route that handles the verification link. Laravel's EmailVerification
middleware automatically handles the verification process and updates the user's email_verified_at
column in the database.
Step 4: Checking If Email is Verified: To check if an email is verified, you can use the email_verified_at
column on the User
model. If the value is null, the email is not verified. If the value is not null, the email is verified.
Code Example:phpCopy codeif (auth()->user()->email_verified_at) { // Email is verified} else { // Email is not verified}
Commonly Asked Questions
Q1: Can I customize the email verification process?
A1: Yes, you can customize the email verification process in Laravel. You can modify the email template, customize the verification link, and add additional steps or validation before marking the email as verified.
Q2: How can I resend the verification email?
A2: Laravel provides a resend
method on the EmailVerificationNotification
class that allows you to resend the verification email. You can trigger this method based on user actions or implement a dedicated route for resending verification emails.
Q3: Can I restrict certain actions until the email is verified?
A3: Yes, you can restrict user actions until the email is verified. By adding the verified
middleware to specific routes or controllers, you can ensure that only verified users can access certain features or perform certain actions.
Q4: Can I use third-party email providers for verification?
A4: Yes, Laravel's email verification feature supports integration with third-party email providers. You can configure Laravel to use SMTP, Mailgun, SendGrid, or other providers for sending verification emails.
Conclusion
Implementing email verification in Laravel is an essential step to ensure the authenticity and security of user accounts. By following the steps outlined in this expert guide, you can set up email verification and easily check if an email is verified in Laravel. Enhance the security of your application, build user trust, and protect against unauthorized access by implementing email verification in Laravel today.