Email verification is an essential aspect of web development and data validation. Regular expressions, commonly known as regex, provide a powerful tool for validating the format and structure of email addresses. In this comprehensive guide, we will explore how to perform email verification using regular expressions. As an expert in the field, we aim to equip you with the knowledge and skills necessary to implement robust email validation using regular expressions in your projects.
Understanding Regular Expressions (Regex)
Regular expressions are patterns used to match and manipulate strings. They provide a concise and flexible way to perform complex validations on text data. In the context of email verification, regex allows us to define a pattern that represents the valid structure of an email address.
Performing Email Validation with Regular Expressions
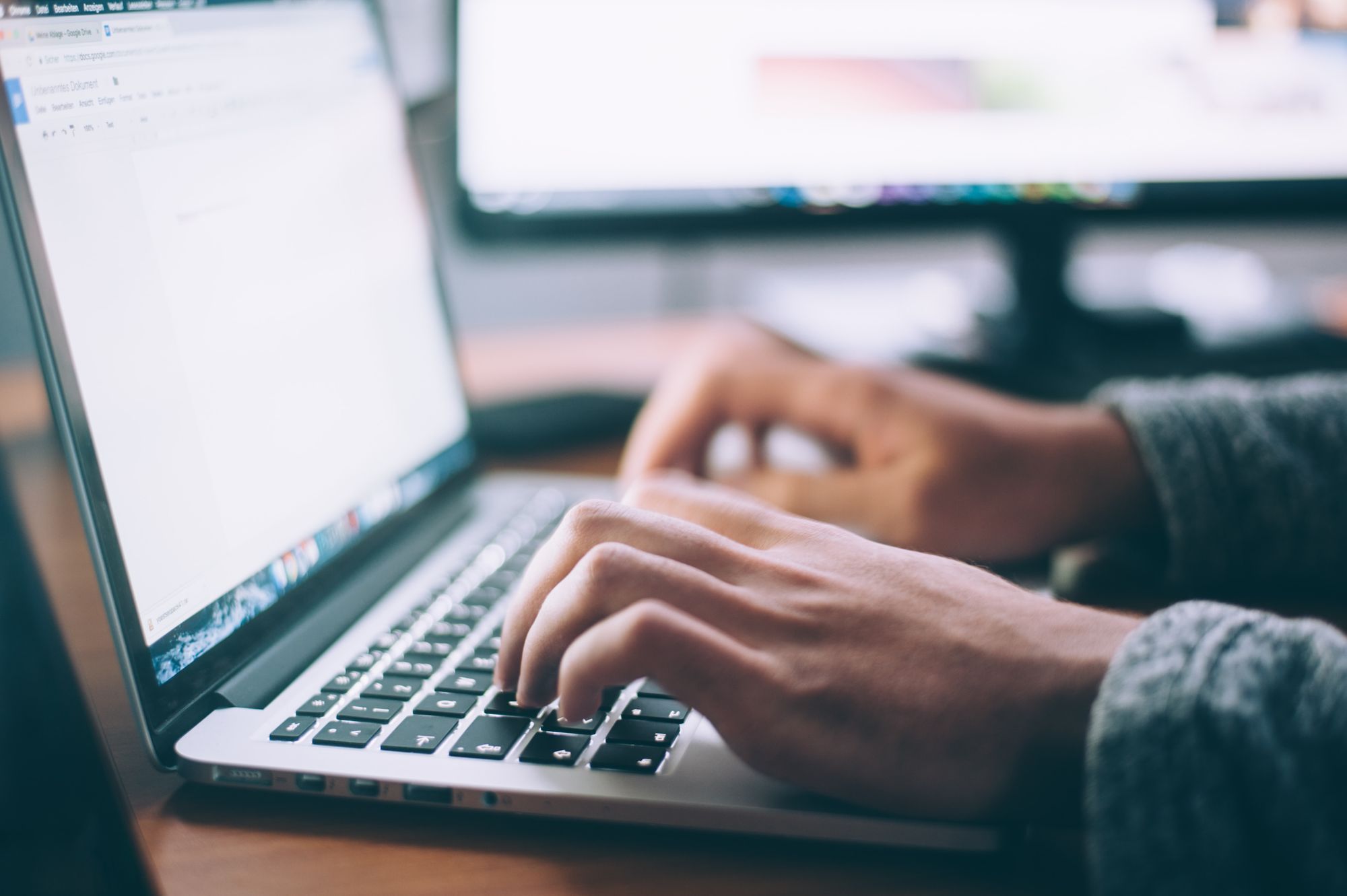
Let's delve into the process of validating email addresses using regular expressions:
- Defining the Regex Pattern: To validate an email address, we need to define a regex pattern that conforms to the standard structure of email addresses. This pattern typically includes rules for the username, domain, and top-level domain (TLD). There are various regex patterns available, and we will explore some commonly used ones later in this guide.
- Creating a Validation Function: Once we have the regex pattern, we can create a validation function that takes an email address as input and checks if it matches the defined regex pattern. If the email address matches the pattern, it is considered valid; otherwise, it is considered invalid.
- Implementing the Validation Function: Depending on the programming language or tool you are using, the implementation of the validation function may vary. Most programming languages have built-in functions or libraries that provide regex capabilities. You can leverage these tools to match the email address against the regex pattern and determine its validity.
- Integrating Validation in Your Application: Once the validation function is ready, you can integrate it into your application's form validation logic. By calling the validation function when a user submits an email address, you can provide immediate feedback on the validity of the input.
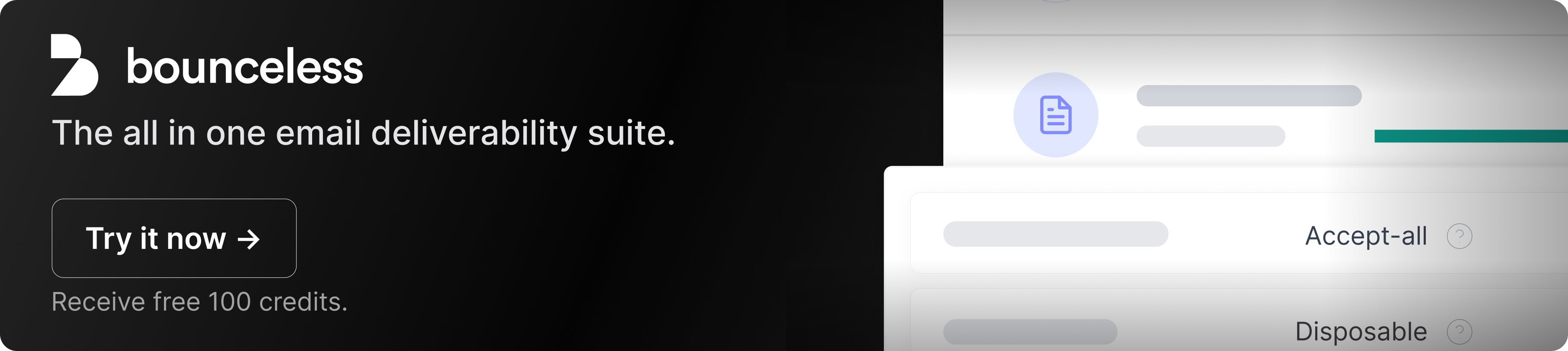
Commonly Used Regex Patterns for Email Validation
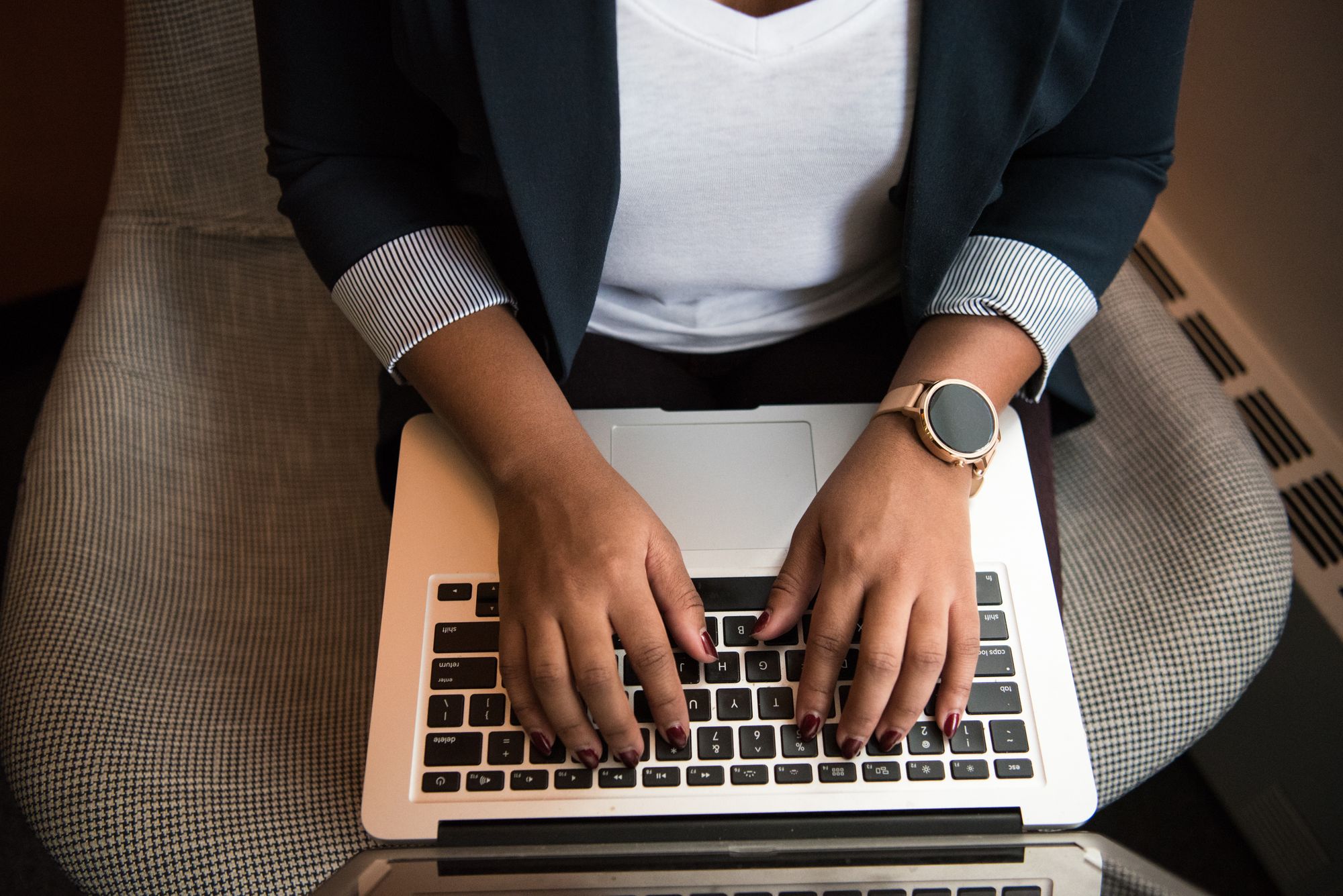
Here are some commonly used regex patterns for email validation:
- Basic Pattern: This simple pattern checks for the presence of an "@" symbol and a dot (".") in the domain section of the email address. While it provides a basic level of validation, it may not catch all possible edge cases.
- RFC 5322 Pattern: This pattern follows the specification outlined in RFC 5322 and provides a more comprehensive email validation. It covers a wider range of valid email formats, including complex domain structures and special characters.
- Customized Pattern: Depending on your specific requirements, you can customize a regex pattern to match the validation rules you need. This allows you to enforce additional constraints, such as specific TLDs or restrictions on username length.
Frequently Asked Questions
Here are answers to some commonly asked questions about email verification with regular expressions:
- Can regular expressions handle all edge cases in email validation?Regular expressions can handle most common email address formats, but it is challenging to create a single regex pattern that covers every possible edge case. It's important to strike a balance between strict validation and allowing for legitimate variations in email addresses.
- Is it better to use a basic or a more comprehensive regex pattern for email validation?The choice between a basic or comprehensive regex pattern depends on your specific requirements. If you need a simple validation, a basic pattern may suffice. However, if you want to ensure a high level of accuracy, it is recommended to use a more comprehensive pattern like the RFC 5322 pattern.
- Are regular expressions the only method for email validation?No, regular expressions are not the only method for email validation. Depending on the programming language or tool you are using, there may be built-in functions or libraries specifically designed for email validation. It's worth exploring these options before implementing regex-based validation.
- How can I test and debug my regex pattern for email validation?To test and debug your regex pattern, you can use online regex testers or tools provided by your programming environment. These tools allow you to input sample email addresses and see if they match the regex pattern correctly.
- Should email validation be performed on the client-side or server-side?It is recommended to perform email validation on both the client-side and server-side. Client-side validation provides immediate feedback to users, while server-side validation ensures data integrity and security.
Conclusion
By following the techniques and guidelines provided in this guide, you can implement robust email validation using regular expressions. Remember to consider your specific requirements and strike a balance between strict validation and allowing for legitimate email address variations.