If you are developing a mobile app with Xamarin Forms, you may need to
validate user input, especially when it comes to email addresses. Email
validation ensures that users enter a valid email address before submitting a
form or creating an account. In this article, we will discuss how to validate
email addresses in Xamarin Forms using Behaviors and Data Annotations.
Using Behaviors for Email Validation in Xamarin Forms
Behaviors are a powerful tool in Xamarin Forms that allow you to add custom
functionality to controls without subclassing them. You can use Behaviors to
perform input validation, formatting, or any other custom logic that you need.
To validate email addresses using Behaviors, you need to create a custom
Behavior that checks if the entered value matches the email format.
Here is an example of a custom Behavior for email validation:
public class EmailValidationBehavior : Behavior<Entry>{const string emailRegex = @"^([\w\.\-]+)@([\w\-]+)((\.(\w){2,3})+)$";static readonly BindablePropertyKey IsValidPropertyKey = BindableProperty.CreateReadOnly("IsValid", typeof(bool), typeof(EmailValidationBehavior), false);public static readonly BindableProperty IsValidProperty = IsValidPropertyKey.BindableProperty;public bool IsValid{get { return (bool)GetValue(IsValidProperty); }private set { SetValue(IsValidPropertyKey, value); }}protected override void OnAttachedTo(Entry bindable){bindable.TextChanged += HandleTextChanged;}protected override void OnDetachingFrom(Entry bindable){bindable.TextChanged -= HandleTextChanged;}void HandleTextChanged(object sender, TextChangedEventArgs e){IsValid = (Regex.IsMatch(e.NewTextValue, emailRegex, RegexOptions.IgnoreCase, TimeSpan.FromMilliseconds(250)));((Entry)sender).TextColor = IsValid ? Color.Default : Color.Red;}}
This Behavior adds a read-only IsValid property to the Entry control and sets
its value to true or false depending on whether the entered value matches the
email format. It also changes the text color of the Entry control to red if
the entered value is invalid.
To use this Behavior in your Xamarin Forms app, you need to add it to the
Entry control that you want to validate, like this:
<Entry><Entry.Behaviors><local:EmailValidationBehavior /></Entry.Behaviors></Entry>
This code snippet adds the EmailValidationBehavior to the Entry control and
enables email validation for it.
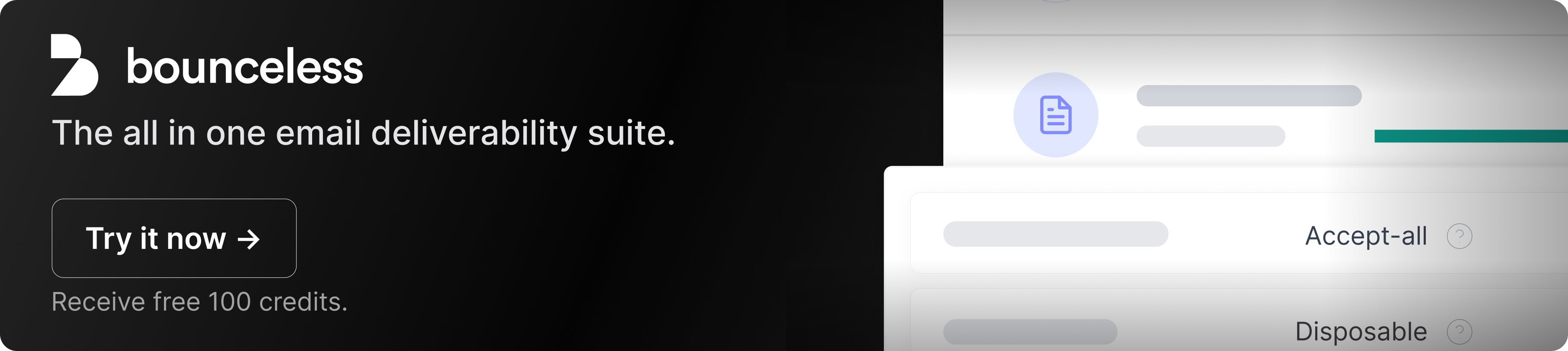
Using Data Annotations for Email Validation in Xamarin Forms
Data Annotations are another way to validate user input in Xamarin Forms. Data
Annotations are attributes that you can add to model properties to define
validation rules for them. To validate email addresses using Data Annotations,
you need to add the [EmailAddress] attribute to the property that represents
the email address.
Here is an example of a model class that uses Data Annotations for email
validation:
public class UserModel{[Required(ErrorMessage = "Email is required")][EmailAddress(ErrorMessage = "Email is not valid")]public string Email { get; set; }[Required(ErrorMessage = "Password is required")]public string Password { get; set; }}
This UserModel class has two properties: Email and Password. The Email
property has the [EmailAddress] attribute, which ensures that the entered
value is a valid email address. The Password property has the [Required]
attribute, which ensures that the entered value is not empty.
To use this UserModel class in your Xamarin Forms app, you need to bind the
Entry controls to its properties, like this:
<Entry Text="{Binding Email}" /><Entry Text="{Binding Password}" />
This code snippet binds the Text property of the Entry controls to the Email
and Password properties of the UserModel class.
Conclusion
Email validation is an essential part of mobile app development, and Xamarin
Forms provides several ways to implement it. You can use Behaviors to add
custom validation logic to controls or Data Annotations to define validation
rules for model properties. Both methods are easy to implement and can help
you create more robust and user-friendly mobile apps.