In Laravel 8, email validation is a crucial aspect of ensuring data integrity and delivering a seamless user experience. Validating email addresses not only prevents the entry of incorrect or fake email addresses but also enhances the reliability of your application's user data. In this comprehensive guide, we will delve into the world of email validation in Laravel 8, providing you with expert insights, best practices, and essential techniques to implement robust email verification in your application.
Laravel's Built-in Validation: A Solid Foundation
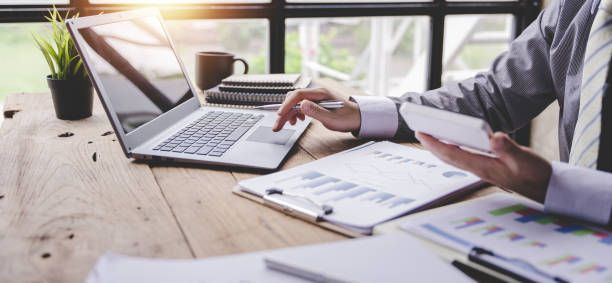
Laravel, being a popular PHP framework, offers a powerful and flexible built-in validation system. With Laravel's validation rules, you can easily validate email addresses in your application. By using the email
rule, Laravel automatically ensures that the input is a valid email address format. This rule checks the syntax of the email and confirms its validity based on established standards.
For example, to validate an email field in a form, you can use the following validation rule:phpCopy code$request->validate([ 'email' => 'required|email', ]);
This rule combination ensures that the field is not empty (required
) and that the input value is a valid email address (email
).
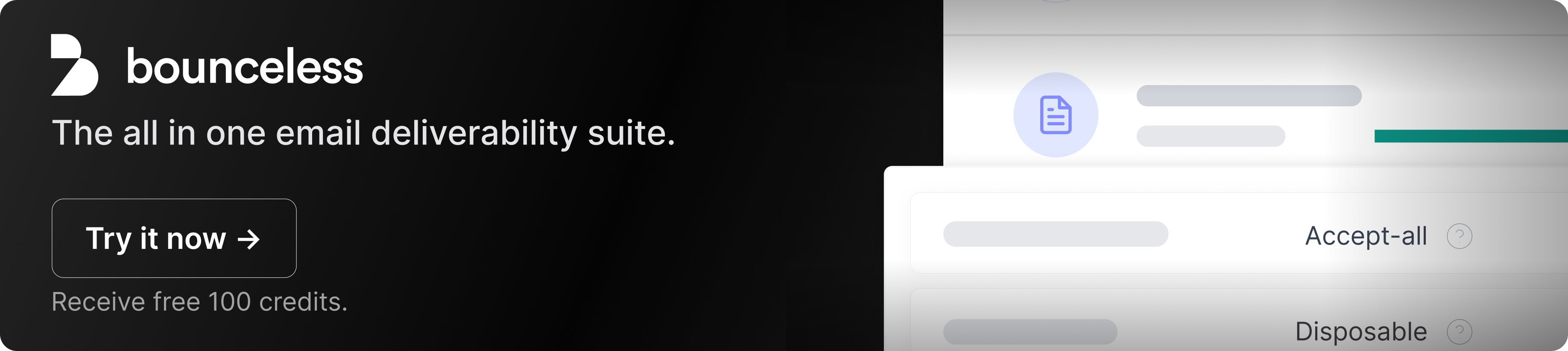
Custom Validation Rules: Tailoring Email Validation
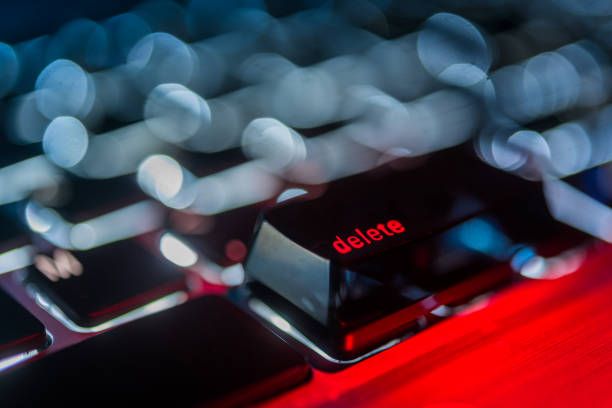
While Laravel provides a solid foundation for email validation, you may have specific requirements that go beyond basic syntax checks. In such cases, Laravel allows you to create custom validation rules tailored to your application's needs. You can define these rules in the App\Providers\AppServiceProvider
or any other service provider.
For instance, you can create a custom rule to verify if the email domain exists and is active:phpCopy codenamespace App\Rules; use Illuminate\Contracts\Validation\Rule; class ActiveEmailDomain implements Rule{ public function passes($attribute, $value) { // Perform domain validation logic here } public function message() { return 'The email domain is inactive or does not exist.'; } }
You can then use this custom rule in your validation:phpCopy code$request->validate([ 'email' => ['required', 'email', new ActiveEmailDomain], ]);
By leveraging custom validation rules, you can enhance email verification in your Laravel 8 application and ensure the validity and relevance of user-provided email addresses.
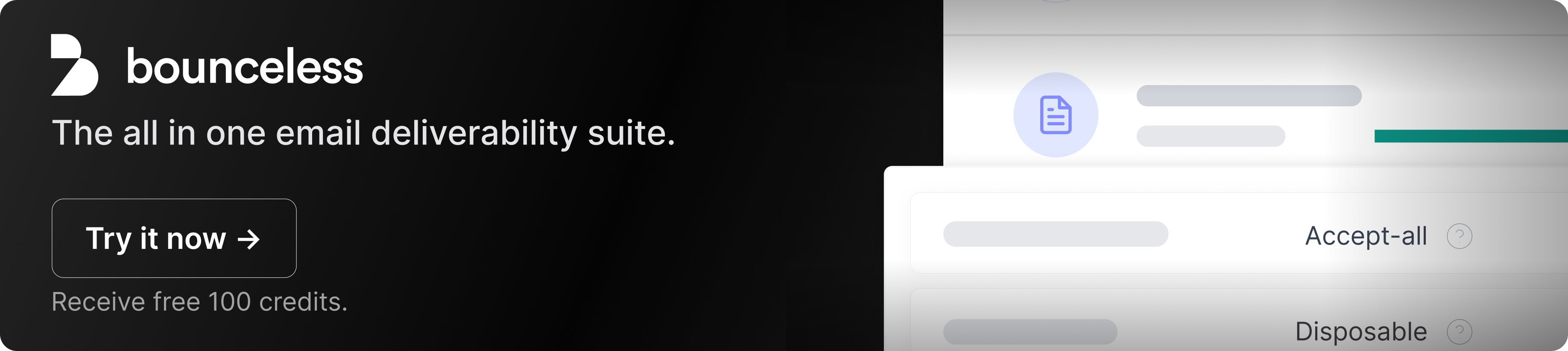
Third-Party Packages: Expanding Your Validation Arsenal
In addition to Laravel's built-in validation capabilities, several third-party packages can further augment your email validation capabilities in Laravel 8. These packages offer additional features, such as DNS checks, disposable email detection, and advanced validation techniques.
One such package is the "egulias/email-validator" package. It provides a comprehensive set of validation rules compliant with established email standards. This package allows you to perform advanced email validation checks, including domain existence, mailbox existence, and strict RFC compliance.
To install the "egulias/email-validator" package, you can use Composer:shellCopy codecomposer require egulias/email-validator
Once installed, you can use the package's validation rule in your Laravel 8 application:phpCopy code$request->validate([ 'email' => ['required', 'email', 'Egulias\\EmailValidator\\Validation\\RFCValidation'], ]);
By incorporating third-party packages like "egulias/email-validator," you can enhance your email validation capabilities and ensure the highest level of accuracy and integrity for the email addresses collected in your Laravel 8 application.
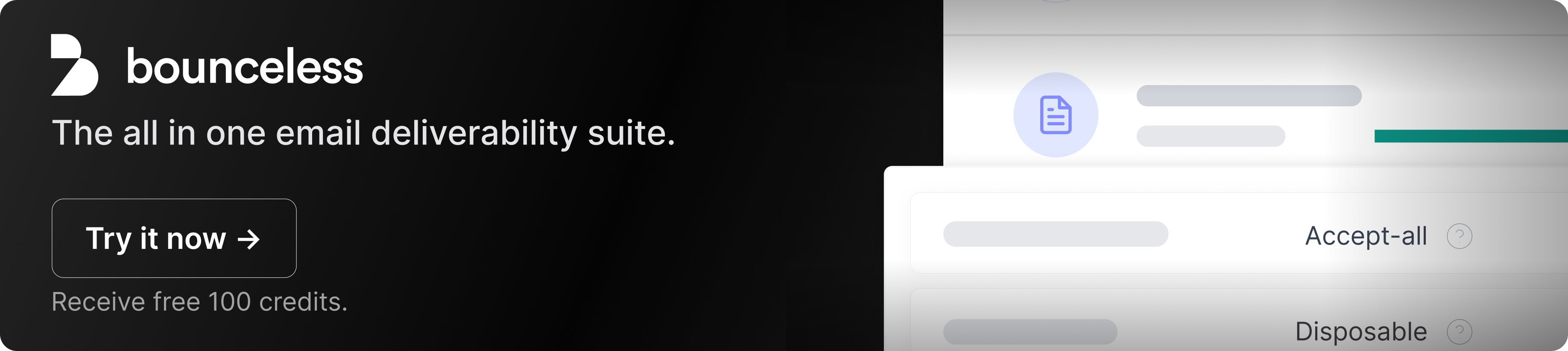
User Experience: Enhancing Email Validation Feedback
While validating email addresses is essential for data integrity, providing meaningful feedback to users is equally important for an exceptional user experience. Laravel's validation system allows you to easily customize error messages and tailor them to specific validation rules.
For example, you can customize the error message for the email validation rule:phpCopy code$request->validate([ 'email' => 'required|email', ], [ 'email.email' => 'Please enter a valid email address.', ]);
With this customization, if the user enters an invalid email address, they will receive a clear error message indicating the specific issue.
Frequently Asked Questions (FAQs)
Q1: How does email validation in Laravel 8 prevent fake or incorrect email addresses?
A1: Email validation in Laravel 8 verifies the syntax and format of email addresses. By using built-in validation rules or custom rules, you can prevent the entry of fake or incorrect email addresses in your application.
Q2: Can Laravel's email validation rules handle complex email validation requirements?
A2: Laravel's built-in email validation rules cover the basic syntax and format checks. For more complex requirements, you can create custom validation rules or leverage third-party packages to enhance email validation capabilities.
Q3: How can I provide real-time feedback during email address input?
A3: Laravel's validation system allows you to perform real-time validation using JavaScript frameworks like Vue.js or React.js. By leveraging these frameworks and making AJAX requests to your server, you can provide instant feedback to users as they enter their email addresses.
Q4: Are there any security considerations when validating email addresses in Laravel 8?
A4: While email validation itself does not pose security risks, it is important to handle user input securely and prevent vulnerabilities like cross-site scripting (XSS) attacks. Sanitizing and escaping user input and using Laravel's built-in protection mechanisms can help mitigate such risks.
Q5: Can email validation impact performance in Laravel 8?
A5: Basic email validation in Laravel 8 has a negligible impact on performance. However, advanced validation techniques involving external API calls or extensive checks may introduce slight performance overhead. It is important to balance validation requirements with performance considerations.
In conclusion, email validation in Laravel 8 is a vital aspect of maintaining data integrity and delivering an exceptional user experience. By utilizing Laravel's built-in validation system, creating custom validation rules, and leveraging third-party packages, you can ensure the accuracy and validity of email addresses in your application. Implementing robust email validation techniques will not only enhance the reliability of your user data but also foster trust and engagement with your audience. Master the art of email validation in Laravel 8 and elevate your application to new heights of data integrity and user satisfaction.