Email validation is a critical aspect of developing robust and reliable Kotlin applications. Verifying the correctness of email addresses entered by users helps maintain data integrity, prevent errors, and ensure effective communication. In this comprehensive guide, we will delve into the world of email validation in Kotlin. We will explore different approaches, techniques, and libraries available to validate email addresses and provide a seamless user experience. By the end of this article, you will have the knowledge and skills to implement robust email validation in your Kotlin projects.
Importance of Email Validation
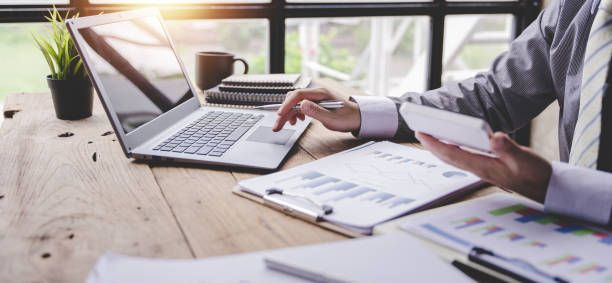
Validating email addresses is crucial for several reasons. It ensures that the email address provided by the user conforms to the standard email format, minimizing the risk of erroneous data. Proper email validation also enhances user experience by preventing users from entering incorrect or invalid email addresses, reducing frustration and errors during registration or communication processes. Additionally, valid email addresses are essential for effective email communication and maintaining a clean and accurate user database.
Regular Expressions (Regex) for Email Validation
One common approach to email validation is using regular expressions (regex). Regular expressions are powerful patterns that match specific patterns of text. For email validation, a regex pattern can be used to validate the structure and format of an email address. Kotlin provides built-in support for regex, allowing developers to define and apply email validation patterns easily. Various online resources, such as the Stack Overflow posts mentioned earlier, offer regex patterns suitable for email validation.
Android-specific Email Validation
If you are developing an Android application using Kotlin, you can leverage the Android framework's built-in email validation capabilities. The android.util.Patterns.EMAIL_ADDRESS
pattern provided by the Android SDK allows you to validate email addresses using a simple and efficient approach. This pattern can be used to check whether the entered email address matches the standard email format defined by the Android platform.
Third-Party Libraries for Email Validation
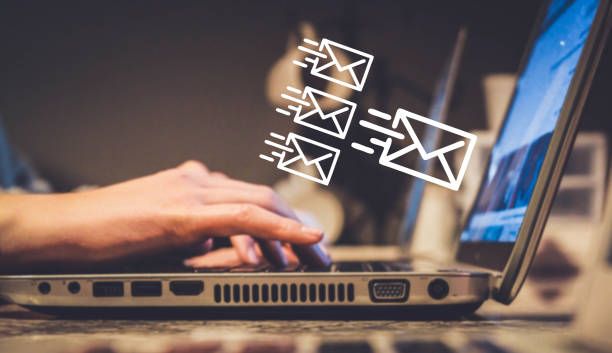
In addition to regex and built-in Android patterns, several third-party libraries can simplify the process of email validation in Kotlin. These libraries offer comprehensive features, including advanced email address validation, error handling, and localization support. Some popular libraries include Apache Commons Validator, JavaMail API, and KEmailValidator. These libraries provide additional functionalities, such as checking domain existence or verifying the mailbox's validity through SMTP requests.
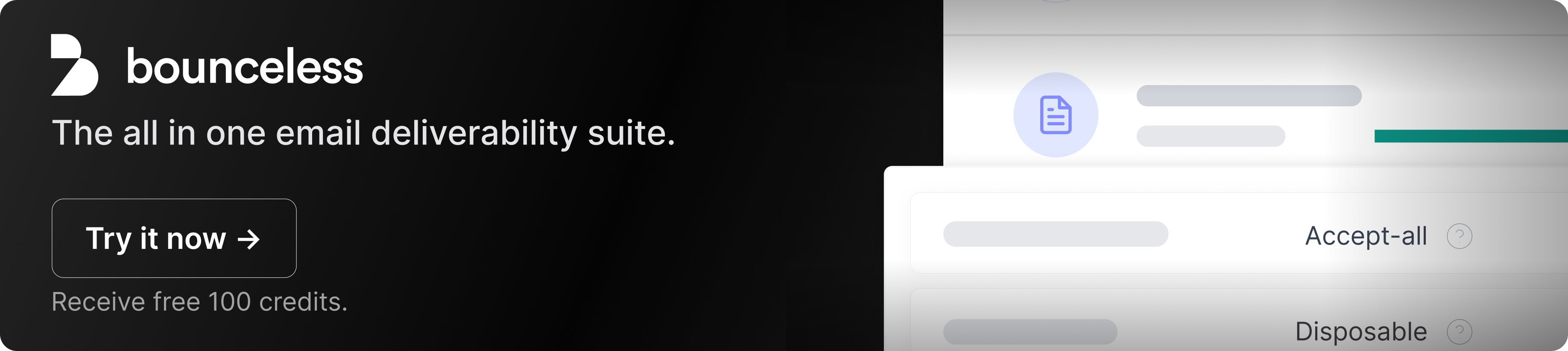
Custom Email Validation Logic
In certain scenarios, you may have specific business requirements or constraints that go beyond standard email validation. In such cases, implementing custom email validation logic becomes necessary. By writing custom validation code, you can define specific rules, such as checking against a list of restricted domains or enforcing additional constraints. Kotlin's flexible syntax and programming constructs allow you to implement tailored email validation logic according to your application's unique needs.
Commonly Asked Questions
Q1. Why is email validation important?
Email validation is crucial for data integrity, user experience, and effective communication. Validating email addresses ensures that the entered addresses adhere to the standard email format, reducing errors and improving data quality. It also enhances user experience by preventing users from entering incorrect email addresses and allows for accurate communication through email channels.
Q2. Can I use regular expressions for email validation in Kotlin?
Yes, regular expressions (regex) are commonly used for email validation in Kotlin. Kotlin provides built-in support for regex, making it convenient to define and apply email validation patterns. By using regex patterns suitable for email validation, you can verify the structure and format of an email address.
Q3. Are there any Kotlin-specific libraries for email validation?
While there are no Kotlin-specific libraries dedicated solely to email validation, you can utilize various Java libraries seamlessly in Kotlin projects. Apache Commons Validator, JavaMail API, and KEmailValidator are popular libraries that offer comprehensive email validation features, including domain existence checks and mailbox verification.
Q4. How can I implement custom email validation logic in Kotlin?
To implement custom email validation logic in Kotlin, you can leverage the language's flexibility and programming constructs. By writing custom code, you can define specific rules and constraints beyond standard email validation. This may involve checking against a list of restricted domains, enforcing additional formatting rules, or implementing domain-specific validation logic.
Q5. Is email validation necessary for Android applications developed with Kotlin?
Yes, email validation is essential for Android applications developed with Kotlin. Validating email addresses helps ensure the accuracy of user data, improves the user experience, and prevents errors during registration or communication processes. Android provides built-in patterns and libraries to simplify email validation in Kotlin.
Conclusion:
Email validation is a critical aspect of Kotlin application development, ensuring data accuracy, user satisfaction, and effective communication. By leveraging regular expressions, built-in Android patterns, or third-party libraries, you can implement robust email validation in your Kotlin projects. Additionally, custom validation logic allows you to tailor the validation process to your specific application requirements. With a thorough understanding of email validation techniques in Kotlin, you can enhance your application's reliability and provide a seamless user experience. Embrace the power of email validation and create Kotlin applications that handle email addresses with precision and accuracy.