Are you tired of receiving emails with invalid addresses? Do you want to
ensure that your website users enter valid email addresses? If so, you need to
implement email validation in JavaScript. In this article, we will explain
what email validation is, how it works, and how to implement it in your
JavaScript code. We will also answer some of the most commonly asked questions
about email validation.
What is Email Validation?
Email validation is the process of verifying whether an email address is valid
or not. This involves checking the syntax of the email address to ensure that
it follows the correct format, as well as checking whether the domain name and
mailbox exist and can receive emails.
Why is Email Validation Important?
Email validation is important for several reasons:
- It helps to prevent spam and fraudulent emails from being sent to your inbox.
- It ensures that your website users enter valid email addresses, which can help to improve the accuracy of your data and prevent errors.
- It can help to improve the deliverability of your emails, as emails with invalid addresses are more likely to be rejected by mail servers.
How Does Email Validation Work?
Email validation typically involves two steps:
- Checking the syntax of the email address: This involves checking whether the email address follows the correct format. This can be done using regular expressions or built-in JavaScript functions such as the
indexOf()
method. - Checking the domain and mailbox: This involves checking whether the domain name and mailbox exist and can receive emails. This can be done using various techniques, such as sending a test email or using an API.
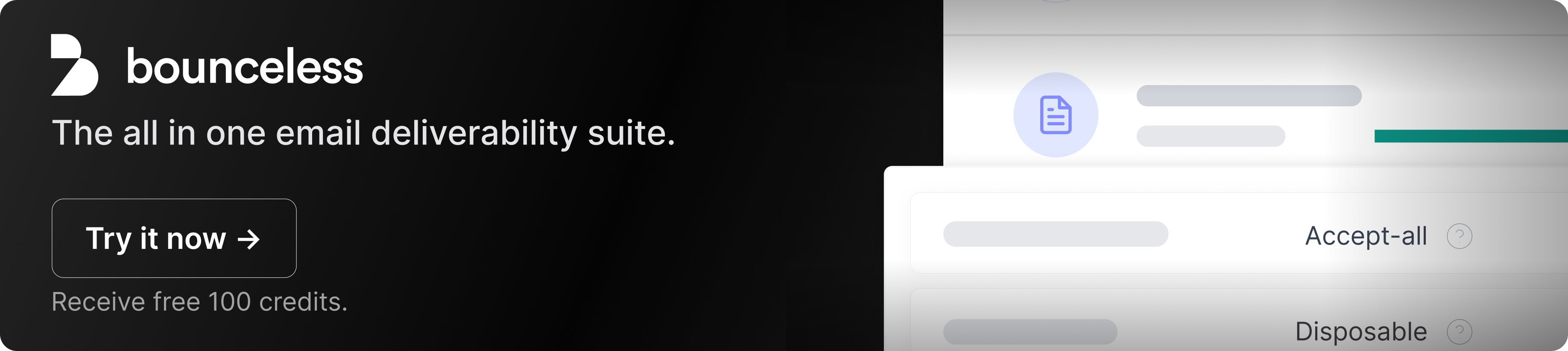
Implementing Email Validation in JavaScript
Implementing email validation in JavaScript is relatively straightforward. You
can use regular expressions or built-in JavaScript functions to check the
syntax of the email address, and you can use various techniques to check the
domain and mailbox.
Here is an example of how to implement email validation using a regular
expression:
function validateEmail(email) {var re = /^(([^<>()[\]\.,;:\s@"]+(\.[^<>()[\]\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;return re.test(email);}var email = "example@example.com";if (validateEmail(email)) {console.log("Valid email address");} else {console.log("Invalid email address");}
In this example, we use a regular expression to check the syntax of the email
address. The regular expression checks whether the email address follows the
correct format, including the presence of an @ symbol, a domain name, and a
top-level domain.
Here is an example of how to implement email validation using an API:
async function validateEmail(email) {const response = await fetch(`https://api.abstractapi.com/v1/email/validate?api_key=YOUR_API_KEY&email;=${email}`);const data = await response.json();return data.is_valid;}const email = "example@example.com";validateEmail(email).then(result => {if (result) {console.log("Valid email address");} else {console.log("Invalid email address");}});
In this example, we use the Abstract API to check the validity of the email
address. We send a request to the API with the email address, and the API
returns a response indicating whether the email address is valid or not.
FAQs
What is the correct format for an email address?
The correct format for an email address is:
username@domain.com
The username can contain letters, numbers, and special characters such as dots
and hyphens. The domain name must be a valid domain name, such as gmail.com or
yahoo.com.
Can email validation be bypassed?
Yes, email validation can be bypassed by spammers and fraudsters who use fake
or disposable email addresses. However, implementing email validation can help
to reduce the number of invalid email addresses that are submitted to your
website.
What are the benefits of using an API for email validation?
Using an API for email validation can provide several benefits, such as:
- Improved accuracy: APIs can use advanced algorithms to check the validity of an email address, which can improve the accuracy of the results.
- Improved speed: APIs can perform email validation quickly, which can help to improve the user experience.
- Reduced workload: APIs can automate the process of email validation, which can reduce the workload on your website.