As a developer, email validation is an essential aspect of building any web
application, and Angular provides a straightforward way to accomplish this
task. In this article, I’ll guide you through the process of implementing
email validation in an Angular application.
What is Email Validation?
Email validation is the process of verifying whether an email address is valid
or not. It is a critical component of any web application that involves user
registration or communication via email. Email validation helps to ensure that
only legitimate email addresses are used, reducing the likelihood of spam and
other malicious activities.
Implementing Email Validation in Angular
Angular provides several ways to implement email validation, including using
the built-in EmailValidator class or creating a custom validator. Let's take a
closer look at these two options:
Using the EmailValidator Class
The EmailValidator class is built into the Angular Forms module and provides a
simple way to validate email addresses. To use the EmailValidator class, you
need to import it from the Angular Forms module and add it to the validators
array of your form control:
import { Component } from '@angular/core';import { FormGroup, FormControl, Validators } from '@angular/forms';@Component({selector: 'app-root',template: `<form [formGroup]="form"><input type="email" formControlName="email"></form>`,})export class AppComponent {form = new FormGroup({email: new FormControl('', [Validators.required, Validators.email])});}
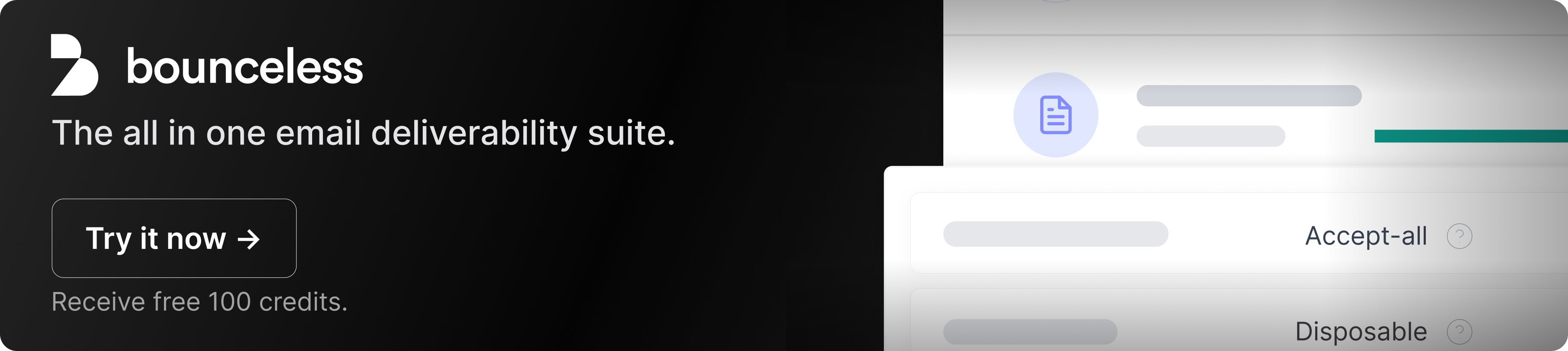
Creating a Custom Validator
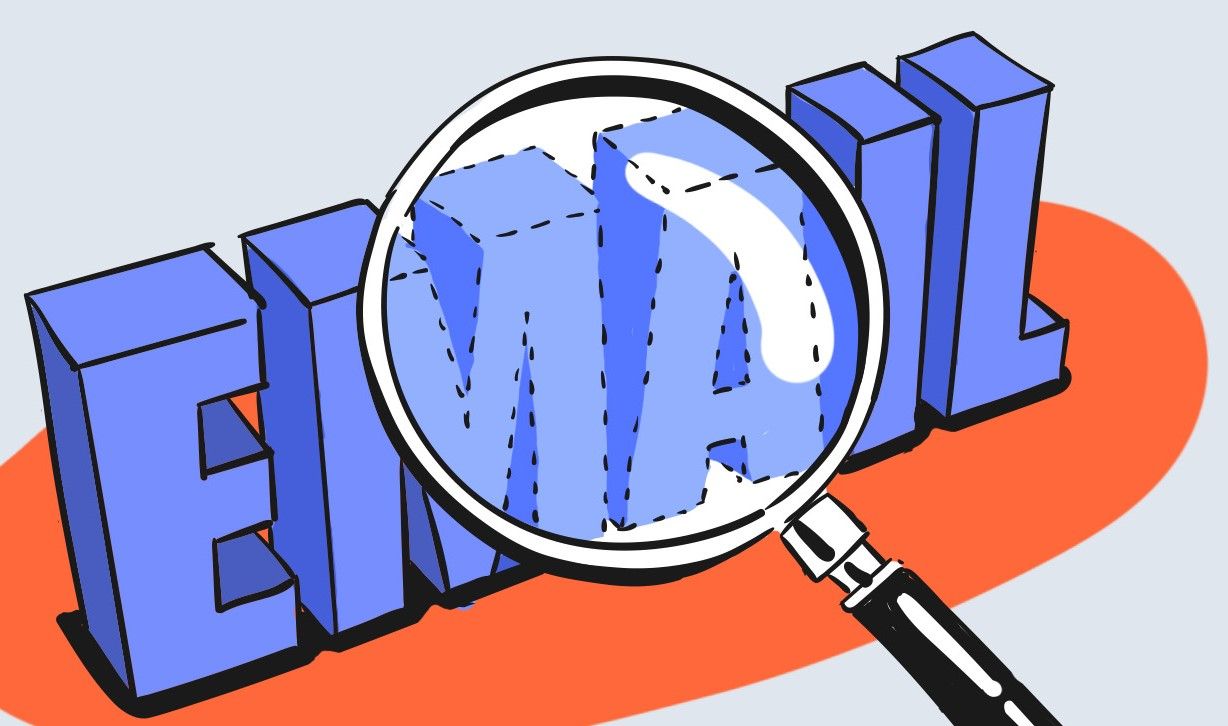
If you need more control over the email validation process, you can create a
custom validator. To create a custom validator, you need to implement the
Validator interface and define a validate method that takes a form control as
an argument:
import { Component } from '@angular/core';import { FormGroup, FormControl, ValidatorFn, Validators } from '@angular/forms';function emailValidator(): ValidatorFn {return (control: FormControl): {[key: string]: any} | null => {const valid = /\S+@\S+\.\S+/.test(control.value);return valid ? null : { invalidEmail: { value: control.value } };};}@Component({selector: 'app-root',template: `<form [formGroup]="form"><input type="email" formControlName="email"></form>`,})export class AppComponent {form = new FormGroup({email: new FormControl('', [Validators.required, emailValidator()])});}
Conclusion
Implementing email validation in an Angular application is a critical step in
ensuring the security and reliability of your application. Whether you choose
to use the built-in EmailValidator class or create a custom validator, the
process is straightforward and can be accomplished in just a few lines of
code. By following the steps outlined in this article, you’ll be able to add
email validation to your Angular forms with ease.
Frequently Asked Questions
What does email validation do?
Email validation is the process of verifying whether an email address is valid
or not. It helps to ensure that only legitimate email addresses are used,
reducing the likelihood of spam and other malicious activities.
How do I implement email validation in Angular?
Angular provides several ways to implement email validation, including using
the built-in EmailValidator class or creating a custom validator. Both
approaches are straightforward and can be accomplished with just a few lines
of code.
What is the EmailValidator class in Angular?
The EmailValidator class is a built-in class in the Angular Forms module that
provides a simple way to validate email addresses. It can be used by importing
it from the Angular Forms module and adding it to the validators array of your
form control.
Can I create a custom email validator in Angular?
Yes, you can create a custom email validator in Angular by implementing the
Validator interface and defining a validate method that takes a form control
as an argument. This approach provides more control over the email validation
process.