In today's digital age, email communication plays a pivotal role in both personal and professional spheres. However, ensuring the validity of email addresses is crucial to avoid bounced emails, improve deliverability, and maintain a clean database. Python, a powerful and versatile programming language, offers various techniques and libraries to efficiently check the validity of email addresses. In this comprehensive guide, we will explore different approaches, popular libraries, and best practices for implementing an email checker in Python.
Understanding Email Validation
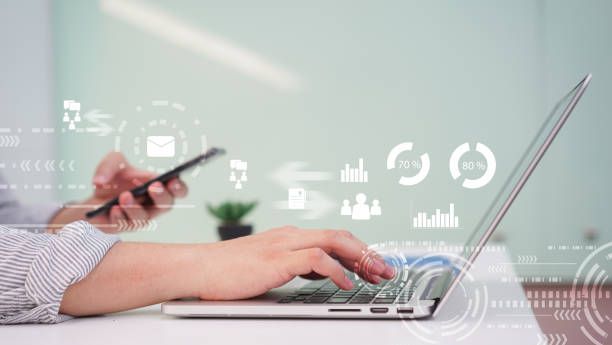
Before delving into the intricacies of implementing an email checker in Python, let's first understand the concept of email validation. Email validation is the process of verifying whether an email address conforms to a specific format and is associated with an existing domain. It helps in identifying potential errors, typos, or malicious inputs before sending or storing the email address.
Validating an email address typically involves checking its syntax, domain existence, and mailbox existence. Although Python provides built-in modules to handle basic email validation, relying solely on these modules may not be sufficient for comprehensive email verification. Let's explore some effective techniques and libraries to enhance the email checking process in Python.
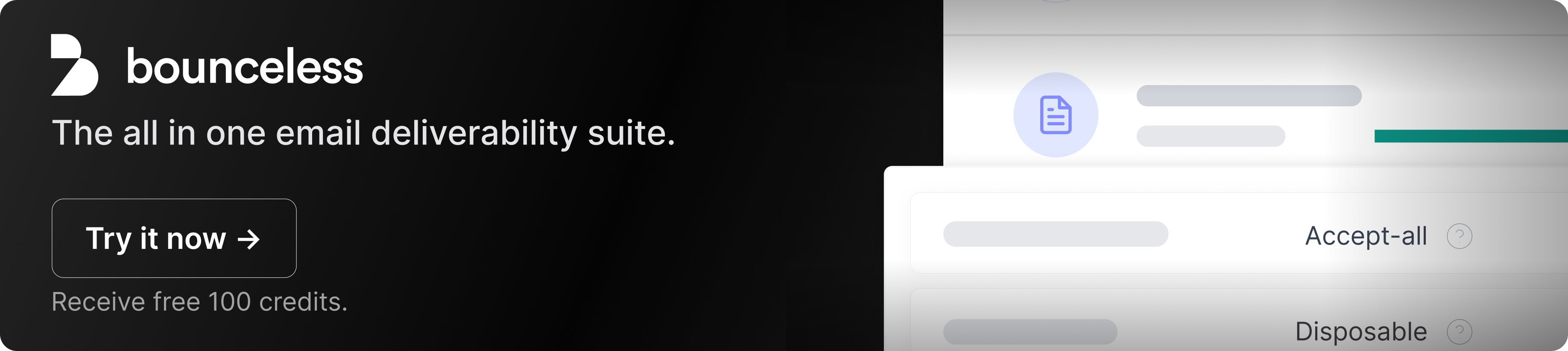
Python Libraries for Email Validation
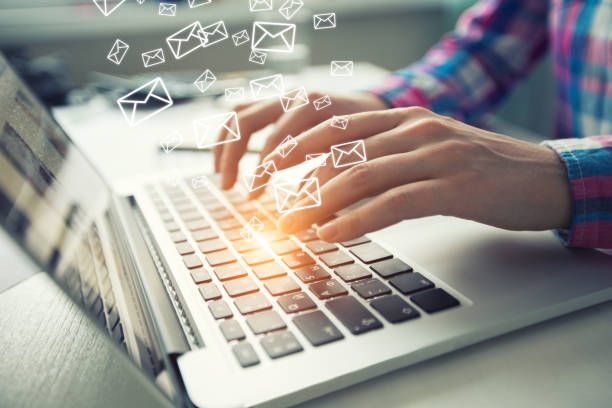
Regular Expressions (RegEx):
Python's built-in 're' module offers robust support for regular expressions, enabling developers to define custom patterns for email address validation. While regular expressions can be powerful, constructing an accurate email validation pattern can be challenging due to the complexity of the email address structure.
Email-validator Library
The 'email-validator' library is a popular choice for email validation in Python. It provides a simple and straightforward interface to validate email addresses against the relevant standards and guidelines. With the 'email-validator' library, you can easily check the syntax, domain existence, and even perform DNS and SMTP checks to validate the mailbox existence.
Validate-email-address Library
Another notable library for email validation in Python is the 'validate-email-address' library. This library offers comprehensive email address validation capabilities, including syntax validation, domain and mailbox existence checks, and even disposable email address detection. It provides a reliable and efficient solution for verifying email addresses within your Python projects.
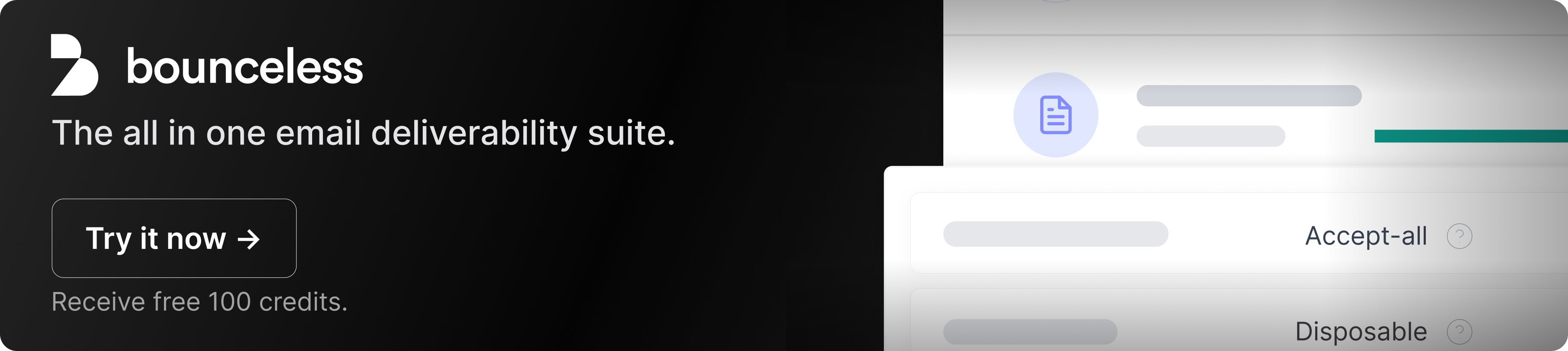
Implementing Email Checker in Python:
Now that we are familiar with the available libraries, let's dive into the implementation of an email checker in Python. The following steps outline a basic approach to validate an email address using the 'email-validator' library:
Installing the 'email-validator' library:
- Open your command prompt or terminal.
- Execute the following command:
pip install email-validator
Importing the necessary modules:pythonCopy codefrom email_validator import validate_email, EmailNotValidError
Validating an email address:pythonCopy codedef check_email(email): try: valid = validate_email(email) return True except EmailNotValidError: return False
Testing the email checker:pythonCopy codeemail_to_check = 'example@example.com'is_valid = check_email(email_to_check) print(f"The email address {email_to_check} is valid: {is_valid}")
By following these steps, you can easily integrate email validation capabilities into your Python projects. Feel free to explore the functionalities provided by different libraries to customize the validation process according to your requirements.
Frequently Asked Questions (FAQs):
How important is email validation?
Email validation is crucial for maintaining a clean and accurate email database, enhancing deliverability rates, and preventing potential issues like bounced emails or spam complaints. It helps in ensuring effective communication and building reliable customer relationships.
What are the consequences of not validating email addresses?
Failing to validate email addresses can lead to undelivered messages, damaged sender reputation, increased bounce rates, and wasted resources. It may also result in your emails being flagged as spam, impacting the overall email marketing effectiveness.
Can email validation guarantee deliverability?
Email validation improves the likelihood of deliverability, but it does not guarantee it. Other factors, such as email content, sender reputation, and recipient mail server policies, also influence successful email delivery.
Is it necessary to use third-party libraries for email validation in Python?
While Python's built-in modules provide basic email validation capabilities, using third-party libraries like 'email-validator' or 'validate-email-address' offers more comprehensive and accurate email checking features, including domain and mailbox existence checks.
Conclusion:
Implementing an email checker in Python is essential for ensuring the validity and integrity of email addresses in your applications. By leveraging the power of libraries like 'email-validator' or 'validate-email-address,' you can efficiently validate email addresses, enhance deliverability rates, and maintain a clean email database. Remember to incorporate email validation as an integral part of your development process to ensure reliable communication and successful email campaigns.