In today's digital age, email communication plays a vital role in personal and professional interactions. As a web developer or website owner, ensuring the validity and accuracy of user-submitted email addresses is crucial for maintaining a seamless user experience and effective communication channels. JavaScript provides powerful tools and techniques to implement an email checker, enabling you to validate email addresses on the client-side. In this comprehensive article, we will explore the concept of email validation in JavaScript, discuss various implementation approaches, and guide you through the process of creating an efficient email checker to enhance your web applications.
Why Validate Email Addresses?
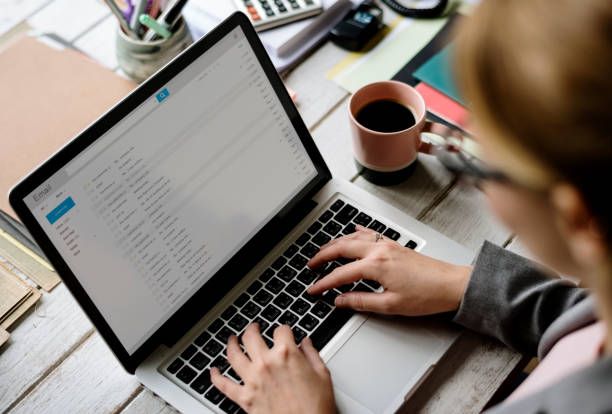
Validating email addresses serves several essential purposes for web developers and website owners. Let's explore the key reasons why implementing an email checker in JavaScript is crucial:
Enhanced User Experience
By validating email addresses on the client-side, you can provide real-time feedback to users during the form submission process. This immediate feedback helps users correct errors and ensures that they provide valid email addresses, minimizing frustration and enhancing the overall user experience.
Data Integrity and Accuracy
Validating email addresses helps maintain data integrity by ensuring that only properly formatted and valid addresses are stored in databases or used for communication purposes. This helps eliminate errors, reduce bounce rates, and improve the accuracy of user data.
Reduced Server Load and Bandwidth Usage
Client-side email validation offloads the task from the server, reducing server load and bandwidth usage. By validating email addresses before they are submitted to the server, you can save valuable resources and improve the efficiency and scalability of your web application.
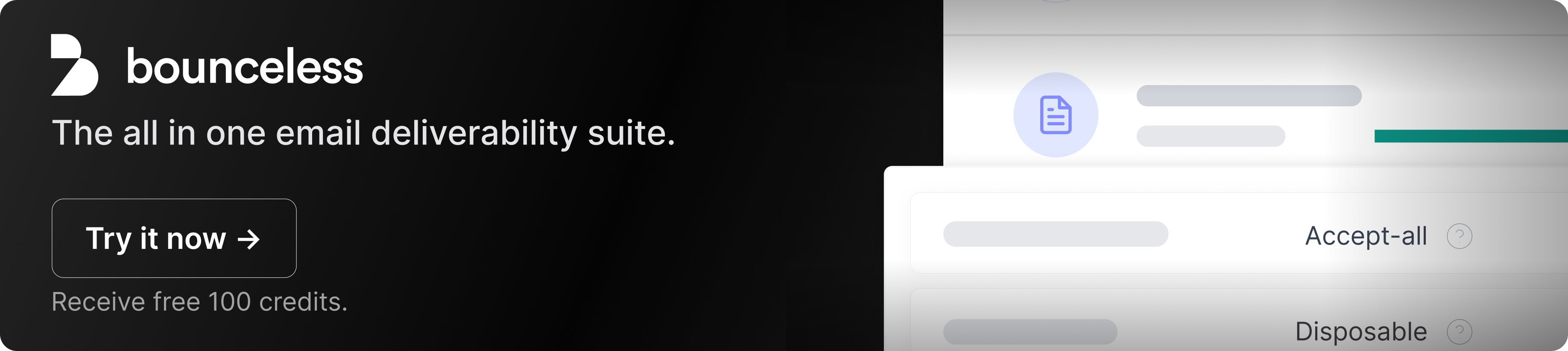
Approaches to Email Validation in JavaScript
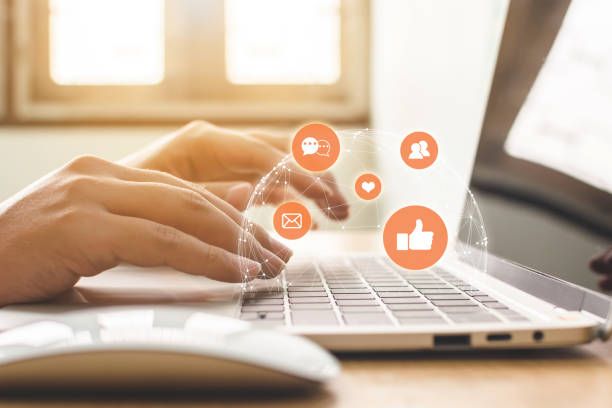
When it comes to implementing an email checker in JavaScript, there are multiple approaches you can consider. Let's explore two commonly used methods:
Regular Expressions (Regex)
Regular expressions offer a powerful and flexible way to validate email addresses in JavaScript. By defining a pattern that matches the structure of a valid email address, you can use regular expressions to test whether an entered email address meets the specified criteria. Various regular expressions patterns exist for email validation, each with its own level of strictness and complexity.
HTML5 Email Input Type
HTML5 introduced the <input>
element with the type="email"
attribute, which provides built-in email validation in modern browsers. This attribute uses a pre-defined email validation pattern to ensure that user input conforms to the structure of a valid email address. However, it's important to note that relying solely on HTML5 email input validation may not be sufficient, as it may not cover all edge cases and may vary in consistency across different browsers.
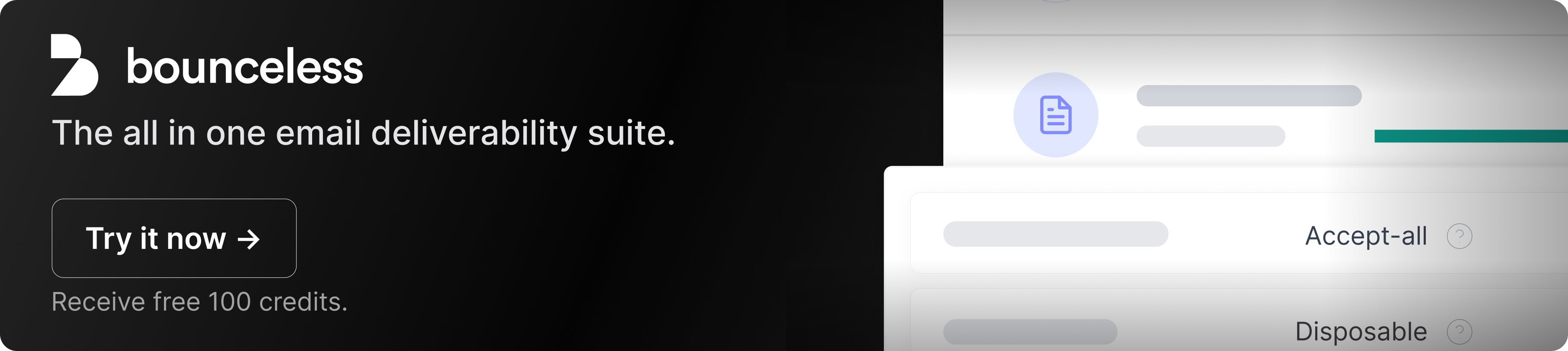
Implementing an Email Checker in JavaScript
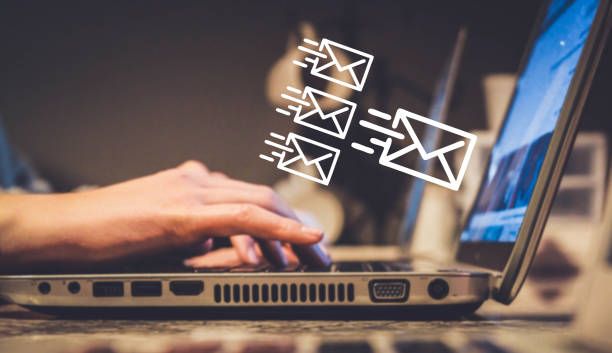
Now, let's walk through the process of implementing an email checker in JavaScript using regular expressions:
Create an HTML form with an email input field and a submit button.
javascriptCopy code<form> <input type="email" id="emailInput" required> <button type="submit">Submit</button></form>
Access the input field and attach an event listener to the form submission.
javascriptCopy codeconst form = document.querySelector('form'); const emailInput = document.getElementById('emailInput'); form.addEventListener('submit', validateEmail);
Define a function to handle the form submission and validate the email address.
javascriptCopy codefunction validateEmail(event) { event.preventDefault(); const email = emailInput.value; // Regular expression pattern for email validation const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; if (emailPattern.test(email)) { // Valid email address // Proceed with form submission or further processing } else { // Invalid email address // Display an error message or provide feedback to the user } }
By following these steps, you can implement an email checker in JavaScript using regular expressions to validate the entered email address.
Frequently Asked Questions
Q: Why is client-side email validation necessary if server-side validation is already in place?
A: Client-side email validation provides immediate feedback to users, reducing the need for server round-trips and enhancing the user experience. It helps catch errors early, ensures faster form submissions, and reduces unnecessary server load.
Q: Are regular expressions the only method for email validation in JavaScript?
A: Regular expressions are a widely used method for email validation due to their flexibility and power. However, other techniques, such as using third-party libraries or API services, can also be employed depending on the specific requirements and complexity of the email validation process.
Q: Can client-side email validation alone guarantee the authenticity of an email address?
A: Client-side email validation ensures that the entered email address adheres to the specified format. However, it does not guarantee the authenticity of the email address itself. Additional server-side validation and verification steps are recommended to ensure the legitimacy of email addresses.
Q: What are the best practices for displaying error messages during email validation?
A: When displaying error messages for email validation, it is important to provide clear and concise instructions to users, indicating the specific validation criteria that were not met. Consider using visually prominent error messages near the email input field to capture the user's attention.
Conclusion
Implementing an email checker in JavaScript allows you to validate email addresses on the client-side, enhancing the user experience, data integrity, and communication channels of your web applications. By following best practices, leveraging regular expressions or HTML5 email input validation, and considering additional server-side validation, you can ensure the accuracy and validity of user-submitted email addresses. With a robust email checker in place, you can streamline your web forms, improve data quality, and provide a seamless experience for users interacting with your web application.