Email address validation is a crucial aspect of web development, ensuring that users enter valid email addresses when submitting forms or interacting with your web applications. JavaScript provides a powerful set of tools and techniques to validate email addresses efficiently and accurately.
In this comprehensive guide, we will explore different methods, libraries, and best practices for email address validation in JavaScript. Whether you are a beginner or an experienced developer, this article will equip you with the knowledge and resources to master email address validation in JavaScript.
Understanding Email Address Validation

Validating email addresses involves verifying if an email input conforms to the standard email format and ensuring that the domain exists and is active. While it might seem simple at first, validating email addresses can be a complex task due to the various rules and potential edge cases involved. However, JavaScript provides numerous techniques and libraries to simplify this process and handle different scenarios effectively.
Basic Email Address Validation with Regular Expressions
Regular expressions (regex) are a powerful tool in JavaScript for pattern matching and string manipulation. They can be used to validate email addresses by checking if the input matches the standard email format. Let's take a look at an example of a basic email address validation regex pattern:javascriptCopy codeconst emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
In this regex pattern, we ensure that the email address has at least one character before the "@" symbol, followed by the "@" symbol itself, a domain name (at least one character before and after the dot), and the top-level domain (TLD). While this basic pattern covers most cases, it doesn't handle more advanced scenarios such as internationalized email addresses or specific domain restrictions.
Advanced Email Address Validation with Libraries
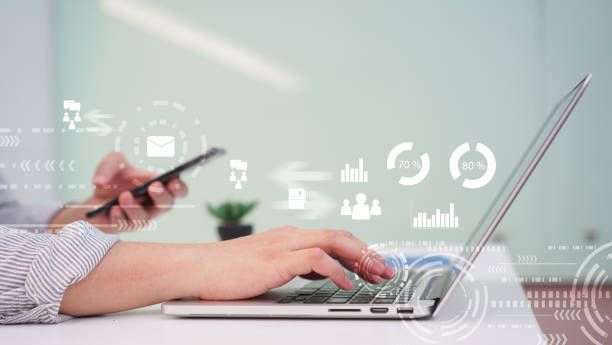
To handle more complex email address validation scenarios, various JavaScript libraries are available. One popular library is Mailcheck. Mailcheck suggests corrections for common typos in email addresses, such as misspelled domain names or TLDs. It provides a better user experience by reducing the likelihood of users submitting incorrect email addresses. You can easily integrate Mailcheck into your web application using the provided JavaScript API.javascriptCopy codeMailcheck.run({ email: 'user@gnail.com', suggested: function(element, suggestion) { // Handle the suggested correction }, empty: function(element) { // Handle the case when no suggestion is available } });
By leveraging the power of libraries like Mailcheck, you can not only validate email addresses but also enhance the user experience by suggesting corrections in case of common typos.
Best Practices for Email Address Validation
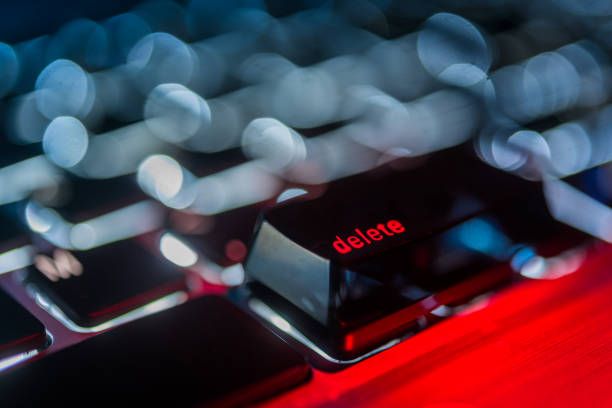
While using regular expressions or libraries can significantly improve the accuracy of email address validation, it is important to follow best practices to ensure a robust and reliable validation process. Here are some essential best practices to consider:
- Server-Side Validation: JavaScript-based email address validation should always be complemented with server-side validation. Client-side validation can be bypassed, so it's crucial to have a server-side mechanism to validate email addresses before processing them.
- HTML5 Email Input Type: Utilize the HTML5 email input type for form fields that accept email addresses. This built-in browser validation automatically validates the email format and provides a native error message if the input is invalid.
- Regular Expression Optimization: Regular expressions can become complex and resource-intensive, affecting performance. Optimize your regex patterns to strike a balance between accuracy and efficiency. Test and profile your validation code to ensure optimal performance.
- Internationalized Email Addresses: Consider supporting internationalized email addresses, which allow non-ASCII characters in the local part of the email address. Use libraries or built-in JavaScript functions like
encodeURIComponent
to handle encoding and decoding of internationalized characters.
Frequently Asked Questions
Q1. Can I rely solely on client-side email address validation?
A1. While client-side validation improves user experience, it should always be complemented with server-side validation. Client-side validation can be bypassed, and relying solely on it can expose your application to potential security vulnerabilities.
Q2. What is the most accurate regex pattern for validating email addresses?
A2. Email address validation with regex can be challenging due to the complexity of email address rules. While there is no one-size-fits-all regex pattern, using a combination of basic patterns, like the one mentioned earlier, along with additional checks for specific requirements can provide a good balance between accuracy and performance.
Q3. Are there any built-in JavaScript functions for email address validation?
A3. JavaScript itself does not provide built-in functions specifically for email address validation. However, HTML5 introduced the email input type, which performs basic validation automatically in supporting browsers.
Q4. How can I handle disposable email addresses during validation?
A4. To handle disposable email addresses, you can use third-party services or databases that maintain a list of disposable email domains. By checking the domain part of the email address against this list, you can identify and reject disposable email addresses.
Conclusion
Mastering email address validation in JavaScript is crucial for developing robust and user-friendly web applications. By utilizing regular expressions, libraries like Mailcheck, and following best practices, you can ensure accurate and reliable email address validation. Remember to complement client-side validation with server-side validation for enhanced security. Stay up-to-date with the latest email address validation techniques and continuously refine your validation code to improve the user experience. With the knowledge gained from this comprehensive guide, you are well-equipped to handle email address validation challenges effectively in your JavaScript projects.