In the digital age, where email is the backbone of communication, validating email addresses is paramount for web developers and designers. JavaScript, being a versatile programming language, plays a vital role in email validation on the client-side. In this comprehensive guide, we will explore the ins and outs of email validation with JavaScript, equipping you with the knowledge to ensure error-free input and enhance user experience. As a seasoned expert in the field, I'll walk you through the best practices, essential techniques, and answer common questions to empower you to master email validation like a pro.
Understanding the Importance of Email Validation:
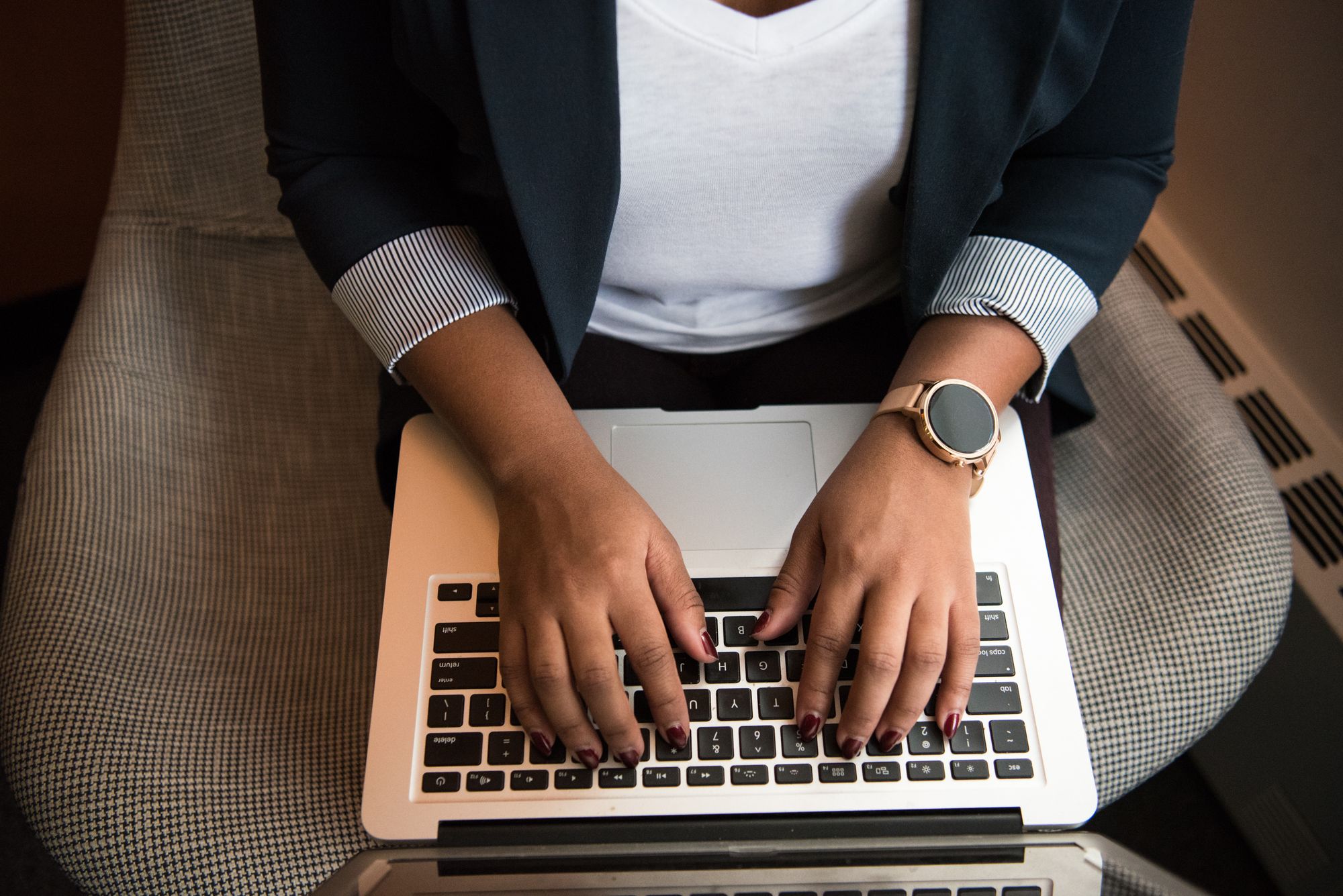
Before delving into the technicalities of email validation with JavaScript, let's emphasize why it is crucial. When users submit forms on websites or applications, ensuring the accuracy of their provided email addresses is fundamental. Email validation helps to:
Improve Data Quality: Accurate email addresses lead to better data quality, allowing businesses to reach their customers more effectively.
Enhance User Experience: Validating email addresses in real-time prevents users from encountering form submission errors later, leading to a smoother and more satisfying experience.
Mitigate Spam and Bots: By verifying email addresses, you can reduce the chances of spam submissions and malicious bot activities.
Ensure Successful Communication: With validated email addresses, businesses can maintain clear and reliable communication channels with their users.
Email Validation Methods:
Several methods for email validation exist, but not all are equally effective. One popular approach involves using Regular Expressions (RegEx) to match email patterns. RegEx patterns help identify valid email addresses based on specific rules, but they might not catch all edge cases.
Another technique involves utilizing third-party email validation APIs, which can be more accurate but may come with additional costs or depend on external services. For client-side email validation, JavaScript proves to be a powerful and efficient tool, offering real-time feedback to users during form submission.
Performing Email Validation with JavaScript:
To begin validating email addresses with JavaScript, developers can use various built-in methods and functions. One of the most common methods is using the RegExp
object to create a RegEx pattern for email validation. The following is a sample JavaScript function for email validation:
function isValidEmail(email) {
const emailPattern = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
return emailPattern.test(email);
}
In this function, we use the test()
method to check if the email
parameter matches the email pattern defined by the emailPattern
variable.
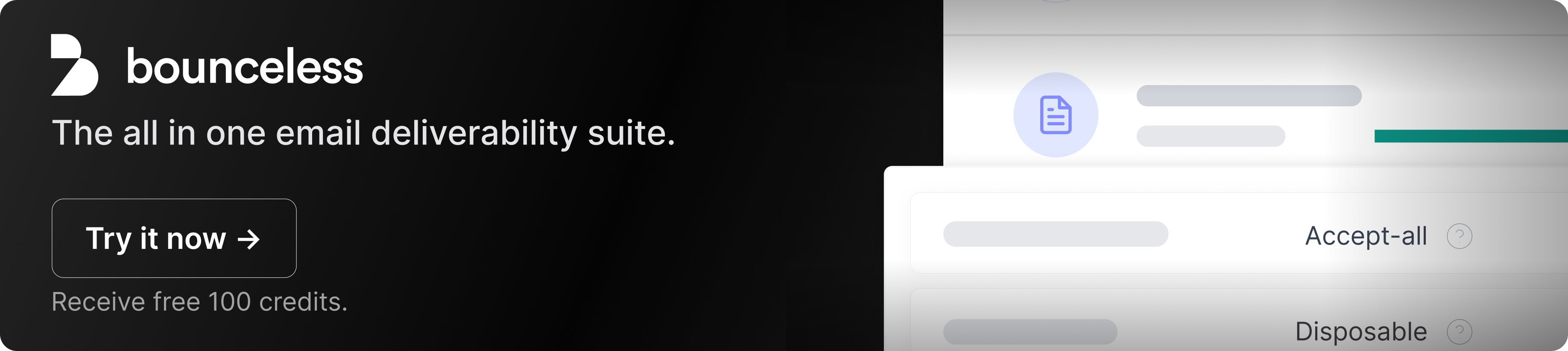
Handling Common Pitfalls:
While the above function provides a basic level of email validation, there are still some common pitfalls to address. For instance, it does not detect email addresses with multiple consecutive dots or other unusual formats that are technically valid but rarely used. To tackle such issues, you can enhance the validation logic by adding additional checks and customizing the RegEx pattern according to your specific use case.
Moreover, it's important to consider the user experience when providing error messages for invalid email inputs. Clear and concise messages will guide users in correcting their mistakes, reducing frustration and form abandonment.
Best Practices for Robust Email Validation:
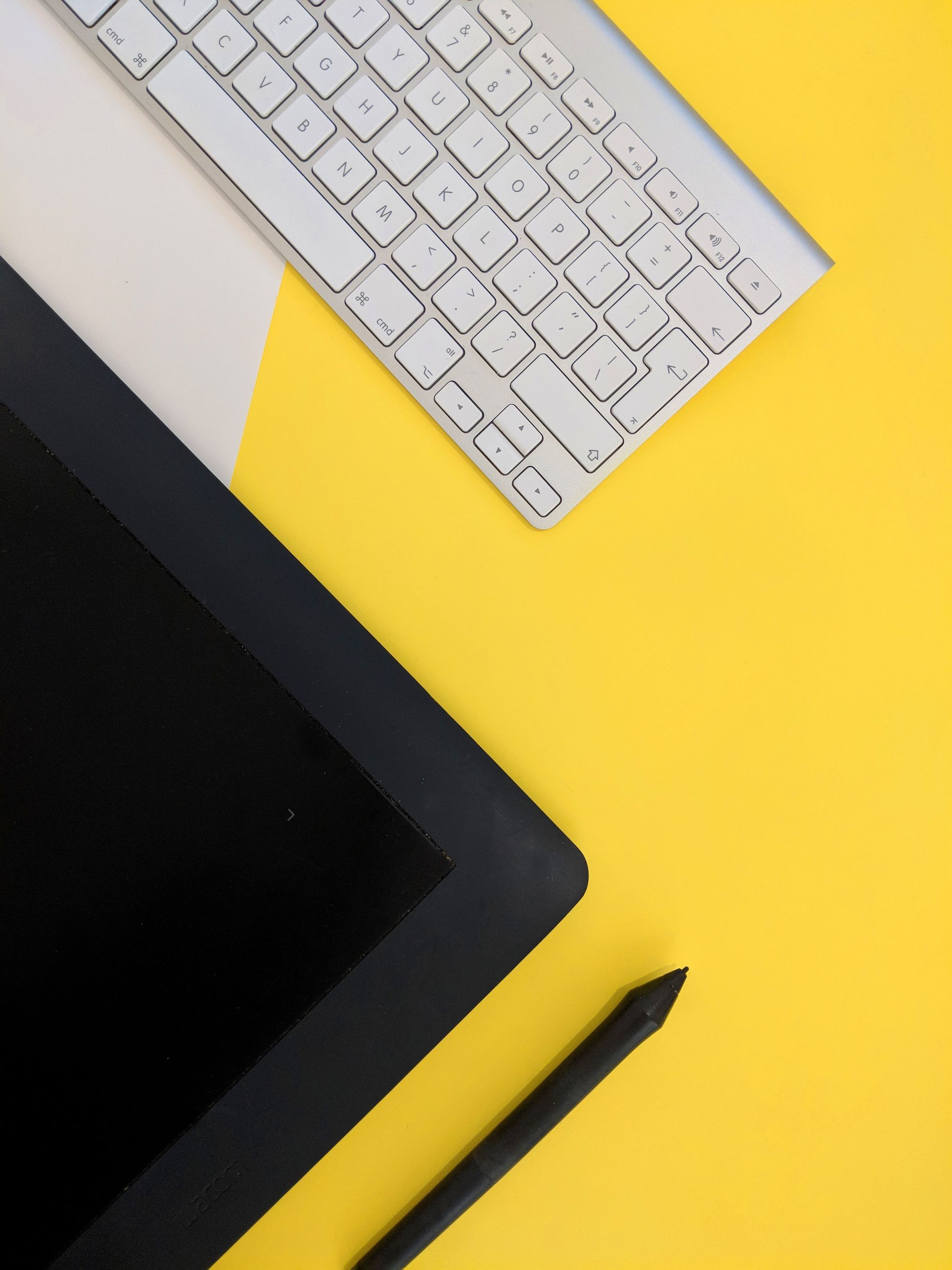
To achieve the best results, follow these best practices for robust email validation with JavaScript:
Use Built-in HTML5 Validation: HTML5 provides built-in email validation attributes like type="email"
and pattern
to ensure a basic level of validation before JavaScript is even executed.
Implement Real-Time Feedback: Offer users real-time feedback while they fill out the email field, guiding them through the validation process.
Avoid Overvalidation: Strive for a balance between strict validation and avoiding false negatives. Ensure that the majority of valid email addresses pass the validation while preventing obvious errors.
Regular Expression Optimization: Fine-tune your RegEx pattern to optimize performance and accuracy, taking into account any specific requirements.
Consider Server-Side Validation: While client-side validation is beneficial for instant feedback, complement it with server-side validation to maintain data integrity and security.
Update Validation Logic Regularly: Keep an eye on email address trends and standards to update your validation logic as needed.
Frequently Asked Questions (FAQs):
Can JavaScript alone handle all email validation tasks?
While JavaScript is powerful for client-side validation, it is crucial to combine it with server-side validation for comprehensive security and data integrity.
What are the limitations of using RegEx for email validation?
RegEx patterns may not catch all edge cases, and some technically valid email addresses might still be considered invalid due to strict patterns.
Is it better to use third-party email validation APIs?
Third-party APIs can offer more accurate validation, but they come with potential costs and reliance on external services. Evaluate your needs and budget before making a decision.
How can I provide meaningful error messages for email validation?
Craft clear and concise error messages that guide users in correcting their mistakes. Avoid generic error messages and provide specific instructions.
Are there any built-in HTML attributes for email validation?
Yes, HTML5 offers type="email"
and pattern
attributes that provide basic email validation without using JavaScript.
In conclusion, mastering email validation with JavaScript is essential for maintaining data quality, enhancing user experience, and preventing spam and bot activities. By implementing best practices, understanding common pitfalls, and continuously updating your validation logic, you can ensure error-free user input and foster better communication with your audience. Remember to strike a balance between strict validation and user convenience, and consider both client-side and server-side validation for a robust approach. With the knowledge gained from this comprehensive guide, you're well-equipped to excel in the world of email validation with JavaScript. Happy coding!